Question
I have this lab in java and I have gone through the tutorials and I just am not getting the understanding... Any help and feedback
I have this lab in java and I have gone through the tutorials and I just am not getting the understanding... Any help and feedback is greatly appreciated: I hope you can read it I had to redo for the question to post and I will put the rest of the java files in another question.
This is the short version of the lab
CSC-251 Lambda and Anonymous Classes Mutual Fund Screen
When people allocate their investment portfolios (think 401k plans), they often want to find mutual funds or ETFs with certain characteristics. For example, some people might want to limit their investments to mutual funds that invest only in big, safe companies. Others might want to specifically exclude such companies.
The attached starter code contains the beginnings of such an application. You are to complete it.
The FundScreen Program
A single mutual funds description is held in a MutualFund object. A collection of MutualFund objects is held in a Portfolio, which subclasses ArrayList
The main method is in FundScreen, which is the class you are to modify.
Your Mission
FundScreen has two methods that you are to modify: keep and exclude. Your mission is to complete these methods using only calls to ArrayLists removeIf method. Information on removeIf is in the ArrayList description. Google oracle java 8 api ArrayList to find Oracles official documentation for ArrayList. Locations for calls to removeIf are noted with TODO flags in the code.
keep
The keep method allows the user to specify which mutual funds should be kept in the portfolio. All other funds will be removed. For example, in the following interaction,
Make a Selection:
[0] List
[1] Exclude
[2] Retain
[3] Reset Portfolio
[4] Exit
2
What field and values identify funds you wish to keep?
Make a Selection:
[0] Ticker
[1] Average Holding Size
[2] Minimum Investment
[3] Value Measure
[4] Domicile
0
Which ticker? VIOV
The user specifies that fund VIOV is to be kept in the portfolio, and all others are to be discarded.
ALL CALLS TO removeIf IN THE keep METHOD ARE TO USE ANONYMOUS CLASSES AS THE Predicate.
Since we havent covered enum, the Domicile processing of the keep method is completed for you.
exclude
The exclude method is the opposite of keep. It allows the user to specify which funds are to be removed from the portfolio. The following interaction removes fund VT from the portfolio, and leaves all the others:
Make a Selection:
[0] List
[1] Exclude
[2] Retain
[3] Reset Portfolio
[4] Exit
1
What field and values identify funds you wish to remove?
Make a Selection:
[0] Ticker
[1] Average Holding Size
[2] Minimum Investment
[3] Value Measure
[4] Domicile
0
Which ticker? vt
ALL CALLS TO removeIf IN THE exclude METHOD ARE TO USE LAMBDA EXPRESSIONS AS THE Predicate.
Objectives
At the end of this exercise, the student will be able to
Write anonymous classes to implement a Predicate interface
Write lambda expressions to implement a Predicate interface
Use the removeIf method of ArrayList to identify elements to be removed from the collection
Grading Elements
The keep method identifies elements to be kept in the list based on ticker, average holding size, minimum investment, or value measure. All other elements are discarded
The exclude method allows the user to specify elements that are to be removed from the list based on ticker, average holding size, minimum investment, or value measure. All other elements are kept.
Only ArrayLists removeIf method is used to modify the list
All calls to removeIf in the keep method use an anonymous class to implement the Predicate interface
All calls to removeIf in the exclude method use a lambda expression to implement the Predicate interface
This is the fundscreen.java
import java.util.Scanner; import java.util.function.Predicate;
public class FundScreen {
public static final String LIST = "List"; public static final String EXCLUDE = "Exclude"; public static final String KEEP = "Retain"; public static final String RESET_PORTFOLIO = "Reset Portfolio"; public static final String EXIT = "Exit";
public static final String TICKER = "Ticker"; public static final String AVG_HOLDING_SIZE = "Average Holding Size"; public static final String MIN_INVESTMENT = "Minimum Investment"; public static final String VALUE_MEASURE = "Value Measure"; public static final String DOMICILE = "Domicile";
public static final String[] BIG_MENU = { LIST, EXCLUDE, KEEP, RESET_PORTFOLIO, EXIT, };
public static final String[] FUND_FIELDS = { TICKER, AVG_HOLDING_SIZE, MIN_INVESTMENT, VALUE_MEASURE, DOMICILE, };
public static void main(String[] args) { Scanner kybd = new Scanner(System.in); Portfolio portfolio = new Portfolio(); String userChoice = ""; while (!EXIT.equals(userChoice)) { userChoice = Utils.userChoose(kybd, BIG_MENU); if (LIST.equals(userChoice)) { System.out.println("Current Portfolio"); Utils.userDisplay(kybd, portfolio); } else if (EXCLUDE.equals(userChoice)) { exclude(kybd, portfolio); } else if (KEEP.equals(userChoice)) { keep(kybd, portfolio); } else if (RESET_PORTFOLIO.equals(userChoice)) { portfolio.refresh(); } } }
private static void keep(Scanner kybd, Portfolio portfolio) { System.out.println("What field and values identify funds you wish to keep?"); String keeping = Utils.userChoose(kybd, FUND_FIELDS); if (TICKER.equals(keeping)) { System.out.print("Which ticker? "); String ticker = kybd.next().toUpperCase(); // TODO call portfolio.removeIf(...); } else if (AVG_HOLDING_SIZE.equals(keeping)) { System.out.print("Lower limit for holding size: "); double floor = kybd.nextDouble(); System.out.print("Upper limit for holding size: "); double ceiling = kybd.nextDouble(); // TODO call portfolio.removeIf(...);
} else if (MIN_INVESTMENT.equals(keeping)) { System.out.print("Lower limit for minimum investment: "); double floor = kybd.nextDouble(); System.out.print("Upper limit for minimum investment: "); double ceiling = kybd.nextDouble(); // TODO call portfolio.removeIf(...);
} else if (VALUE_MEASURE.equals(keeping)) { System.out.print("Lower limit for value: "); double floor = kybd.nextDouble(); System.out.print("Upper limit for value: "); double ceiling = kybd.nextDouble(); // TODO call portfolio.removeIf(...);
} else if (DOMICILE.equals(keeping)) { keeping = Utils.userChoose(kybd, MutualFund.MARKET.values()); MutualFund.MARKET mkt = MutualFund.MARKET.valueOf(keeping); // this one is supplied for you portfolio.removeIf(new Predicate
@Override public boolean test(MutualFund t) { System.out.println(t); return !(mkt == t.getDomicile()); } });
} }
private static void exclude(Scanner kybd, Portfolio portfolio) { System.out.println("What field and values identify funds you wish to remove?"); String removing = Utils.userChoose(kybd, FUND_FIELDS); if (TICKER.equals(removing)) { System.out.print("Which ticker? "); String ticker = kybd.next().toUpperCase(); // TODO call portfolio.removeIf(...);
} else if (AVG_HOLDING_SIZE.equals(removing)) { System.out.print("Lower limit for holding size: "); double floor = kybd.nextDouble(); System.out.print("Upper limit for holding size: "); double ceiling = kybd.nextDouble(); // TODO call portfolio.removeIf(...);
} else if (MIN_INVESTMENT.equals(removing)) { System.out.print("Lower limit for minimum investment: "); double floor = kybd.nextDouble(); System.out.print("Upper limit for minimum investment: "); double ceiling = kybd.nextDouble(); // TODO call portfolio.removeIf(...); } else if (VALUE_MEASURE.equals(removing)) { System.out.print("Lower limit for value: "); double floor = kybd.nextDouble(); System.out.print("Upper limit for value: "); double ceiling = kybd.nextDouble(); // TODO call portfolio.removeIf(...);
} else if (DOMICILE.equals(removing)) { removing = Utils.userChoose(kybd, MutualFund.MARKET.values()); MutualFund.MARKET mkt = MutualFund.MARKET.valueOf(removing); // TODO call portfolio.removeIf(...); } }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
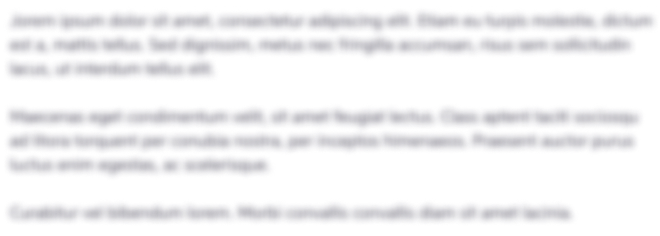
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started