Question
I have this program I'm working on, I have most of it done. My main issue is I cant attach the bar chart to my
I have this program I'm working on, I have most of it done. My main issue is I cant attach the bar chart to my GUI layout. If anyone could help I would greatly appreciate it.
Instructions
Write a GUI application with three labeled text fields, one each for the initial amount of a savings account greater than 0, the annual interest rate greater than 0, and the number of years greater than 0. Add a button Calculate and a read-only text area to display the balance of the savings account after the end of each year. Include another area with a bar chart that shows the balance after the end of each year. All data entered by the user should be validated and there should be a quit or cancel button to end the program. Pressing the calculate button again will recalculate everything again. It is the programmers responsibility to ensure that no matter what the user enters, the program does not crash. Call the program SavingsCalculatorViewer.java
My code
SavingsChart.java
package savingscalculatorviewer;
/** * * @author jmart790 */ import java.awt.Color; import java.awt.Dimension; import java.awt.Font; import java.awt.FontMetrics; import java.awt.Graphics;
import javax.swing.JPanel;
public class SavingsChart extends JPanel { private double[] savingsAmount; private String[] year; private String title;
public SavingsChart(double[] savings, String[] savingsYear, String titleLabel) { year = savingsYear; savingsAmount = savings; title = titleLabel; }
public void paintComponent(Graphics g) { super.paintComponent(g); if (savingsAmount == null || savingsAmount.length == 0) return; double minValue = 0; double maxValue = 0; for (int i = 0; i < savingsAmount.length; i++) { if (minValue > savingsAmount[i]) minValue = savingsAmount[i]; if (maxValue < savingsAmount[i]) maxValue = savingsAmount[i]; }
Dimension graphSize = getSize(); int graphWidth = graphSize.width; int graphHeight = graphSize.height; int barWidth = graphWidth / savingsAmount.length;
Font titleFont = new Font("SansSerif", Font.BOLD, 16); FontMetrics titleFontMetrics = g.getFontMetrics(titleFont); Font labelFont = new Font("SansSerif", Font.PLAIN, 10); FontMetrics labelFontMetrics = g.getFontMetrics(labelFont);
int titleWidth = titleFontMetrics.stringWidth(title); int y = titleFontMetrics.getAscent(); int x = (graphWidth - titleWidth) / 2; g.setFont(titleFont); g.drawString(title, x, y);
int top = titleFontMetrics.getHeight(); int bottom = labelFontMetrics.getHeight(); if (maxValue == minValue) return; double scale = (graphHeight - top - bottom) / (maxValue - minValue); y = graphHeight - labelFontMetrics.getDescent(); g.setFont(labelFont);
for (int i = 0; i < savingsAmount.length; i++) { int valueX = i * barWidth + 1; int valueY = top; int height = (int) (savingsAmount[i] * scale); if (savingsAmount[i] >= 0) { valueY += (int) ((maxValue - savingsAmount[i]) * scale); } else { valueY += (int) (maxValue * scale); height = -height; }
g.setColor(Color.GREEN); g.fillRect(valueX, valueY, barWidth - 2, height); g.setColor(Color.black); g.drawRect(valueX, valueY, barWidth - 2, height); int labelWidth = labelFontMetrics.stringWidth(year[i]); x = i * barWidth + (barWidth - labelWidth) / 2; g.drawString(year[i], x, y); } } }
SavingsCalculatorViewer.java
package savingscalculatorviewer;
import java.awt.GridBagConstraints; import java.awt.GridBagLayout; import java.awt.Insets; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent; import java.awt.event.WindowListener; import java.text.NumberFormat; import javax.swing.JButton; import javax.swing.JFormattedTextField; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JScrollPane; import javax.swing.JTextArea;
public class SavingsCalculatorViewer extends JFrame implements ActionListener { private JTextArea outputArea; // Displays yearly savings private JButton calcButton; // Triggers savings calculation private JButton quitButton; // Triggers exit private JFormattedTextField initSavingsField; // Holds savings amount private JFormattedTextField interestRateField; // Holds interest amount private JFormattedTextField yearsField; // Holds num years
/* Constructor creates GUI components and adds GUI components using a GridBagLayout. */ SavingsCalculatorViewer() { GridBagConstraints savingsLayout = null; // Used to specify GUI component layout JScrollPane scrollPane = null; // Container that adds a scroll bar JLabel initSavingsLabel = new JLabel("Initial savings:"); // Label for savings JLabel interestRateLabel = new JLabel("Interest rate:"); // Label for interest JLabel yearsLabel = new JLabel("Years:"); // Label for num years JLabel outputLabel = new JLabel("Yearly savings:"); // Label for yearly savings
// Format for the savings input NumberFormat currencyFormat = null; // Format for the interest rate input NumberFormat percentFormat = null; // Format for the years input NumberFormat yearsFormat = null;
// Set frame's title setTitle("Savings Calculator");
// Create output area and add it to scroll pane outputArea = new JTextArea(10, 15); scrollPane = new JScrollPane(outputArea); outputArea.setEditable(false);
calcButton = new JButton("Calculate"); calcButton.addActionListener(this); quitButton = new JButton("Exit"); quitButton.addActionListener(e -> this.dispose());
// Create savings field and specify the currency format currencyFormat = NumberFormat.getNumberInstance(); initSavingsField = new JFormattedTextField(currencyFormat); initSavingsField.setEditable(true); initSavingsField.setColumns(15); // Initial width of 10 units initSavingsField.setValue(0);
// Create rate field and specify the percent format percentFormat = NumberFormat.getNumberInstance(); percentFormat.setMinimumFractionDigits(1); interestRateField = new JFormattedTextField(percentFormat); interestRateField.setEditable(true); interestRateField.setValue(0);
// Create years field and specify the default number (for doubles) format yearsFormat = NumberFormat.getIntegerInstance(); yearsField = new JFormattedTextField(yearsFormat); yearsField.setEditable(true); yearsField.setValue(0);
// Use a GridBagLayout setLayout(new GridBagLayout());
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(10, 10, 5, 1); savingsLayout.anchor = GridBagConstraints.LINE_END; savingsLayout.gridx = 0; savingsLayout.gridy = 0; add(initSavingsLabel, savingsLayout);
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(10, 1, 5, 10); savingsLayout.fill = GridBagConstraints.HORIZONTAL; savingsLayout.gridx = 1; savingsLayout.gridy = 0; add(initSavingsField, savingsLayout);
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(5, 10, 5, 1); savingsLayout.anchor = GridBagConstraints.LINE_END; savingsLayout.gridx = 0; savingsLayout.gridy = 1; add(interestRateLabel, savingsLayout);
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(5, 1, 5, 10); savingsLayout.fill = GridBagConstraints.HORIZONTAL; savingsLayout.gridx = 1; savingsLayout.gridy = 1; add(interestRateField, savingsLayout);
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(5, 10, 10, 1); savingsLayout.anchor = GridBagConstraints.LINE_END; savingsLayout.gridx = 0; savingsLayout.gridy = 2; add(yearsLabel, savingsLayout);
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(5, 1, 10, 10); savingsLayout.fill = GridBagConstraints.HORIZONTAL; savingsLayout.gridx = 1; savingsLayout.gridy = 2; add(yearsField, savingsLayout);
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(0, 5, 0, 10); savingsLayout.fill = GridBagConstraints.BOTH; savingsLayout.gridx = 2; savingsLayout.gridy = 1; add(calcButton, savingsLayout); savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(0, 5, 0, 10); savingsLayout.fill = GridBagConstraints.BOTH; savingsLayout.gridx = 2; savingsLayout.gridy = 2; add(quitButton, savingsLayout);
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(10, 10, 1, 10); savingsLayout.fill = GridBagConstraints.HORIZONTAL; savingsLayout.gridx = 0; savingsLayout.gridy = 3; add(outputLabel, savingsLayout);
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(1, 10, 10, 10); savingsLayout.fill = GridBagConstraints.HORIZONTAL; savingsLayout.gridx = 0; savingsLayout.gridy = 4; savingsLayout.gridwidth = 3; // 3 cells wide add(scrollPane, savingsLayout);
double savingsDollars = ((Number) initSavingsField.getValue()).doubleValue(); double interestRate = ((Number) interestRateField.getValue()).doubleValue(); int numYears = ((Number) yearsField.getValue()).intValue(); JFrame f = new JFrame(); f.setSize(400, 300); double[] values = new double[numYears]; String[] names = new String[numYears]; for (int i = 0; i < numYears; i++) { values[i] = savingsDollars + (savingsDollars * interestRate); int yearValue = i+1; names[i] = "Year " + yearValue; }
SavingsChart newChart = new SavingsChart(values, names, "Savings Chart");
savingsLayout = new GridBagConstraints(); savingsLayout.insets = new Insets(5, 5, 5, 5); savingsLayout.fill = GridBagConstraints.EAST; savingsLayout.gridx = 10; savingsLayout.gridy = 40; add(newChart, savingsLayout);
} @Override public void actionPerformed(ActionEvent event) { int i = 0; // Loop index double savingsDollars = 0.0; // Yearly savings double interestRate = 0.0; // Annual interest rate int numYears = 0; // Num years to calc savings
// Get values from fields savingsDollars = ((Number) initSavingsField.getValue()).doubleValue(); interestRate = ((Number) interestRateField.getValue()).doubleValue(); numYears = ((Number) yearsField.getValue()).intValue();
// Clear the text area outputArea.setText("");
// Calculate savings iteratively in a while loop i = 1; while (i <= numYears) { outputArea.append(String.format("Savings in year " + i + ": $%,.2f ", savingsDollars)); savingsDollars = savingsDollars + (savingsDollars * interestRate);
i = i + 1; }
}
/* Creates a SavingsInterestCalcFrame and makes it visible */ public static void main(String[] args) { // Creates SavingsInterestCalcFrame and its components SavingsCalculatorViewer myFrame = new SavingsCalculatorViewer();
myFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); myFrame.pack(); myFrame.setVisible(true);
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
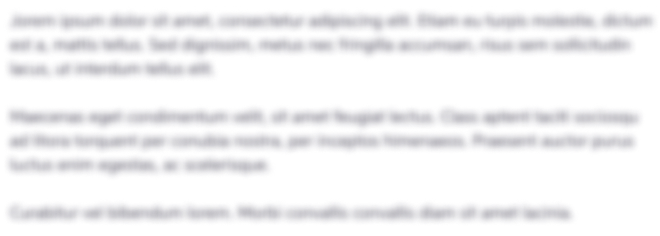
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started