Question
I HAVE TO WRITE A JAVA PROGRAM USING ECLIPSE USING JavaFX. I AM HAVING TROUBLE TAKING MY CODE FROM THE BEFORE ASSIGNMENT AND MAKING IT
I HAVE TO WRITE A JAVA PROGRAM USING ECLIPSE USING JavaFX. I AM HAVING TROUBLE TAKING MY CODE FROM THE BEFORE ASSIGNMENT AND MAKING IT LOOK LIKE THE OUTPUT THAT THEY WANT. I Have To Use an image of a field with a brown fence around it. Plots can be solid colored rectangles of different colors. Set the dimensions to 200, plot dimensions to 20, and number of plots to 6. Under the field display your name and a list of each plot color in a rectangle of black border and correct color and upper left coordinate.
I HAVE LINKED MY CODE THAT NEEDS TO BE USED FOR THIS ASSIGNMENT BELOW THE FIRST IMAGE AND LABELED THE CLASSES IN BOLD.
GeometricObject.java:
public abstract class GeometricObject {
private String color = "white"; // shape color
private boolean filled; // fill status
protected GeometricObject() { // POST: default shape is unfilled blue
this.color = "blue";
this.filled = false; }
// POST: shape set by user
protected GeometricObject(String color, boolean filled) {
this.color = color;
this.filled = filled;
}
// accessors and modifiers
public String getColor() { return color; }
public void setColor(String color) { this.color = color; }
public boolean isFilled() { return filled; }
public void setFilled(boolean filled) { this.filled = filled;
}
public String toString() { // POST: string representation of shape
return "color: " + color + " filled: " + filled;
}
public abstract double getArea(); // POST: return area of geometric object
public abstract double getPerimeter(); // POST: return perimeter of geometric object
}
Point.Java:
public class Point {
private double x ; // x coordinate
private double y; // y coordinate
public Point ( ) // POST: point (0,0)
{ x = y = 0; }
public Point (double x, double y) // POST: point (x,y)
{ this.x = x;
this.y = y; }
// accessors and modifiers
public double getX() {
return x; }
public void setX(double x) {
this.x = x; }
public double getY() {
return y; }
public void setY(double y) {
this.y = y; }
public String toString() // POST: return (x,y)
{ return " (" + x + "," + y + ") "; }
}
Rectangle.Java:
public class Rectangle extends GeometricObject{
private double width;
private double height;
public Rectangle() {
super();
width = 2.0;
height = 1.0;
}
public Rectangle(double width, double height, String color, boolean filled) {
super (color,filled);
this.width = width;
this.height = height;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
@Override
public double getArea() {
return width * height;
}
@Override
public double getPerimeter() {
return ((2 * width) + (2 * height));
}
@Override
public String toString ( ) {
return super.toString() + " height: " + height + " width: " + width + " area: " +
String.format("%-6.2f",getArea()) + " perimeter: " + String.format("%-10.2f",getPerimeter());
}
}
Rectangle2D.Java:
public class Rectangle2D extends Rectangle {
private Point upperLeft;
public Rectangle2D()
{
super();
upperLeft = new Point(0,0);
}
public Rectangle2D(double x, double y,double width, double height)
{
super(width, height, "white", false);
upperLeft = new Point(x, y);
}
public Rectangle2D(double x, double y,double width, double height, String color, boolean filled)
{
super(width, height, color, filled);
upperLeft = new Point(x, y);
}
public Point getUpperLeft()
{
return upperLeft;
}
public String toString()
{
return super.toString() +" upper left: " + upperLeft;
}
public boolean contains(Point other)
{
if(upperLeft.getX()
{
if(upperLeft.getY()
return true;
}
return false; //for all other cases
}
public Boolean contains (Rectangle2D other)
// POST: return true if object contains other rectangle
{
//compute the lowerRight corner of the other rectangle
Point lowerRight = new Point(other.upperLeft.getX() + other.getWidth() , other.upperLeft.getY() + other.getHeight());
//only if this rectangle contains both the upper left and lower right corners of the other rectangle,
//the other rectangle is fully contained in this rectangle
if(contains(other.upperLeft) && contains(lowerRight))
return true;
else
return false;
}
public Boolean overlaps (Rectangle2D other)
// POST: return true if other overlaps object
{
//calculate the 3 other corners of the other rectangle
Point lowerRight = new Point(other.upperLeft.getX() + other.getWidth() , other.upperLeft.getY() + other.getHeight());
Point upperRight = new Point(other.upperLeft.getX() + other.getWidth() , other.upperLeft.getY() );
Point lowerLeft = new Point(other.upperLeft.getX() , other.upperLeft.getY() + other.getHeight());
//there is an overlap if any of the 4 corners of the other rectangle are contained in this rectangle
if(contains(other.upperLeft) || contains(lowerRight) || contains(upperRight) || contains(lowerLeft))
return true;
else
return false;
}
}
Tester.Java:
import java.util.Scanner;
public class Tester {
public static void main(String[] args) {
Scanner scan = new Scanner (System.in);
System.out.print("Enter (x,y) for start rectangle: ");
double x = scan.nextDouble();
double y = scan.nextDouble();
System.out.print("Enter width,height for start rectangle: ");
double w = scan.nextDouble();
double h = scan.nextDouble();
Rectangle2D rec = new Rectangle2D (x,y,w,h);
String code;
System.out.print("Contains or overlap (C/O): ");
code = scan.next();
if (code.equals("C"))
{ System.out.print("Compare to point or rectangle: (P/R): ");
code = scan.next();
if (code.equals("P"))
{ Point p = new Point();
System.out.print("Enter (x,y) for point: ");
p.setX(scan.nextDouble());
p.setY(scan.nextDouble());
if (rec.contains(p))System.out.println("rec contains point");
else System.out.println("rec does not contain point");
}
else
{ System.out.print("Enter (x,y) for second rectangle: ");
x = scan.nextDouble();
y = scan.nextDouble();
System.out.print("Enter width,height for second rectangle: ");
w = scan.nextDouble();
h = scan.nextDouble();
Rectangle2D rec2 = new Rectangle2D (x,y,w,h);
if (rec.contains(rec2))System.out.println("rec contains second rec" + "");
else System.out.println("rec does not contain second rec");
}
}
else
{ System.out.print("Enter (x,y) for second rectangle: ");
x = scan.nextDouble();
y = scan.nextDouble();
System.out.print("Enter width,height for second rectangle: ");
w = scan.nextDouble();
h = scan.nextDouble();
Rectangle2D rec2 = new Rectangle2D (x,y,w,h);
if (rec.overlaps(rec2))System.out.println("second rec overlaps rec" + "");
else System.out.println("second rec does not overlap rec");
}
scan.close();
}
}
Part II. (80 pts.) Write a program to solve the following problem. Use the code written for Assignment #1 and display the field of plots using JavaFX. Use an image of a field with a brown fence around it. Plots can be solid colored rectangles of different colors. Set the dimensions to 200, plot dimensions to 20, and number of plots to 6. Under the field display your name and a list of each plot color in a rectangle of black border and correct color and upper left coordinate. Change the font name and font size from default values to some different font (not ones seen like Courier New or Times New Roman) Assignment 2 Field Plot by Student (38.0,27.0) (24.0.1560) (1700.101.0) (1040.93.0) (6701410) O(65.0,76.0) Part II. (80 pts.) Write a program to solve the following problem. Use the code written for Assignment #1 and display the field of plots using JavaFX. Use an image of a field with a brown fence around it. Plots can be solid colored rectangles of different colors. Set the dimensions to 200, plot dimensions to 20, and number of plots to 6. Under the field display your name and a list of each plot color in a rectangle of black border and correct color and upper left coordinate. Change the font name and font size from default values to some different font (not ones seen like Courier New or Times New Roman) Assignment 2 Field Plot by Student (38.0,27.0) (24.0.1560) (1700.101.0) (1040.93.0) (6701410) O(65.0,76.0)Step by Step Solution
There are 3 Steps involved in it
Step: 1
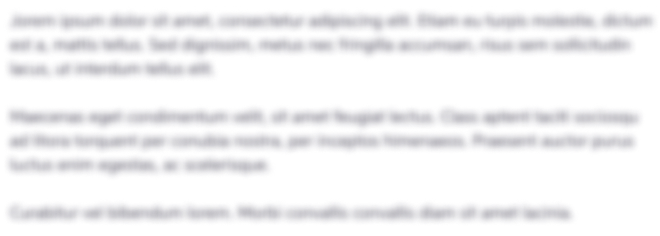
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started