Question
I have to write a testing program for parenthesis. For example, the brackets must close in the correct order, () and ()[]{} are all valid
I have to write a testing program for parenthesis. For example, the brackets must close in the correct order, "()" and "()[]{}" are all valid but "([])" is not.
Here is my program.
bool isValid(string s)
{
stack
for (int i = 0; i < s.size(); i++)
{
if(s[i] == '(' || s[i] == '[' || s[i] == '{' )
parenthesis.push(s[i]);
else
{
if(parenthesis.empty())
return false;
if(s[i] == ')' && parenthesis.top() != '(')
return false;
if(s[i] == ']' && parenthesis.top() != '[')
return false;
if(s[i] == '}' && parenthesis.top() != '{')
return false;
parenthesis.pop();
}
}
return parenthesis.empty();
}
Here is the main program:
int main()
{
string s;
cout << "Input string" << endl;
cin >> s;
if(isValid(s))
cout << "Valid" << endl;
else
cout << "Invalid" << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
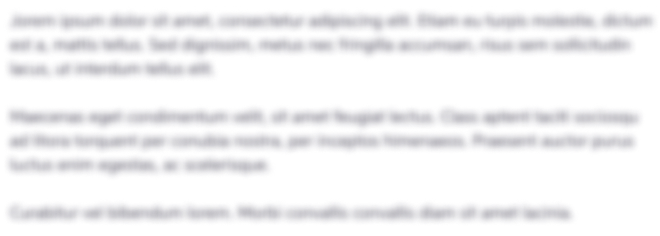
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started