Question
I I really need help with this assignment public interface Polynomial { /** * Returns coefficient of the term with 'power' * @param power *
I
I really need help with this assignment
public interface Polynomial {
/**
* Returns coefficient of the term with 'power'
* @param power
* @return
*/
public double coefficient(int power);
/**
* Returns the degree of polynomial (i.e. largest power of a non-zero term)
* @return
*/
public int degree();
/**
* Add a term to the polynomial
* @param power
* @param coefficient
* @throws Exception when the power is invalid (e.g. negative)
*/
public void addTerm(int power, double coefficient) throws Exception;
/**
* Remove the term corresponding to the power from the polynomial
* @param power
*/
public void removeTerm(int power);
/**
* Returns evaluation of the polynomial at 'point'
* @param point
* @return
*/
public double evaluate(double point);
/**
* Returns a new polynomial that is the result of addition of polynomial p to
this polynomial
* @param p
* @return
*/
public Polynomial add(Polynomial p);
/**
* Returns a new polynomial that is the result of subtraction of polynomial p
to this polynomial
* @param p
* @return
*/
public Polynomial subtract(Polynomial p);
/**
* Returns a new polynomial that is the result of multiplication of polynomial
p with this polynomial
* @param p
* @return
*/
public Polynomial multiply(Polynomial p);
}
public class ArrayPolynomial implements Polynomial {
// maximum degree of a polynomial
public static final int MAX_DEGREE = 100;
// coefficient array
private double [] coefficients = new double[MAX_DEGREE+1];
// Default constructor
public ArrayPolynomial() {}
/**
* Create a single term polynomial
* @param power
* @param coefficient
* @throws Exception when power is negative or exceeds MAX_DEGREE
*/
public ArrayPolynomial(int power, double coefficient) throws Exception {
if (power MAX_DEGREE) {
throw new Exception("Invalid power for the term");
}
/**
* Complete code here for lab assignment
*/
}
/**
* Create a new polynomial that is a copy of "another".
* NOTE : you should use only the interface methods of Polynomial to get
* the coefficients of "another"
*
* @param another
* @throws Exception when degree of another exceeds MAX_DEGREE
*/
public ArrayPolynomial(Polynomial another) throws Exception {
/**
* Complete code here for lab assignment
*/
}
@Override
public void addTerm(int power, double coefficient) throws Exception {
/**
* Complete code here for lab assignment
* Make sure you check power for validity !!
*/
}
@Override
public void removeTerm(int power) {
/**
* Complete code here
* Make sure you check power for validity !!
*/
}
@Override
public double coefficient(int power) {
/**
* Complete code here for lab assignment (Modify return statement !!)
* Make sure you check power for validity !!
*/
return 0;
}
@Override
public int degree() {
/**
* Complete code here for lab assignment
*/
return 0;
}
@Override
public double evaluate(double point) {
double value = 0.0;
/**
* Complete code here for homework
*/
return value;
}
@Override
public Polynomial add(Polynomial p) {
Polynomial result = new ArrayPolynomial();
/**
* Complete code here for homework
*/
return result;
}
@Override
public Polynomial subtract(Polynomial p) {
Polynomial result = new ArrayPolynomial();
/**
* Complete code here for homework
*/
return result;
}
@Override
public Polynomial multiply(Polynomial p) {
Polynomial result = new ArrayPolynomial();
/**
* Complete code here for homework
*/
return result;
}
public String toString() {
StringBuilder builder = new StringBuilder();
int degree = degree();
if (degree == 0) {
return "" + coefficients[0];
}
for (int i=degree; i >=0; i--) {
if (coefficients[i] == 0) {
continue;
}
if (i
builder.append(coefficients[i] > 0 ? " + " : " - ");
builder.append(Math.abs(coefficients[i]));
} else {
builder.append(coefficients[i]);
}
if (i > 0) {
builder.append("x");
if (i > 1) {
builder.append("^" + i);
}
}
}
return builder.toString();
}
/**
* Returns true if the object obj is a Polynomial and its coefficients are the
same
* for all the terms in the polynomial
* @param obj
* @return true or false
*/
@Override
public boolean equals(Object obj) {
/**
* Complete code here for lab assignment
* Note : Cast obj to Polynomial (not ArrayPolynomial) to check if
* coefficients are the same for all the powers in the polynomial
*/
return false;
}
public static void main(String [] args) throws Exception {
// Remove comments in code after you have implemented all the functions in
// homework assignment
Polynomial p1 = new ArrayPolynomial();
System.out.println("p1(x) = " + p1);
assert p1.degree() == 0;
assert p1.coefficient(0) == 0;
assert p1.coefficient(2) == 0;
assert p1.equals(new ArrayPolynomial());
Polynomial p2 = new ArrayPolynomial(4, 5.6);
p2.addTerm(0,3.1);
p2.addTerm(3,2.5);
p2.addTerm(2,-2.5);
System.out.println("p2(x) = " + p2);
assert p2.degree() == 4;
assert p2.coefficient(2) == -2.5;
assert p2.toString().equals("5.6x^4 + 2.5x^3 - 2.5x^2 + 3.1");
// System.out.println("p2(1) = " + p2.evaluate(1));
// assert p2.evaluate(1) == 8.7;
Polynomial p3 = new ArrayPolynomial(0, -4);
p3.addTerm(5,3);
p3.addTerm(5,-1);
System.out.println("p3(x) = " + p3);
assert p3.degree() == 5;
assert p3.coefficient(5) == 2;
assert p3.coefficient(0) == -4;
// System.out.println("p3(2) = " + p3.evaluate(2));
// assert p3.evaluate(2) == 60;
Polynomial p21 = new ArrayPolynomial(p2);
System.out.println("p21(x) = " + p21);
assert p21.equals(p2);
// p21.removeTerm(4);
// System.out.println("p21(x) = " + p21);
// assert !p21.equals(p2);
// assert p21.coefficient(4) == 0;
try {
Polynomial p5 = new ArrayPolynomial(-5, 4);
assert false;
} catch (Exception e) {
// Exception expected
assert true;
}
// System.out.println("p2(x) + p3(x) = " + p2.add(p3));
// Polynomial result = p2.add(p3);
// assert result.degree() == 5;
// assert Math.abs(result.coefficient(5) - 2)
// System.out.println("p2(x) - p3(x) = " +p2.subtract(p3));
// result = p2.subtract(p3);
// assert result.degree() == 5;
// assert Math.abs(result.coefficient(5) - -2)
// assert Math.abs(result.coefficient(0) - 7.1)
// System.out.println("p2(x) * p3(x) = " +p2.multiply(p3));
// result = p2.multiply(p3);
// assert result.degree() == 9;
// assert Math.abs(result.coefficient(9) - 11.2)
// assert Math.abs(result.coefficient(5) - 6.2)
// assert Math.abs(result.coefficient(0) - -12.4)
// assert Math.abs(p2.evaluate(1) * p3.evaluate(1) - result.evaluate(1))
0.0001;
}
}
Lab Assignment 2 Polynomial ADT In this lab assignment, you will be implementing a part of ArrayPloynomial.java that is a specific implementation of Polynomial ADT mentioned in homework assignment 2. In this implementation you will be using an array to store the coefficients where the k-th position in the array contains coefficient of rk in the polynomial. It will be zero if xk term does not exist in the polynomial. You are provided a starter file for ArrayPolynomial.java with data members defined. You need to complete the following (non-default) constructors: public ArrayPolynomial (int power, double coefficient) throws Exception {.... } public ArrayPolynomial (Polynomial another) throws Exception {.. } You also need to complete the following functions: public void addTerm (int power, double coefficient) throws Exception {.. } public double coefficient (int power) {.. } public int degree () {.. } public boolean equals (Object other) {.. } Make sure you run ArrayPolynomial.java with the JVM option "-ea" in order to enable assertions. Array Polynomial Lab Assignment 2 Polynomial ADT In this lab assignment, you will be implementing a part of ArrayPloynomial.java that is a specific implementation of Polynomial ADT mentioned in homework assignment 2. In this implementation you will be using an array to store the coefficients where the k-th position in the array contains coefficient of rk in the polynomial. It will be zero if xk term does not exist in the polynomial. You are provided a starter file for ArrayPolynomial.java with data members defined. You need to complete the following (non-default) constructors: public ArrayPolynomial (int power, double coefficient) throws Exception {.... } public ArrayPolynomial (Polynomial another) throws Exception {.. } You also need to complete the following functions: public void addTerm (int power, double coefficient) throws Exception {.. } public double coefficient (int power) {.. } public int degree () {.. } public boolean equals (Object other) {.. } Make sure you run ArrayPolynomial.java with the JVM option "-ea" in order to enable assertions. Array PolynomialStep by Step Solution
There are 3 Steps involved in it
Step: 1
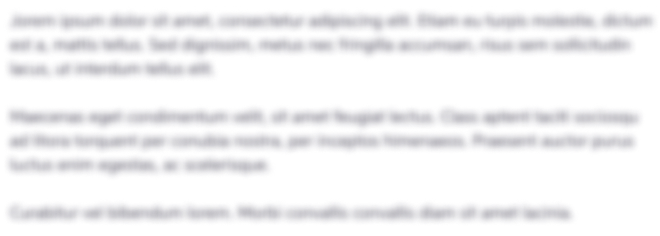
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started