Question
I just need the code for rectangle_eq(self, other), rectangle_and(self, other), rectangle_contains(self, p), region_contains(self, p), region_bounding_box(self), rectangle_random_point(self), region_montecarlo_volume(self, n=1000), and region_montecarlo_difference(self, other, n=1000) with the correct
I just need the code for rectangle_eq(self, other), rectangle_and(self, other), rectangle_contains(self, p), region_contains(self, p), region_bounding_box(self), rectangle_random_point(self), region_montecarlo_volume(self, n=1000), and region_montecarlo_difference(self, other, n=1000) with the correct parameters for each of them. It should pass the tests assigned for each of them.
def rectangle_eq(self, other):
### YOUR CODE HERE
Rectangle.__eq__ = rectangle_eq
def rectangle_and(self, other):
if self.ndims != other.ndims:
raise TypeError("The rectangles have different dimensions: {} and {}".format(
self.ndims, other.ndims
))
# Challenge: can you write this as a one-liner shorter than this comment is?
# There are no bonus points, note. Just for the fun.
### YOUR CODE HERE
Rectangle.__and__ = rectangle_and
def rectangle_contains(self, p):
# The point is a tuple with one element per dimension of the rectangle.
if len(p) != self.ndims:
raise TypeError()
### YOUR CODE HERE
Rectangle.__contains__ = rectangle_contains
def region_contains(self, p):
### YOUR CODE HERE
Region.__contains__ = region_contains
def region_bounding_box(self):
"""Returns the bounding box of the region, as a rectangle.
This returns None if the region does not contain any rectangle."""
if len(self.rectangles) == 0:
return None
### YOUR CODE HERE
Region.bounding_box = property(region_bounding_box)
def rectangle_random_point(self):
### YOUR CODE HERE
Rectangle.random_point = rectangle_random_point
def region_montecarlo_volume(self, n=1000):
"""Computes the volume of a region, using Monte Carlo approximation
with n samples."""
# The solution, written without any particular trick, takes 7 lines.
# If you write a much longer solution, you are on the wrong track.
### YOUR CODE HERE
Region.montecarlo_volume = region_montecarlo_volume
def region_montecarlo_difference(self, other, n=1000):
"""Checks whether a region self is different from a region other, using
a Monte Carlo method with n samples. It returns either a point p that
witnesses the difference of the regions, or None, if no such point is found."""
# This can be done without hurry in 6 lines of code.
### YOUR CODE HERE
Region.montecarlo_difference = region_montecarlo_difference
def region_montecarlo_equality(self, other, n=1000):
return self.montecarlo_difference(other, n=n) is None
Region.montecarlo_equality = region_montecarlo_equality
1 import string 2 3 class Rectangle(object): 4 5 def __init__(self, *intervals, name=None): 6 A rectangle is initialized with a list, whose elements are 7 either Interval, or a pair of numbers. 8 It would be perhaps cleaner to accept only list of intervals, 9 but specifying rectangles via a list of pairs, with each pair le defining an interval, makes for a concise shorthand that will be 121 useful in tests. 12 Every rectangle has a name, used to depict it. 13 If no name is provided, we invent a random one." 14 self.intervals = [] 15 for i in intervals: self.intervals.append(i if type(i) == Interval else Interval("i)) 17 # I want each rectangle to have a name. 18 if name is None: 19 self.name = ".join( 20 random.choices(string.ascii_letters + string.digits, k=8)) 21 else: 22 self.name = name 23 def repr_(self): 25 Function used to print a rectangle." 26 s = Rectangle " + self.name + ":" 27 5 + repr([(*.xe, i.xl) for i in self.intervals]) 28 returns 29 3e def clone(self, name=None): 31 Returns a clone of itself, with a given name. 32 name = name or self.name + 33 return Rectangle(*self.intervals, name=name) 34 35 def _len_(self): 36 ***"Returns the number of dimensions of the rectangle 37 (not the length of the edges). This is used with 38 ___getitem_. below, to get the interval along a dimension. 39 return len(self.intervals) def 41 42 43 44 getitem_(self, n): ***Returns the interval along the n-th dimension" return self.intervals[n] 45 46 47 48 def -setitem_(self, n, 1): ***Sets the interval along the n-th dimension to be i self.intervals[n] = i @property def ndims (self): Returns the number of dimensions of the interval." return len(self. intervals) 49 50 51 52 53 54 55 56 57 @property def volume (self): return np.prod([1. length for i in self.intervals]) 1 class Region (object): def 5 6 _init__(self, *rectangles, name=None): "A region is initialized via a set of rectangles. """" self.rectangles = list(rectangles) if name is None: self.name = "join random.choices(string.ascii_letters + string.digits, k=8)) else: self.name = name S 10 112 def draw(self): draw_rectangles (*self.rectangles, prefix=self.name + ":") 13 15 def or _(self, other): Union of regions. **** return Region(*(self.rectangles + other.rectangles), name=self.name + "_union_" + other.name) 17 20 1 def rectangle_eq(self, other): * YOUR CODE HERE 4 Rectangle. _eQ__ = rectangle_eq I] 1 # 5 points: tests for rectangle equality. 3 assert Rectangle((2, 3), (4, 5)) Rectangle((2, 3), (4, 5)) 4 assert Rectangle((2, 3), (4, 5)) != Rectangle((4, 5), (2, 3)) 5 assert Rectangle((2, 3), (4, 5)) != Rectangle((2, 3), (4, 5), (6, 7)) 1 ### Rectangle intersection 4 3 def rectangle_and(self, other): if self.ndims != other.ndims: raise TypeError("The rectangles have different dimensions: O and {}".format( self.ndims, other.ndims )) # Challenge: can you write this as a one-liner shorter than this comment is? # There are no bonus points, note. Just for the fun. ### YOUR CODE HERE 9 12 Rectangle. __and rectangle_and 1 # Let's see how your rectangle intersection works. 2 r1 = Rectangle((2, 3), (2, 4)) 3 r2 = Rectangle(e, 4), (1, 3)) 4 draw_rectangles (ri, r2) 5 draw_rectangles (r1 & r2) 1 # 10 points: tests for rectangle intersection. 2 3 ri = Rectangle((2, 3), (0, 4)) 4 r2 = Rectangle( (2, 4), (1, 3)) 5 assert ri & r2 == Rectangle((2, 3), (1, 3)) 7 -1 = Rectangle((2, 3), (0, 4)) 8 r2 = Rectangle((@, 4), (1, 5)) 9 assert ri & r2 == Rectangle((2, 3), (1, 4)) 10 11 ri = Rectangle((-1, 5), (0, 6)) 12 r2 = Rectangle((, 4), (-1, 3)) 13 assert ri & r2 == Rectangle((e, 4), (, 3)) 15 n1 = Rectangle((2, 6), (0, 4)) 16 r2 = Rectangle((e, 6), (0, 3)) 13 assert ri & r2 == Rectangle((2, 6), (0, 3)) 28 [] 1 *** Membership of a point in a rectangle. 3 def rectangle_contains(self, p): # The point is a tuple with one element per dimension of the rectangle. if len(p) != self.ndims: raise TypeError() * YOUR CODE HERE 9 Rectangle. _contains = rectangle_contains 1# 5 points: tests for membership. 3 assert (2, 3) in Rectangle((0, 4), (1, 5)) 4 assert (e, 4) in Rectangle((0, 4), (4, 5)) 5 assert (4, 5) in Rectangle((@, 4), (4, 5)) 6 assert (e, e, e) not in Rectangle((3, 4), (0, 3), (0, 8)) 7 A point belongs into a region if it belongs into some rectangle of the region. We let you implement this. 1 ### Membership of a point in a region 3 def region_contains(self, p): ### YOUR CODE HERE 6 Region. __contains. 7. region_contains [] un N 1 assert (2, 1) in Region(Rectangle((, 2), (2, 3)), Rectangle((4, 6), (5, 8))) 3 assert (2, 1) not in Region (Rectangle((@, 1), (0, 3)), Rectangle((4, 6), (5, 8))) 4 [ ] 1 ### Compute the bounding box of a region N 3 def region_bounding box(self): ""Returns the bounding box of the region, as a rectangle. This returns None if the region does not contain any rectangle. if len(self.rectangles) return None 3 #*# YOUR CODE HERE 10 Region.bounding_box property(region_bounding_box) [] BE 1 # 10 points: tests for bounding boxes 2 3 reg = Region (Rectangle((0, 2), (1, 3)), Rectangle((4, 6), (5, 8))) 4 assert reg.bounding_box Rectangle((0, 6), (1, 8)) 5 6 reg = Region Rectangle((0, 5), (4, 5), (1, 9)), Rectangle((4, 20), (-2, 3), (4, 21)), Rectangle((7, 99), (3, 7), (2, 3)) 10 11 assert reg.bounding_box Rectangle((@, 99), (-2, 7), (1, 21)) 17 9 [] 1 ### Random point of a rectangle 12 3 def rectangle_random_point(self): ### YOUR CODE HERE 4 6 Rectangle.random_point = rectangle_random_point 1 # 3 points: random point of a rectangle. 3 r = Rectangle((0, 2), (1, 3)) 4 BB von un 5 for i in range(5): 6 P = r.random_point() 7 assert isinstance (p, tuple) 8 assert len() == 2 assert p in r print(p) 1 # 3 points: random point of a rectangle. 2 3 import numpy as np 4 5 r = Rectangle((1, 2), (1, 6)) 6 XS, ys = [], [] 7 for in range (10000): B P = r.random_point) 9 assert p in r 18 xs.append(p[]) ys.append(p[1]) 12 assert np.std(x5) * 4.9 np.stdlys)Step by Step Solution
There are 3 Steps involved in it
Step: 1
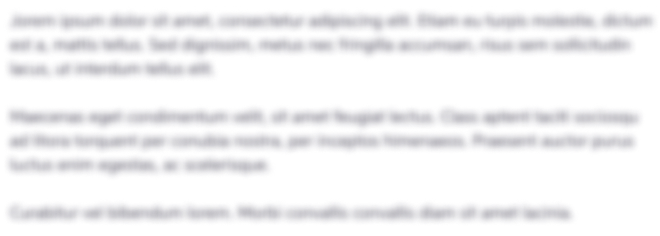
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started