Question
I must use the pseudo-code to below Read Me File: Top-down Approach The top-down approach is a technique of saving values that have already been
I must use the pseudo-code to below
Read Me File:
Top-down Approach
The top-down approach is a technique of saving values that have already been calculated, a technique that is referred to as memoization. With this technique we first break the problem up into sub-problems and then calculate and store values. To facilitate this approach we can use a map structure. A map is also known as an "associative array" in which "keys" are associated with "values." The arrays you have worked with thus far could be thought of as an associative map wherein the "key" is an integer (i.e., the index into the array), and of course, the "value" is the contents of the array at that index (key). Fortunately for us, the standard template library includes such a structure. The program Demo.cpp in this repo demonstrates the use of a map, and is shown below:
#include#include #include #include
Compiling and running (in a Cygwin terminal) this program would look like (where '$' is the command-line prompt and not typed):
$ g++ map_demo.cpp $ ./a.exe John's grade = C After raising his grade, John's grade = B Jim wasn't found in the map grade_list.begin()->first = John grade_list.begin()->second = B Mappings in grade_list: John <-- B Sue <-- A 0 <-- 1 1 <-- 1
For more details and a quick tutorial on using the map found in the STL, see STL Maps -- Associative Arrays.
With this understanding of the map object, let us return to the top-down approach to finding the nth Fibonacci number using a map.
Suppose we have a simple map object, m, which maps each Fibonacci number calculated that has already been calculated to its result, and we modify our function to use it and update it. The resulting function requires only O(n) time instead of exponential time (but requires O(n) space):
// This, of course, is just pseudo-code mapm m[0] = 1 m[1] = 1 function fib(n) if key n is not in map m m[n] = fib(n - 1) + fib(n - 2) return m[n]
$ cd generator $ cmake -G "Unix Makefiles" .. ... lots of output $ make ... more output $ ../out/lab03.exe This program computes the nth Fibonacci number. To end this program, enter a negative value for n. n = -1 Program has ended successfully. Good bye... $ ../out/lab03.exe This program computes the nth Fibonacci number. To end this program, enter a negative value for n. n = 9 Top-down approach: fib(9) = 0 Bottom-up approach: fib(9) = 0 n = 2 Top-down approach: fib(2) = 0 Bottom-up approach: fib(2) = 0 n = -1 Program has ended successfully. Good bye...
Clearly, the values are not being calculated correctly. This is because the functions are merely stubbed out.
Below I must use the above Pseudo-code in the program below. I am completely lost as to what I need to write.
/** | |
* CSC232 Data Structures with C++ | |
* Missouri State University, Spring 2019 | |
* | |
* @file TopDownFibCalculator.cpp | |
* @authors Jim Daehn | |
* Your Name | |
* @brief Definition of TopDownFibCalculator. | |
*/ | |
#include "TopDownFibCalculator.h" | |
int TopDownFibCalculator::nthFibonacciNumber(int n) const { | |
// TODO: Implement me using the algorithm shown in the README.md file of this project. | |
return 0; | |
} |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
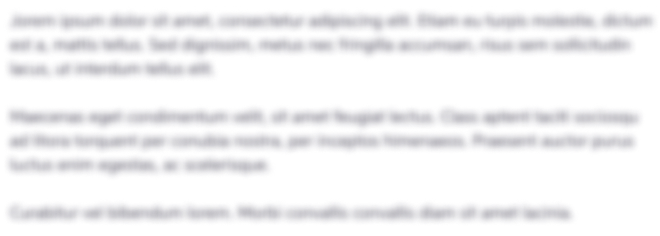
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started