Question
I need a non-leaf function in a MIPS program that determines if an integer(read in as a char) is negative and if so, uses existing
I need a non-leaf function in a MIPS program that determines if an integer(read in as a char) is negative and if so, uses existing function toBinary to convert the absolute value to 2's complement in a string. Should do the following:
Below is the C equivalent of this program:
++++++++++++++++++++++++++++++++++ C Example Code +++++++++++++++++++++++++++++++++++++
#include
#include
// leaf function - convert decimal to binary in string
void toBinary(int num, char strNum[])
{
int i;
for(i = 7; i >= 0; i--){ // index 7 is the LSB (bit-0)
int rem = num & 0x01; // retrieve remainder
num = num >> 1; // shift right by 1 bit (== divide by 2)
strNum[i] = '0' + rem; // ascii '0' is decimal 48, '1' is 49
}
}
// non-leaf function convert decimal (negative) to 2s complement in string
void twosComplement(int num, char strNum[])
{
int i, absolute, carry;
absolute = abs(num);
toBinary(absolute, strNum); // call toBinary for absolute value (positive)
printf("absolute value = %s ", strNum);
// flip, complement
for(i = 7; i >=0; i--){
if(strNum[i] == '0')
strNum[i] = '1';
else
strNum[i] = '0';
}
printf("1's complement = %s ", strNum);
// add 1
for(i = 7, carry = 1; i >=0 && carry; i--){ // if carry is 0, stop!!
int sum = (strNum[i] - 48) + carry; // ascii '0' is decimal 48, '1' is 49
strNum[i] = '0' + (sum & 0x01); // logical and
carry = (sum >> 1) & 0x01; // shift right by 1 bit (divide by 2) & logical and
}
printf("2's complement = %s ", strNum);
}
int main()
{
char Binary[10] = "";
int num;
printf("Enter a number: ");
scanf("%d", &num);
if(num > 127 || num
{
printf("Out of Range for 8-bit singed Binary");
return 0;
}
if(num >= 0) // positive or 0
toBinary(num, Binary);
else // negative to two's complement
twosComplement(num, Binary);
printf("%d's 8-bit Binary Representation is %s ",num , Binary);
return 0;
}
o Write a non-leaf function to convert a negative integer number to 2's complement in string Two parameters: integer number, string array or pointer Get absolute value of the negative number Call a function that you implement from part I .Flip each binary bit of the string (do 1's complement): '0' f-> 1' -Add 1 to LSB Repeat adding a carry to higher bitStep by Step Solution
There are 3 Steps involved in it
Step: 1
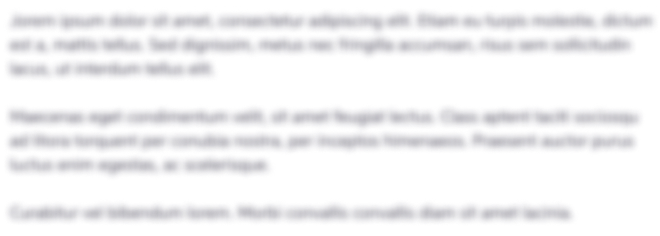
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started