Question
I need a program that is a new version of the area calculation program below that makes use of inheritance in C++. It needs to
I need a program that is a new version of the area calculation program below that makes use of inheritance in C++. It needs to add a new Shape base class to the area calculation program that includes data members common to all shapes (such as a shape ID, a shape type, and a unit of measure). The Shape base class should also include a virtual getArea() member function. Revise the Circle and Square classes so that they inherit from the Shape base class. Also, it needs to add a third shape to the program that also inherits from the Shape class.
The finished program should ask the user to enter the relevant information for each shape and then print the area and other information for each shape.
THE CODE NEEDS to be organized into header (.h) and implementation (.cpp) files.
I need the new code to be like the one I have posted with header file example circle.h and implementation files example circle.cpp
My original code is below:
CODE :
circle.h :
#ifndef CIRCLE_H #define CIRCLE_H
struct Circle { // circle structure class
public: Circle(); // declaration void setRadius(double r); // setting the radius double getRadius(); // getting the radius double Area(); // get the area calculated void Display(); // display the datda
protected: double Radius; // radius value declared
private:
};
#endif
square.h :
#ifndef SQUARE_H #define SQUARE_H
struct Square { // square structure class
public : Square(); // declaration void setLength(double l); // setting the length double getLength(); // getting the length double Area(); // get the area calculated void Display(); // display the data
protected : double Length; // length value declaration
private: };
#endif
circle.cpp :
#include
using namespace std;
const double PI = 3.14159; // initialisng the global values Circle::Circle() { // method declaration
Radius = 0; // initialisng the radius value
}
void Circle::setRadius(double r) {
Radius = r <= 0 ? 1 : r; // checking the input value
}
double Circle::getRadius() {
return Radius; //getting and setting the value
}
double Circle::Area(){ return Radius * Radius * PI; // returning the area }
void Circle::Display(){ // display the data
cout << " Circle Properties"; cout << " Radius = " << getRadius(); cout << " Area = " << Area() << " "; }
square.cpp :
#include
using namespace std;
Square::Square(){ // method declaration
Length = 0; // length initialisng
}
void Square::setLength(double l) {
Length = l <= 0 ? 1 : l; // checking the data
}
double Square::getLength() {
return Length; // returning the length
}
double Square::Area() {
return Length*4; // returning the area
} void Square::Display(){ // display the data
cout << " Square Properties"; cout << " Length = " << getLength(); cout << " Area = " << Area() << " "; }
main.cpp :
#include "circle.h" #include
using namespace std;
int main(){
int radius,length;
Circle circle; // Displaying a circle using the default value of the radius circle.Display(); Circle circ; // circle with a value supplied for the radius
cout << "Enter the radius value :" << endl; // taking the value from the user cin >> radius;
circ.setRadius(radius); circ.Display();
Square square; // Displaying a square square.Display(); Square squ; // square with a value supplied for the length
cout << "Enter the length value :" << endl; cin >> length;
squ.setLength(length); // of the base class squ.Display();
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
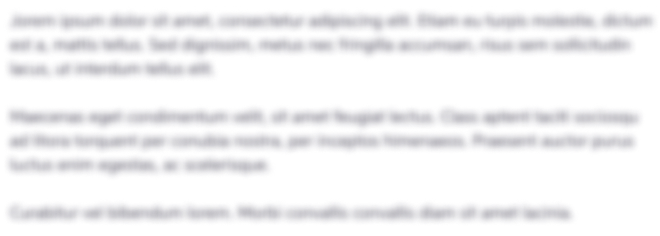
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started