Question
I need assistance in figuring out what is wrong with my C++code. I have written a function to update the values in my array but
I need assistance in figuring out what is wrong with my C++code. I have written a function to update the values in my array but when I run the program the values do not increment. They stay the same. Here are the assignment details. My code is posted below the assignment details. Please help me figure out how to get my function addMedals to do what it is supposed to do. Thanks.
Your program should use a structure named Country that keeps the following data for a given country (use the provided member names):
Country Name (name)
Number of gold medals awarded (gold)
Number of silver medals awarded (silver)
Number of bronze medals awarded (bronze)
The program should maintain an array of 8 countries, initialized with the following data.
Country | Gold | Silver | Bronze |
---|---|---|---|
Australia | 11 | 14 | 12 |
Canada | 5 | 0 | 1 |
China | 9 | 14 | 11 |
Great Britain | 8 | 4 | 8 |
Japan | 8 | 10 | 10 |
Netherlands | 7 | 6 | 7 |
Russia | 6 | 10 | 8 |
USA | 10 | 6 | 7 |
When the program runs, it should repeat a loop that performs the following steps:
Print a numbered list of the Countries with column headers and corresponding data for each country on a separate row (see "Sample execution" below for the exact format). The "Total" column records the total number of medals awarded to that country. Use the following code to print the header. Infer the formatting of each row from this code.
cout << left << setw(3) << "N" << setw(15) << "Country" << right << setw(10) << "Gold" << setw (10) << "Silver" << setw (10) << "Bronze" << setw(10) << "Total" << endl;
The user is prompted enter the country number or quit (enter 0 to quit)
If the country number is not 0, then the user is prompted to enter the medal type awarded (G for gold, S for silver, and B for bronze). And, if the input is valid, the medal count for the indicated country is incremented appropriately.
The loop repeats until the user enters 0 to quit.
When the user quits the program, the program should display a message indicating the total medals awarded to all countries, and which country has the most Gold medals.
Your program must include these four functions
showResults to display the table of countries, given the array of Countries.
addMedal to update the medal counts: given the array of Countries, a country number, and a medal character, it increments the appropriate medal count. This function should NOT contain a loop, it should NOT do any input/output. The main program loop should be in main. If the country number or medal character is invalid it should do nothing (not output an error message).
totalMedals to determine the total number of medals awarded, given the array of Countries, it should NOT do any input/output. It must return an int value.
mostGoldMedals to determine which country has the most gold medals, given the array of Countries, it should NOT do any input/output. It must return the index of the country with the most gold medals. It must return an int value.
Sample execution:
N Country Gold Silver Bronze Total 1 Australia 11 14 12 37 2 Canada 5 0 1 6 3 China 9 14 11 34 4 Great Britain 8 4 8 20 5 Japan 8 10 10 28 6 Netherlands 7 6 7 20 7 Russia 6 10 8 24 8 USA 10 6 7 23 Enter the country number (0 to quit): 2 Enter the medal type (G,S, or B): S N Country Gold Silver Bronze Total 1 Australia 11 14 12 37 2 Canada 5 1 1 7 3 China 9 14 11 34 4 Great Britain 8 4 8 20 5 Japan 8 10 10 28 6 Netherlands 7 6 7 20 7 Russia 6 10 8 24 8 USA 10 6 7 23 Enter the country number (0 to quit): 0 Total medals Awarded: 193 Country with the most Gold Medals: Australia
IMPORTANT: Your output must match exactly.
Additional Requirements:
You must use the identifiers specified above to name your structure, members, and functions, and you must have the proper parameter types in the proper order, in order to pass the unit tests (Automatic tests 13-16).
Use a named constant for the array size.
If more than one country has the most gold medals, your program should output the first one.
Do not use any features of C++ that we have not yet covered in class (use features from Chapters 1-11). Do not use classes!
Style: See the Style Guidelines document on Canvas under Files. Especially pay attention to the comments required for the top of the file and for each function.
You must submit a program for grading by midnight Friday Jan 27 (worth 5pts). It must be a reasonable attempt (try passing just the first test case, see steps 1 and 2 in the Incremental Development section below).
Logistics
Use any IDE (codeblocks, Xcode, CLion, replit.com, etc) to develop your code.
Drag "main.cpp" file or select it from your disk. Make sure that the file name is correct (otherwise it will not upload). Click submit for grading. If some tests have failed, check the difference of your output to the correct one and then change your program accordingly. Then drag and submit for grading again. Check "Only show failing tests" to hide passed tests.
Incremental Development
Develop program gradually, one piece at a time.
Submit often! Make sure that your output matches the expected output in tests, BEFORE you add any more code.
Your program must pass at least 1 test case for your program to be graded!
Not sure how to do incremental development? Follow the steps below:
Write the display table function.
Write main: initialize the array of Country struct, call the display table function, prompt for the country number and input the country number. Test for yourself, then submit for grading and modify your code to pass Test 1.
Write the function to determine the total number of medals awarded, add the function call to main and output the result. submit for grading and modify your code to pass Test 2.
Write the function to determine which country has the most gold medals, add the function call to main and output the results appropriately. submit for grading and modify your code to pass Test 3.
Now write the function to update the medal counts. It has the country number and medal letter as parameters (don't input them from the user inside the function, that happens in main). All this function does is the following: checks that the country number and the medal letter are valid, and if so, adds one to the appropriate medal count in the array.
In main, add code to: prompt the user to input the medal type and input it. Call the function to update the medal counts. Don't add the loop yet, but copy paste the code to prompt + input the next country code before outputting the total count and leading country. submit for grading and modify your code to pass Test 4.
Now you can add the loop in the main function so that it properly continues to read in the country code and (if it's not 0) input the medal type, calls functions appropriately, and exits the loop if the country code is 0. submit for grading and modify your code to pass the remaining tests.
#include
void showResults(Country country[]); void addMedal(Country country[], int, char, char, char); int totalMedals(Country country[], int); int mostGoldMedals(Country country[], int);
int main() { int number; int gold; int num; char type; char G; char S; char B; Country country[SIZE] = { {"Australia", 11, 14, 12}, {"Canada", 5, 0, 1}, {"China", 9, 14, 11}, {"Great Britain", 8, 4, 8}, {"Japan", 8, 10, 10}, {"Netherlands", 7, 6, 7}, {"Russia", 6, 10, 8}, {"USA", 10, 6, 7}}; do {showResults(country); cout << "Enter the country number (0 to quit):" << endl; cin >> num; if (num==0) break; cout << "Enter the medal type (G,S, or B):"<
addMedal(country,number, G, S, B);
}while (num > 0); cout << "Total medals Awarded: " << totalMedals(country, number); cout << endl;
cout << "Country with the most Gold Medals: "<< country [mostGoldMedals(country, gold)-1].name; cout << endl;
} void showResults(Country country[]) { cout << left << setw(3) << "N" << setw(15) << "Country" << right << setw(10) << "Gold" << setw(10) << "Silver" << setw(10) << "Bronze" << setw(10) << "Total" << endl; for (int i = 0; i < SIZE; i++) { cout << left << setw(3) << i + 1 << setw(15) << country[i].name << right << setw(10) << country[i].gold << setw(10) << country[i].silver << setw(10) << country[i].bronze << setw(10) << country[i].gold + country[i].silver + country[i].bronze << endl; } } int totalMedals(Country country[], int total) { total = 0; for (int i = 0; i < SIZE; i++) { total = total + country[i].gold + country[i].silver + country[i].bronze; } return total; } int mostGoldMedals(Country country[], int count) { int most = 0; count=0; for (int i = 0; i < SIZE; i++) { if (country[i].gold > most) { most = country[i].gold; count ++; } } return count; } void addMedal(Country country [],int number, char G,char S, char B){ char type; if (number==1) { if (type == G) { country[0].gold = country[0].gold + 1;} else if (type == S) {country[0].silver =country[0].silver + 1;} else {country[0].bronze = country[0].bronze + 1;} } else if (number==2){ if (type == G) { country[1].gold = country[1].gold + 1;} else if (type == S) {country[1].silver = country[1].silver + 1;} else {country[1].bronze = country[1].bronze + 1 ;} } else if (number==3){ if (type == G) { country[2].gold = country[2].gold + 1;} else if (type == S) {country[2].silver = country[2].silver + 1;} else {country[2].bronze = country[2].bronze + 1 ;} } else if (number==4){ if (type == G) { country[3].gold = country[3].gold + 1;} else if (type == S) {country[3].silver = country[3].silver + 1;} else {country[3].bronze = country[3].bronze + 1 ;} } else if (number==5){ if (type == G) { country[4].gold = country[4].gold;} else if (type == S) {country[4].silver = country[4].silver;} else {country[4].bronze = country[4].bronze + 1;} } else if (number==6){ if (type == G) { country[5].gold = country[5].gold + 1;} else if (type == S) {country[5].silver = country[5].silver + 1;} else {country[5].bronze = country[5].bronze + 1;} } else if (number==7){ if (type == G) { country[6].gold = country[6].gold + 1;} else if (type == S) {country[6].silver = country[6].silver + 1;} else {country[6].bronze = country[6].bronze + 1;} } else if (number==8){ if (type == G) { country[7].gold = country[7].gold + 1;} else if (type == S) {country[7].silver = country[7].silver + 1;} else {country[7].bronze = country[7].bronze + 1;} } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
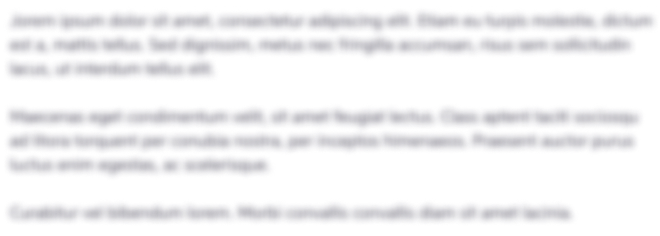
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started