Question
I need assistance understanding where my code is wrong (entire code for the 3 projects is listed after the errors). When compiling, I receive the
I need assistance understanding where my code is wrong (entire code for the 3 projects is listed after the errors). When compiling, I receive the following errors:
----jGRASP exec: javac -g DodecahedronListMenuApp.java
DodecahedronList.java:198: error: class, interface, or enum expected
public String summaryInfo() {
^
DodecahedronList.java:201: error: class, interface, or enum expected
String result = "----- Summary for " + getName()
^
DodecahedronList.java:216: error: class, interface, or enum expected
return result;
^
DodecahedronList.java:217: error: class, interface, or enum expected
}
^
DodecahedronListMenuApp.java:71: error: cannot find symbol
System.out.println(" " + dodObjList.summaryInfo() + " ");
^
symbol: method summaryInfo()
location: variable dodObjList of type DodecahedronList
5 errors
----jGRASP wedge: exit code for process is 1.
----jGRASP: operation complete.
***PLEASE SEE BELOW FOR FULL JAVA CODE***
Dodecahedron.java
1 import java.text.DecimalFormat;
2 /**
3 * This program creates a class called Dodecahedron that stores the
4 * label, color, and edge.
5 *
6 *
7 *
8 *
9 */
10
11 public class Dodecahedron {
12
13 // Create instance variables and initialize.
14 private String label = " ";
15 private String color = " ";
16 private double edge = 0;
17
18 // Create constructor Dodecahedron.
19 /**
20 * @param labelIn to replace label
21 * @param colorIn to replace color
22 * @param edgeIn to replace edge
23 */
24
25 public Dodecahedron(String labelIn, String colorIn, double edgeIn) {
26 setLabel(labelIn);
27 setColor(colorIn);
28 setEdge(edgeIn);
29 }
30
31 // Create methods.
32 /**
33 * Create method for getLabel.
34 * @return label
35 */
36 public String getLabel() {
37 return label;
38 }
39
40 /**
41 * Create method for setLabel.
42 * @param labelIn for label
43 * @return true if labelIn is not null, false otherwise.
44 */
45 public boolean setLabel(String labelIn) {
46 if (labelIn != null) {
47 6label = labelIn.trim();
48 6return true;
49 6}
50 else {
51 return false;
52 }
53 }
54
55 /**
56 * Create method for getColor.
57 * @return color.
58 */
59 public String getColor() {
60 return color;
61 }
62
63 /**
64 * Create method for setColor.
65 * @param colorIn for color.
66 * @return true if colorIn is not null, false otherwise.
67 */
68 public boolean setColor(String colorIn) {
69 if (colorIn != null) {
70 6color = colorIn.trim();
71 6return true;
72 6}
73 else {
74 return false;
75 }
76 }
77 /**
78 * Create method for getEdge.
79 * @return edge.
80 */
81 public double getEdge() {
82 return edge;
83 }
84
85 /**
86 * Create method for setEdge.
87 * @param edgeIn for edge.
88 * @return true if edgeIn greater than 0, false otherwise.
89 */
90 public boolean setEdge(double edgeIn) {
91 if (edgeIn > 0) {
92 6edge = edgeIn;
93 6return true;
94 6}
95 else {
96 return false;
97 }
98 }
99
100 /**
101 * Create method that calculates surface area.
102 * @return surfaceArea.
103 */
104 public double surfaceArea() {
105 double surfaceArea = ((3 * Math.sqrt(25 + 10 * (Math.sqrt(5))))
106 * (Math.pow(edge, 2)));
107 return surfaceArea;
108 }
109 /**
110 * Create method that calculates volume.
111 * @return volume.
112 */
113 public double volume() {
114 double volume = ((15 + (7 * Math.sqrt(5))) / 4) * (Math.pow(edge, 3));
115 return volume;
116 }
117
118 /**
119 * Create method that calculates surface to volume ratio.
120 * @return surfacetoVolumeRatio.
121 */
122 public double surfaceToVolumeRatio() {
123 double surfaceToVolumeRatio = surfaceArea() / volume();
124 return surfaceToVolumeRatio;
125 }
126
127 /**
128 * Create method that returns a string.
129 * @return formatted string.
130 */
131 public String toString() {
132 DecimalFormat fmt = new DecimalFormat("#,##0.0##");
133 return "Dodecahedron \"" + getLabel() + "\" is \"" + getColor()
134 + "\" with 30 edges of length " + getEdge()
135 + " units. \tsurface area = " + fmt.format(surfaceArea())
136 + " square units \tvolume = " + fmt.format(volume())
137 + " cubic units \tsurface/volume ratio = "
138 + fmt.format(surfaceToVolumeRatio());
139 }
140 }
DodecahedronList.java
1 /**
2 *
3 *
4 *
5 */
6
7 import java.util.ArrayList;
8 import java.text.DecimalFormat;
9 import java.io.File;
10 import java.io.IOException;
11 import java.util.Scanner;
12
13 /** setting up the class dodecahedronlist.
14 * for mod 5 project.
15 */
16
17 public class DodecahedronList {
18 private String listName = "";
19 private ArrayList
20
21 /**
22 * Constructor for the DodecahedronList class.
23 *
24 * @param listNameIn User input for the name of the list.
25 * @param dListIn Represents the list of Dodecahedron objects.
26 */
27 public DodecahedronList(String listNameIn, ArrayList
28 listName = listNameIn;
29 dList = dListIn;
30 }
31
32 /**
33 * The getName method returns a String representing the name of the list.
34 *
35 * @return listName User input for the name of the list.
36 */
37 public String getName() {
38 return listName;
39 }
40
41 public int numberOfDodecahedrons() {
42 if (dList.size() != 0) {
43 6return dList.size();
44 6}
45 else {
46 return 0;
47 }
48 }
49
50 public double totalSurfaceArea() {
51 double totalSurfaceArea = 0;
52
53 if (dList.size() != 0) {
54 6int index = 0;
55 6while (index < dList.size()) {
56 67totalSurfaceArea += dList.get(index).surfaceArea();
57 67index++;
58 6}
59 6}
60 else {
61 return 0;
62 }
63 return totalSurfaceArea;
64 }
65
66 public double totalVolume() {
67 double totalVolume = 0;
68
69 if (dList.size() != 0) {
70 6int index = 0;
71 6while (index < dList.size()) {
72 67totalVolume += dList.get(index).volume();
73 67index++;
74 6}
75 6}
76 else {
77 return 0;
78 }
79 return totalVolume;
80 }
81
82
83 public double averageSurfaceArea() {
84 double averageSurfaceArea = 0;
85
86 if (dList.size() == 0) {
87 6return 0;
88 }
89 averageSurfaceArea = totalSurfaceArea() / dList.size();
90 return averageSurfaceArea;
91 }
92
93 public double averageVolume() {
94 double averageVolume = 0;
95
96 if (dList.size() == 0) {
97 6return 0;
98 }
99 averageVolume = totalVolume() / dList.size();
100 return averageVolume;
101 }
102
103
104 public double averageSurfaceToVolumeRatio() {
105 double averageSurfaceToVolumeRatio = 0;
106 double tSurfaceToVolumeRatio = 0;
107 int index = 0;
108
109 while (index < dList.size()) {
110 7tSurfaceToVolumeRatio += dList.get(index).surfaceToVolumeRatio();
111 7index++;
112 }
113
114 if (dList.size() != 0) {
115 6averageSurfaceToVolumeRatio = tSurfaceToVolumeRatio / dList.size();
116 6}
117 else {
118 return 0;
119 }
120 return averageSurfaceToVolumeRatio;
121 }
122
123 public String toString() {
124 String result = listName + " ";
125
126 int index = 0;
127 while (index < dList.size()) {
128 7result += " " + dList.get(index) + " ";
129 7index++;
130 }
131 return result;
132 }
133
134 public ArrayList
135 return dList;
136 }
137
138 public DodecahedronList readFile(String sFileNameIn) throws IOException {
139 Scanner scanFile = new Scanner(new File(sFileNameIn));
140 ArrayList
141 String dListName = "";
142
143 listName = scanFile.nextLine();
144
145 while (scanFile.hasNext()) {
146 String label = scanFile.nextLine();
147 String color = scanFile.nextLine();
148 double edge = Double.parseDouble(scanFile.nextLine());
149
150 Dodecahedron d = new Dodecahedron(label, color, edge);
151 myNewList.add(d);
152 }
153 DodecahedronList dL = new DodecahedronList(listName, myNewList);
154 return dL;
155 }
156
157 public void addDodecahedron(String sLabelIn,
158 String sColorIn, double dEdgeIn) {
159 Dodecahedron d = new Dodecahedron(sLabelIn, sColorIn, dEdgeIn);
160 dList.add(d);
161 }
162
163 public Dodecahedron findDodecahedron(String sLabelIn) {
164 Dodecahedron result = null;
165
166 for (int i = 0; i < dList.size(); i++) {
167 if (dList.get(i).getLabel().equalsIgnoreCase(sLabelIn)) {
168 result = dList.get(i);
169 break;
170 }
171 }
172 return result;
173 }
174
175 public Dodecahedron deleteDodecahedron(String sLabelIn) {
176 Dodecahedron result = findDodecahedron(sLabelIn);
177
178 if (result != null) {
179 dList.remove(result);
180 }
181 return result;
182 }
183
184 public boolean editDodecahedron(String sLIn, String sCIn, double dEIn) {
185 Dodecahedron result = null;
186
187 for (int i = 0; i < dList.size(); i++) {
188 if (dList.get(i).getLabel().equalsIgnoreCase(sLIn)) {
189 result = dList.get(i);
190 dList.get(i).setColor(sCIn);
191 dList.get(i).setEdge(dEIn);
192 return true;
193 }
194 }
195 return false;
196 }
197 }
198 public String summaryInfo() {
199 DecimalFormat df = new DecimalFormat("#,##0.0##");
200
201 String result = "----- Summary for " + getName()
202 + " -----"
203 + " Number of Dodecahedrons: "
204 + df.format(numberOfDodecahedrons())
205 + " Total Surface Area: "
206 + df.format(totalSurfaceArea())
207 + " Total Volume: "
208 + df.format(totalVolume())
209 + " Average Surface Area: "
210 + df.format(averageSurfaceArea())
211 + " Average Volume: "
212 + df.format(averageVolume())
213 + " Average Surface/Volume Ratio: "
214 + df.format(averageSurfaceToVolumeRatio());
215
216 return result;
217 }
218
DodecahedronListMenuApp.java
1 import java.io.IOException;
2 import java.util.Scanner;
3 import java.util.ArrayList;
4
5 /**
6 *
7 *
8 *
9 *
10 *
11 */
12
13 public class DodecahedronListMenuApp {
14 /**
15 *
16 * @param args not used
17 * @throws IOException in case a file is not found
18 */
19 public static void main(String[] args) throws IOException {
20
21 String listName = "*** no list name assigned ***";
22 ArrayList
23 DodecahedronList dodObjList = new DodecahedronList(listName, dodObj);
24 String fileName = "no file Name";
25
26 String userInput = "";
27 Scanner scanInput = new Scanner(System.in);
28
29 System.out.println("Dodecahedron List System Menu "
30 + "R - Read File and Create Dodecahedron List "
31 + "P - Print Dodecahedron List "
32 + "S - Print Summary "
33 + "A - Add Dodecahedron "
34 + "D - Delete Dodecahedron "
35 + "F - Find Dodecahedron "
36 + "E - Edit Dodecahedron "
37 + "Q - Quit");
38
39 do {
40 7System.out.print("Enter Code [R, P, S, A, D, F, E, or Q]: ");
41 5
42 7String label = "";
43 7String color = "";
44 7double edge = 0;
45 7int index;
46 5
47 7userInput = scanInput.nextLine();
48 7if (userInput.length() == 0) {
49 6continue;
50 5}
51 7userInput = userInput.toUpperCase();
52 7char userChar = userInput.charAt(0);
53 5
54 5
55 7switch (userChar) {
56 5case 'R':
57 56System.out.print("\tFile name: ");
58 56fileName = scanInput.nextLine();
59 56
60 56dodObjList = dodObjList.readFile(fileName);
61 56System.out.println("\tFile read in and Dodecahedron "
62 56 + "List created ");
63 56
64 56break;
65 56
66 5case 'P':
67 56System.out.println(dodObjList.toString());
68 56break;
69 56
70 5case 'S':
71 56System.out.println(" " + dodObjList.summaryInfo() + " ");
72 56break;
73 56
74 5case 'A':
75 56System.out.print("\tLabel: ");
76 56label = scanInput.nextLine();
77 56System.out.print("\tColor: ");
78 56color = scanInput.nextLine();
79 56System.out.print("\tEdge: ");
80 56edge = Double.parseDouble(scanInput.nextLine());
81 56
82 56dodObjList.addDodecahedron(label, color, edge);
83 56
84 56System.out.println("\t*** Dodecahedron added *** ");
85 56break;
86 56
87 5case 'D':
88 56System.out.print("\tLabel: ");
89 56label = scanInput.nextLine();
90 56
91 56if (dodObjList.deleteDodecahedron(label) != null) {
92 566dodObjList.deleteDodecahedron(label);
93 566System.out.println("\t\"" + label + "\" deleted ");
94 56} else {
95 56System.out.println("\t\"" + label + "\" not found ");
96 56}
97 56break;
98 56
99 5case 'F':
100 56System.out.print("\tLabel: ");
101 56label = scanInput.nextLine();
102 56
103 56if (dodObjList.findDodecahedron(label) != null) {
104 566System.out.println(dodObjList.findDodecahedron(label)
105 566 .toString() + " ");
106 56} else {
107 56System.out.println("\t\"" + label + "\" not found ");
108 56}
109 56break;
110 56
111 5case 'E':
112 56System.out.print("\tLabel: ");
113 56label = scanInput.nextLine();
114 56System.out.print("\tColor: ");
115 56color = scanInput.nextLine();
116 56System.out.print("\tEdge: ");
117 56edge = Double.parseDouble(scanInput.nextLine());
118 56
119 56if (dodObjList.findDodecahedron(label) != null) {
120 566dodObjList.editDodecahedron(label, color, edge);
121 566System.out.println("\"" + label + "\" successfully edited ");
122 56} else {
123 56System.out.println("\"" + label + "\" not found ");
124 56}
125 56break;
126 56
127 5case 'Q': case 'q':
128 56break;
129 56
130 5default:
131 56System.out.println("\t*** invalid code *** ");
132 5}
133 } while (!userInput.equalsIgnoreCase("Q"));
134 }
135 }
136
Step by Step Solution
There are 3 Steps involved in it
Step: 1
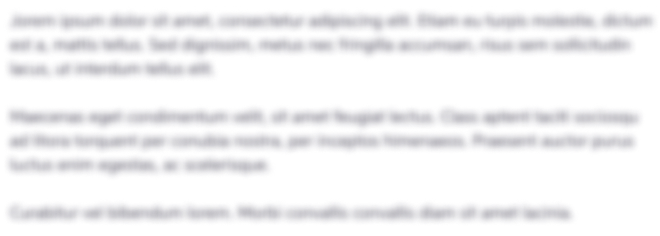
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started