Question
I need help adding an extra column on this code to be used for middle initials in java fxml. I can provide more code if
I need help adding an extra column on this code to be used for middle initials in java fxml. I can provide more code if necessary:
xmlns:fx="http://javafx.com/fxml">
Person.java
public class Person {
private final SimpleStringProperty firstName = new SimpleStringProperty("");
private final SimpleStringProperty middleInnitial = new SimpleStringProperty("");
private final SimpleStringProperty lastName = new SimpleStringProperty("");
private final SimpleStringProperty email = new SimpleStringProperty("");
public Person() {
this("","","","");
}
public Person(String firstName, String middleInnitial, String lastName, String email) {
setFirstName(firstName);
setMiddleInnitial(middleInnitial);
setLastName(lastName);
setEmail(email);
}
public SimpleStringProperty firstNameProperty() {
return firstName;
}
public SimpleStringProperty middleInnitialProperty() {
return middleInnitial;
}
public SimpleStringProperty lastNameProperty() {
return lastName;
}
public SimpleStringProperty emailProperty() {
return email;
}
public void setFirstName(String fName) {
firstName.set(fName);
}
public void setMiddleInnitial(String mInnitial) {
middleInnitial.set(mInnitial);
}
public void setLastName(String lName) {
lastName.set(lName);
}
public void setEmail(String mail) {
email.set(mail);
}
}
FormattedTableCellFactory.java
public class FormattedTableCellFactory
implements Callback> {
private TextAlignment alignment;
private Format format;
public TextAlignment getAlignment() {
return alignment;
}
public void setAlignment(TextAlignment alignment) {
this.alignment = alignment;
}
public Format getFormat() {
return format;
}
public void setFormat(Format format) {
this.format = format;
}
@Override
@SuppressWarnings("unchecked")
public TableCell call(TableColumn p) {
TableCell cell = new TableCell() {
@Override
public void updateItem(Object item, boolean empty) {
if (item == getItem()) {
return;
}
super.updateItem((T) item, empty);
if (item == null) {
super.setText(null);
super.setGraphic(null);
} else if (format != null) {
super.setText(format.format(item));
} else if (item instanceof Node) {
super.setText(null);
super.setGraphic((Node) item);
} else {
super.setText(item.toString());
super.setGraphic(null);
}
}
};
cell.setTextAlignment(alignment);
switch (alignment) {
case CENTER:
cell.setAlignment(Pos.CENTER);
break;
case RIGHT:
cell.setAlignment(Pos.CENTER_RIGHT);
break;
default:
cell.setAlignment(Pos.CENTER_LEFT);
break;
}
return cell;
}
}
FXMLTableView.java
public class FXMLTableView extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
Pane myPane = (Pane)FXMLLoader.load(getClass().getResource("FXMLTableView.fxml"));
Scene myScene = new Scene(myPane);
primaryStage.setScene(myScene);
primaryStage.setTitle("FXML TableView Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
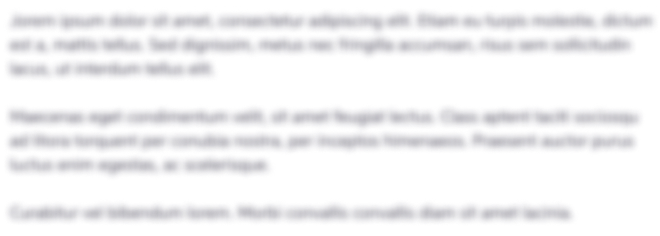
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started