Question
I Need Help Completing This Assignment my code is at the very top the assignment is at the bottom in the picturew I Am In
I Need Help Completing This Assignment my code is at the very top the assignment is at the bottom in the picturew I Am In Intro Algorithmic Design. My coding for part b is down below. My Tester is named the VehicleDetail.java For this part of your program, you will use linked lists and storage for your storage system.
Vehicle.java
public class Vehicle { protected String name; // The model name of the vehicle protected int number_of_wheels; // Stores the number of wheels the Vehicle has protected String make; // the make of the vehicle protected int mpg; // mileage per gallon // Parameterized Constructor public Vehicle(String name, int number_of_wheels, String make, int mpg) { super(); this.name = name; this.number_of_wheels = number_of_wheels; this.make = make; this.mpg = mpg; } public Vehicle(String name2, String make2, int mpg2, int numberwheels) { // TODO Auto-generated constructor stub } /** * * @return name - The Name of the Vehicle * */ public String getName() { return name; } /** * * @param name - Sets the Vehicle Name * */ public void setName(String name) { this.name = name; } /** * * @return number_of_wheels - No. of wheels the Vehicle has * */ public int getNumber_of_wheels() { return number_of_wheels; } /** * * @param number_of_wheels - Sets the No. of wheels for the Vehicle * */ public void setNumber_of_wheels(int number_of_wheels) { this.number_of_wheels = number_of_wheels; } /** * * @return make - The company name who made the Vehicle * */ public String getMake() { return make; } /** * * @param make - Sets the company Name who made the Vehicle * */ public void setMake(String make) { this.make = make; } public int getMpg() { return mpg; } public void setMpg(int mpg) { this.mpg = mpg; } // Display all the Vehicle Attributes @Override public String toString() { return "Vehicle name = " + name + " Number of wheels = " + number_of_wheels + " Make = " + make + " Mpg = " + mpg + ""; } } -------------------------------------------------------------------------------------------------------------------------------------------------------
Truck.java
public class Truck extends Vehicle { private int bedsize; // Only thing that makes Truck different to Vehicle /* Parent: String name, int number_of_wheels, String make, String type, int mpg Purpose of children constructors: */ public Truck(String name, int numwheels, String make, int mpg, int bedsize){ super(name, numwheels, make, mpg); this.bedsize = bedsize; } @Override
public String toString() { return super.toString() + " Bedsize = " + this.bedsize; } }
--------------------------------------------------------------------------------------------------------------------------------------------------------
Plane.java
public class Plane extends Vehicle { private int numWings ; // Only thing that makes Plane different to Vehicle //Constructor public Plane(String name, int numwheels, String make, int mpg, int numWings){ super(name, numwheels, make,mpg); this.numWings = numWings ; }
@Override
public String toString() {
return super.toString() + " Wings = " + this.numWings; } }
---------------------------------------------------------------------------------------------------------------------------------------------------------
Boat.java
public class Boat extends Vehicle { private String motortype; // Only thing that makes Boat different to Vehicle public Boat(String name, int numwheels, String make, int mpg, String motortype){ super(name, numwheels, make,mpg); this.motortype = motortype; } @Override
public String toString() { return super.toString() + " Motortype = " + this.motortype; } }
---------------------------------------------------------------------------------------------------------------------------------------------------------------
Car.java
public class Car extends Vehicle { private int numDoors ; // Only thing that makes Car different to Vehicle //Constructor public Car(String name, int numwheels, String make, int mpg, int numDoors){ super(name, numwheels, make,mpg); this.numDoors = numDoors ; }
@Override
public String toString() {
return super.toString() + " Number of Doors = " + this.numDoors; } }
---------------------------------------------------------------------------------------------------------------------------------------------------------------------
VehicleDetail.java
import java.util.ArrayList;
import java.util.Scanner;
public class VehicleDetails {
public static void main(String[] args) {
// TODO Auto-generated method stub
// copy the array that the adminstrator so that user has acces or have an array that both can have access to
ArrayList list = new ArrayList();
/*
* Algorithm for interactive console based program: prompt get input : y want
* to run while input : n get input op : 1 to administrator, 2 regular user if
* input : op 1 prompt '1 truck, 2 plane, ..' Truck t1 = new Truck("VOLVO SUV",
* 4, "VOLVO", "Pickup", 60, 1000);
*
* promt '1 to add, 2 remove' vehicleList.add(t1); else do-work regular user get
* input : prevent infinite loop
*
*/
int playAgain = 1; {// 1 is yes,
while (playAgain == 1) {
// admin or regular
System.out.println("Choose between the following options?");
System.out.println("1 Administrator Or 2 Regular Customer");
Scanner input = new Scanner(System.in);
int op = input.nextInt();
if (op == 1) {
// Admin
System.out.println("Which option would you like to do?");
System.out.println("Can only view vehicle details from name choosen in add vehicle");// can only view if you add a vehicle
System.out.println("1 Add A Vehicle, 2 Remove A Vehicle, 3 View Car Detail, 4 Switch Users");
op = input.nextInt();
while(op != 4) {
if (op == 1) {
int addVehicle = 1;//1 is Add Vehicle
System.out.println("Choose one of the following numbers");
System.out.println("1 Add Truck");
System.out.println("2 Add Car");
System.out.println("3 Add Boat");
System.out.println("4 Add Plane");
int type = input.nextInt();
System.out.println("What is the name?");
String name = input.next();
System.out.println("How many wheels?");
int numwheels = input.nextInt();
System.out.println("What is the make?");
String make = input.next();
System.out.println("What is the mpg?");
int mpg = input.nextInt();
if (type == 1) {
System.out.println("What is the bedsize?");
int bedsize = input.nextInt();
list.add(new Truck(name, numwheels, make, mpg, bedsize));
}
else if (type == 2) {
System.out.println("How many doors does it have?");
int numDoors = input.nextInt();
list.add(new Car(name, numwheels, make, mpg, numDoors));
}
else if (type == 3) {
System.out.println("What is the motortype?");
String motortype = input.next();
list.add(new Boat(name, numwheels, make, mpg, motortype));
}
else if (type == 4) {
System.out.println("How many wings does it have?");
int numWings = input.nextInt();
list.add(new Plane(name, numwheels, make, mpg, numWings));
}
}
else if (op == 2) {
int removeCar = 2;//2 is Remove Vehicle
// Remove Car
System.out.println("Please enter vehicle you want to remove?");// Remove Vehicle
System.out.println("Type in vehicle you want to remove Truck, Car, Boat, or Plane");
String select = input.next();
for (int i = 0; i
if (list.get(i).getName() == select ) {
list.remove(i);
}
}
}
else if (op == 3) {
int viewDetail = 3;//3 View Car Details
System.out.println("Please enter which vehicle name details you want to view?");
System.out.println("Type in name of vehicle you chose in add vehicle");
String select = input.next();
for (int i = 0; i
String getN = list.get(i).getName();
if (getN.equalsIgnoreCase(select)) {
System.out.println(list.get(i));
}
}
}
System.out.println("Which option would you like to do?");
System.out.println("Can only view vehicle details from name choosen in add vehicle");
System.out.println("1 Add A Vehicle, 2 Remove A Vehicle, 3 View Car Detail, 4 Switch User");
op = input.nextInt();
}
}
// regular
else if (op == 2) {
if(list.isEmpty()==true){
System.out.println("There are no cars added to view administrator must add cars first");
}
else {
int viewDetail = 3;//3 View Car Details
System.out.println("Please enter which vehicle details you want to view?");
System.out.println("Type in name of vehicle the administrator added");
String select = input.next();
for (int i = 0; i
if (list.get(i).getName().equalsIgnoreCase(select)) {
System.out.println(list.get(i));
}
}
System.out.println("Do you want to run the program again?");
System.out.println("1 for yes or 2 for no");
playAgain = input.nextInt(); //
{
{
}
}
}
}
}
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
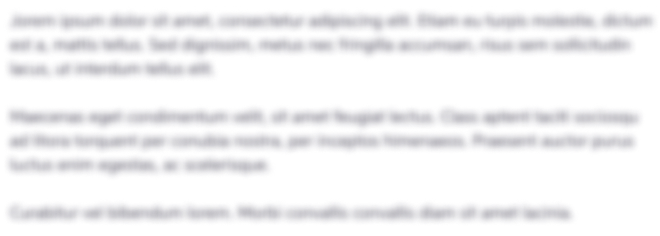
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started