Question
I need help editing my Code for a game i am making using JAVA . I made this game where there is a board of
I need help editing my Code for a game i am making using JAVA. I made this game where there is a board of 10x10 buttons 100 total and it generates 20 being a $$$ which is a treasure under and the rest a O which is a miss and you have 50 tries to find all the treasure. But i need to implement more to my game. To help you understand my code that i have made i will first put down my questions i was given to make the code in the first place first then under i will put the new questions that need to be implemented into my code. PLEASe help and please put Comments everywhere you can so i can understand what you are changing to the fullest extent please so i can learn from you thank you so much.
//////////////////////////////////////////////////////////////////////////////
Done already in the code below questions: FIRST questions i was asked already and what I already made into my code already
so when implementing more dont use this as reference to that but for reference
to know how i made my code already vvvvvvvvvvvvvvvvvvvvv
///////////////////////////////////////////////////////////////
Week 1
Game Features
Create UI similar to the illustration
Use fonts, color, images etc. to make the game look interesting
The treasure field is 10 by 10 locationsEach location can be clicked to reveal
A treasure, or
Nothing
Treasures Left shows the number of treasures left on the board starting with 20
Treasures Found shows the number of treasures the user has found
Tries Left shows the number of clicks on the board the user has left
Counting each field only once
The game starts with 50 tries available
Last Move shows whether the last click on the board revealed a treasure, a miss, or if the game is over.
User Stories
1.As a user, I can see the title of game so that I know what I will be playing.
2.As a player, I can see a board of 10 by 10 locations where I can click, so that I can play the game.
3.As a player, when I click on a location on the board where there is a treasure, the Treasures Left is reduce by one, and the Treasures Found is increased by one , so that I can see how successful I have been so far.
4.As a player, when I click on a location on the board, the location changes so that I can see if there was a treasure randomly placed at the location.
5.As a player, I can see how many treasures that are hidden on the board, so that I know how far away from winning I am.
6.As a player, I can see how many treasure that I have discovered so far, so that I can see how successful I have been.
7.As a player, I can see how many of the 20 tries/turns I started with are left, so that I can see how far I have to go.
8.As a player, when I click on a location on the board, the Tries Left is reduced by one, so that I know how many tries I have left .
9.As a player, when I click on a location that I have already clicked, nothing happens, so that I cannot fake finding treasures.
10.As a player, when I click on a location, the Last Move fields shows: "Miss" - if there was nothing at the location "Treasure" - if there was a treasure at the location "Game Over - You win" - if there are no treasures left "Game Over - You lose" - if there are treasures more left but no tries
11.As a player, when I have no more tries left, clicking on the board locations does nothing.
12.As a player, when I have no more tries left, I can see where all the treasures are on the board.
Technical Implementation
Implement each story one at a time in the order given.
Keep each class in a separate file. Use the CRC card format from the Chapter 8 (p. 547-548) to explain in a comment right before the class definition what the responsibilities and functions of the class are.
Use a border layout for the main window (or another layout that allows the design above).
Use a grid layout for the board and two nested for-loop to populate the board.
Extend the JButton class to create a BoardButton class instances of which are the contents of the board
Set the default text or icon for an unclicked button in the constructor
Make the button disabled when it is clicked.
Extend the JButton class to create a TreasureButton class instances of which are on the board
Change the look of the button when a user clicks on it: change the text or the icon
As you create the buttons, keep them in an array so that you can easily access them later.
The main method should simply contain: new TreasureGame().play();
Keep the code tidy (with comments and indentation) because you will be building on it over the next weeks.
////////////////////////////////////////////////////////
Next will be the new Questions i was given To implement into my code to be used when you make youre changes please leave comments throughout your changes please and thank you vvvvvvvvvvvvv
////////////////////////////////////////////////////
Week 2
New Game Features
The board now also contains 20 mines
20 distinct mines (not on top of each other or a treasure)
Mines have to be at least one location away from a treasure
User Stories
1.As a player, when I click on the board, in addition to a treasure or nothing, I may encounter a mine at random positions.
2.As a player, when I click on the board and encounter a mine, the mine is always one position in away from a treasure in the horizontal and vertical directions.
3.As a player, when I click on a mine, the game ends with Last Move field showing "Game Over - You lose".
4.As a player, after I click on a mine, clicking on the board locations does nothing.
5.As a player, when the game is over, I can see where all the treasures and mines are on the board.
Technical Implementation
Implement each story one at a time in the order given.
Keep each class in a separate file. Use the CRC card format from the Chapter 8 (p. 547-548) to explain in a comment right before the class definition what the responsibilities and functions of the class are. Be sure to have a Responsibilities and a Collaborating Classes sections.
Extend the BoardButton or JButton class, depending on what you did in the first week, to create a MineButton class instances of which are on the board
Change the look of the button when a user clicks on it: change the text or the icon
///////////////////////////////////////////
Now here i will put the code under this each new class starts at the comment that states a .java in it
/////////////////////////////////////////
// BoardButton.java
import java.awt.Dimension;
import javax.swing.JButton;
import javax.swing.SwingConstants;
public class BoardButton extends JButton { // Instance variables
private String displayText; //the displayText variable is used to hold the strings text
/**
* Default Constructor
*/
public BoardButton(TreasureGame game) //creates the miss button for the board buttons with this specific text string {
this("O");
this.addActionListener(new ButtonListener(game));
}
/**
* Parameterized Constructor
*/
public BoardButton(String text) { super();
// Set text
this.displayText = text;
// Set text alignment
setHorizontalAlignment(SwingConstants.CENTER);
// Set size
setPreferredSize(new Dimension(60, 40));
}
/**
* @return the text
*/
public String getDisplayText() {
return displayText; //returns the display text for what button you clicked on
} }
//////////////////////////////////
//TreasureButton.java
public class TreasureButton extends BoardButton
{ /**
* Constructor
*/
public TreasureButton(TreasureGame game) //creates the treasure button for the board buttons with this specific text { super("$$$");
this.addActionListener(new TreasureButtonListener(game));
} }
////////////////////////////////////////
//TreasureGame.java
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.SwingConstants;
public class TreasureGame extends JFrame { // Number of tries
protected static final int NUM_TRIES = 50;
// Number of treasures
protected static final int NUM_TREASURES = 20;
// Last move
private static final String lastMove = "Last move: %s";
// Instance variables
private JLabel lastMoveLbl; //Creates a JLabel instance with the specified text and horizontal alignment.
private StatsPanel statsPanel; //creates the statsPanel
private TreasureBoardPanel boardPanel; //creates the TreasureBoardPanel
private boolean gameOver; //holds the game over variable whether the game is done or not, so the game over text can show
/** * Constructor */ public TreasureGame() { super("Treasure Hunt Game");
// Set layout
setLayout(new BorderLayout());
// Set default close operation
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Show frame
setVisible(true); } /** * Starts the game */ public void play() { gameOver = false; // Add components addComponents();
// Resize frame to the components
pack(); } /** * Adds all the required panels to the frame */ private void addComponents() { // Create game header label
JLabel headerLbl = new JLabel("Treasure Hunt");
headerLbl.setPreferredSize(new Dimension(60, 40));
headerLbl.setHorizontalAlignment(SwingConstants.CENTER);
// Add label
add(headerLbl, BorderLayout.NORTH);
// Add stats panel
statsPanel = new StatsPanel();
add(statsPanel, BorderLayout.WEST);
// Add board panel
boardPanel = new TreasureBoardPanel(this);
add(boardPanel, BorderLayout.CENTER);
// Add last move label
lastMoveLbl = new JLabel(String.format(lastMove, ""));
lastMoveLbl.setPreferredSize(new Dimension(60, 40));
lastMoveLbl.setHorizontalAlignment(SwingConstants.CENTER);
add(lastMoveLbl, BorderLayout.SOUTH); } /** * Updates UI based on the move */ public void updateTreasureFound(boolean treasureFound) { // Update stats panel
statsPanel.updateTreasureFound(treasureFound);
// Update last move
String move = "";
if (statsPanel.getTreasuresLeft() == 0) { move = String.format(lastMove, "Game over - You win");
gameOver = true; //sets the game to over when all treasures are found and you win the game } else if (statsPanel.getTriesLeft() == 0) { move = String.format(lastMove, "Game over - You lose");
gameOver = true; /*sets the game to over when all tries are used and this is for when all treasure is not found and you lost the game */
} else { if (treasureFound)
move = String.format(lastMove, "Treasure"); //display this text when treasure is found
else
move = String.format(lastMove, "Miss"); //display this text when you do not find a treasure } // Set last move label
lastMoveLbl.setText(move);
// If game is over show all button text
if (gameOver&& statsPanel.getTreasuresLeft() != 0)
boardPanel.displayAllTreasures(); //shows all the treasure spots when the game is over } /** * @return the gameOver */ public boolean isGameOver() { return gameOver; //returns the boolean true or false for game over every turn to check if you are done or not } }
///////////////////////////////////
// TreasureBoardPanel.java
import java.awt.GridLayout;
import java.util.Random;
import javax.swing.JPanel;
public class TreasureBoardPanel extends JPanel {
private static final int WIDTH = 10;
private static final int HEIGHT = 10;
// Instance variables
private BoardButton[] button; //variable used to add buttons to the panel
private TreasureGame game; //used to make the constructor for the TreasureBoardPanel
/**
* Constructor
*/
public TreasureBoardPanel(TreasureGame game) {
super();
this.game = game;
// Set layout
setLayout(new GridLayout(WIDTH, HEIGHT));
// Add buttons
addButtons();
}
/**
* Creates and adds buttons to the panel
*/
private void addButtons() {
this.button = new BoardButton[WIDTH * HEIGHT];
// Create random treasure buttons
Random r = new Random();
for (int i = 0; i < TreasureGame.NUM_TREASURES; i++) {
int index = r.nextInt(WIDTH * HEIGHT);
// Check if index is already a button
while (this.button[index] != null)
index = r.nextInt(WIDTH * HEIGHT);
this.button[index] = new TreasureButton(game);
}
// For all the buttons which are not TreasureButton i.e. null buttons
// create BoardButton
for (int i = 0; i < this.button.length; i++) { if (this.button[i] == null) { this.button[i] = new BoardButton(game); }
add(this.button[i]); //adds this button
} } /**
* Displays text on all treasure buttons
*/ public void displayAllTreasures() { for (int i = 0; i < this.button.length; i++) {
this.button[i].setText(this.button[i].getDisplayText()); } } }
///////////////////////////////////////////
//TreasureGUI.java
public class TreasureGameGUI { public static void main(String[] args) //main class used to play the game { new TreasureGame().play(); } }
/////////////////////////////////////
//StatsPanel.java
import java.awt.Dimension;
import javax.swing.Box;
import javax.swing.BoxLayout;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class StatsPanel extends JPanel { private static final String treasuresLeftStr = "Treasures left: %d";
private static final String treasuresFoundStr = "Treasures found: %d";
private static final String triesLeftStr = "Tries left: %d";
// Instance variables
//used to hold the variable of how much treasure is left
private int treasuresLeft; //holds how much treasure is found by the user when playing private int treasuresFound; //holds how many tries left private int triesLeft;
private JLabel treasuresLeftLbl; //Creates a JLabel instance with the specified text and horizontal alignment.
private JLabel treasuresFoundLbl; //Creates a JLabel instance with the specified text and horizontal alignment.
private JLabel triesLeftLbl; //Creates a JLabel instance with the specified text and horizontal alignment.
/**
* Constructor
*/
public StatsPanel() { super();
// Set size
setPreferredSize(new Dimension(200, 100));
// Set layout
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
// Set defaults
treasuresLeft = TreasureGame.NUM_TREASURES;
treasuresFound = 0; //sets default of amount of treasures left to zero because thats what you start at
triesLeft = TreasureGame.NUM_TRIES;
// Create labels
treasuresLeftLbl = new JLabel(
String.format(treasuresLeftStr, treasuresLeft)); //string label created on the left side of the game
treasuresFoundLbl = new JLabel(
String.format(treasuresFoundStr, treasuresFound)); //string label created on the left side of the game
triesLeftLbl = new JLabel(String.format(triesLeftStr, triesLeft)); //string label created on the left
// Add labels
add(Box.createVerticalGlue());
add(treasuresLeftLbl); //adding the label to the game
add(treasuresFoundLbl); //adding the labels to the game
add(triesLeftLbl); //adding the labels to the game
add(Box.createVerticalGlue()); } /** * Increases/Decreases treasure found by 1 based on treasureFound */ public void updateTreasureFound(boolean treasureFound) { if (treasureFound) { treasuresFound += 1; //adds an integer to the amount of treasure found if one is found
treasuresFoundLbl
.setText(String.format(treasuresFoundStr, treasuresFound)); //uses this label tp add it to
treasuresLeft -= 1; //subtracts one integer number from how many treasures are left when one is found
treasuresLeftLbl .setText(String.format(treasuresLeftStr, treasuresLeft)); //subtracts it from this label } // Update tries updateTriesLeft(); } /** * Decreases tries left by 1 */ private void updateTriesLeft() { triesLeft -= 1; //decreases the amount of tries left based on how many tries are used by the player
triesLeftLbl.setText(String.format(triesLeftStr, triesLeft)); //uses this label triesLeft } /** * @return the treasuresLeft */ public int getTreasuresLeft() { return treasuresLeft; //returns amount of treasures left } /** * @return the triesLeft */ public int getTriesLeft() { return triesLeft; //returns amount of tries left } }
////////////////////////////////////////////
// TreasureButtonListener.java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TreasureButtonListener implements ActionListener { // Instance variables private TreasureGame game; //private variable used to make the action listener for the Treasure button listener class /** * Constructor */ public TreasureButtonListener(TreasureGame game) { this.game = game; } /** * Handles the button click event */ @Override public void actionPerformed(ActionEvent e) { // Check if game is not over if (!game.isGameOver()) { // Get source
BoardButton btn = (BoardButton) e.getSource();
// Set text
btn.setText(btn.getDisplayText());
game.updateTreasureFound(true);
// Set disabled
btn.setEnabled(false); } } }
/////////////////////////////////////
// ButtonListener.java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ButtonListener implements ActionListener { // Instance variables private TreasureGame game; /*private variable for this class so it can be repeated here it is used to create the action listener for the button listener class*/ /** * Constructor */ public ButtonListener(TreasureGame game) { this.game = game; } /** * Handles the button click event */ @Override public void actionPerformed(ActionEvent e) { // Check if game is not over if (!game.isGameOver()) { // Get source
BoardButton btn = (BoardButton) e.getSource();
// Set text
btn.setText(btn.getDisplayText());
game.updateTreasureFound(false);
// Set disabled
btn.setEnabled(false); } } }
////////////////////////////////////////////////
The output is this. I sent it through URL because my chegg for some reason wont let me post the picture.
https://cdn.discordapp.com/attachments/285590814934171649/388223710907072512/TreasureHuntGame_Edited.png
Step by Step Solution
There are 3 Steps involved in it
Step: 1
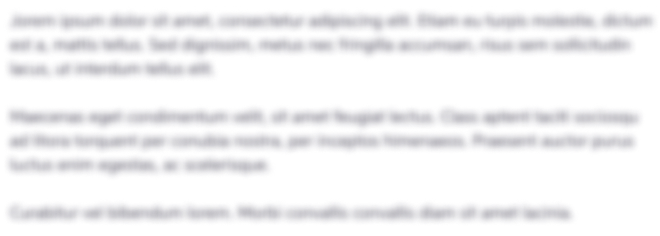
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started