Question
I need help figuring out what is wrong with my code it goes into an infinite loop. Thank you! Here's the input of the test
I need help figuring out what is wrong with my code it goes into an infinite loop. Thank you!
Here's the input of the test case.
1 236.330 237.844 | |
2 | y |
3 | 237.862 237.424 |
4 | y |
5 | -237.424 238.323 |
6 | 237.424 238.323 |
7 | y |
8 | 238.323 238.855 |
9 | y |
10 | 238.855 -237.645 |
11 | 238.855 237.645 |
12 | y |
13 | 237.645 0 |
14 | 237.645 238.321 |
15 | y |
16 | 238.321 239.488 |
17 | y |
18 | 239.488 238.654 |
19 | y |
20 | 238.654 239.533 |
21 | y |
22 | 239.533 239.755 |
23 | y |
24 | 239.755 238.988 |
25 | y |
26 | 238.988 239.444 |
27 | y |
28 | 239.444 239.110 |
29 | y |
30 | 239.110 240.030 |
31 | y |
32 | 240.030 239.678 |
33 | y |
34 | 239.678 240.096 |
35 | y |
36 | 240.986 240.320 |
37 | y |
38 | 240.320 240.789 |
39 | y |
40 | 240.789 240.123 |
41 | y |
42 | 240.123 239.566 |
43 | y |
Expected Output:
Enter the old and new consumer price indices: Inflation rate is 0.640626 2 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is -0.184142 3 Try again? (y or Y): Enter the old and new consumer price indices: Error: CPI values must be greater than 0 4 Enter the old and new consumer price indices: Inflation rate is 0.378648 5 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is 0.223225 6 Try again? (y or Y): Enter the old and new consumer price indices: Error: CPI values must be greater than 0 7 Enter the old and new consumer price indices: Inflation rate is -0.50658 8 Try again? (y or Y): Enter the old and new consumer price indices: Error: CPI values must be greater than 0 9 Enter the old and new consumer price indices: Inflation rate is 0.284456 10 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is 0.489679 11 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is -0.348243 12 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is 0.368315 13 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is 0.0926804 14 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is -0.319909 15 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is 0.190802 16 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is -0.13949 17 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is 0.384759 18 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is -0.14665 19 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is 0.1744 20 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is -0.276358 21 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is 0.195154 22 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is -0.276591 23 Try again? (y or Y): Enter the old and new consumer price indices: Inflation rate is -0.231967 24 Try again? (y or Y): Average rate is 0.0496408 25 Median rate is 0.13354
Here's my code:
#include
// Add a function to get the CPI values from the user and validate that they are greater than 0. void getCPIValues(float &old_cpi, float &new_cpi){ do{ cout << "Enter the old and new consumer price indices: ";
cin >> old_cpi >> new_cpi;
if (!(old_cpi > 0 && new_cpi > 0))
{
cout << "Error: CPI values must be greater than 0 ";
}
} while (!(old_cpi > 0 && new_cpi > 0));
}
//Function that takes two double parameters and swap //their values void swap_values(double *ay, double *yx) { double temp = *ay; *ay = *yx; *yx = temp; }
//Function to sort the array //Bubble Sort void sort_array(double arr[], int number) { int i, j; for (i = 0; i < number-1; i++) // Last i elements are already in place for (j = 0; j < number-i-1; j++) if (arr[j] > arr[j+1]) // Calling swap_values function to // swap elements of array swap_values(&arr[j], &arr[j+1]); }
// Declare and implement a function called swap_values that takes two double parameters like the one we defined in class. // Declare and implement a function that uses either a selection sort or bubble sort to put the array values into ascending order (i.e. smallest to largest). // Function parameters: an array of doubles and an int with the number of elements in the arra
// + Your sort function must use the swap_values function defined above to exchange the values in the array during the sort.
// + You can use either a selection sort or a bubble sort in the sort_array function but it must use the swap_values function.
// double findMedianRate(double arr[], int number){ sort_array(arr, number); if(number % 2!=0){ return (double)arr[number/2]; } else{ return (double)( arr[(number-1)/2] + arr[number/2] ) / 2.0; } }
// if the number of rates is even, then the median rate is calculated as the average of the two rates in the middle. double average_median_rate(double rates[], int number) { double sum = 0; for (int i=0; i //main function int main(){ // Declare a constant called MAX_RATES and set it to 20. const int MAX_RATES = 20; // array of type double to store inflation rates double double_inflationRates[MAX_RATES]; // Add code to main that inserts the computed inflation rate into the next position in the array. int inserts = 0; char ch='Y'; double inflationRate = 0.00; float old_cpi, new_cpi; // while user chooses 'y' or 'Y' while(ch=='Y' or ch=='y'){ // input CPI values getCPIValues(old_cpi, new_cpi); // calculate inflation // inflation = ((currentCPI - oldCPI)/oldCPI) * 100 inflationRate = (double)( ( new_cpi - old_cpi ) / old_cpi ) * 100; // to avoid overflow of array if(inserts < MAX_RATES){ // insert inflation in the array double_inflationRates[inserts++] = inflationRate; } // print the inflation rate cout<<"Inflation rate is "< return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
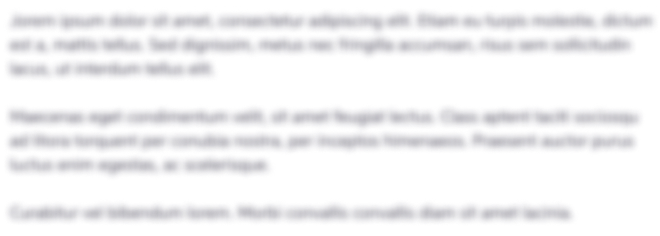
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started