Question
I need help fixing my code, which is given at the bottom. I am trying to create a class for a priority (maximum) heap. I
I need help fixing my code, which is given at the bottom. I am trying to create a class for a priority (maximum) heap. I was given all of the methods except for the add() method and the removeMax() method. The add() method is supposed to add a value while the removeMax() method is supposed to remove the root value. You can make trivial changes, but please do not deviate from the core algorithm. In the main() method, I used add() to add the following values in order: 1 3 2 4 6 7 8 5. Then I performed removeMax() 6 times. The final tree should only have 2 and 1, but mine has 3 and 2.
My code:
public class Heap { private int[] data = new int[10]; // Heap data private int manyItems = 0; // Quantity of items in the heap public static void swap(int n1, int n2) { int temp = n1; n1 = n2; n2 = temp; } private void fixHeap() { int position = 0; int childPos; while (position * 2 + 1 < manyItems) { childPos = position * 2 + 1; if (childPos < manyItems - 1 && data[childPos + 1] > data[childPos]) childPos++; if (data[position] >= data[childPos]) return; swap(position, childPos); position = childPos; } } public int removeMax() { if (manyItems == 0) throw new RuntimeException(); int maxValue = data[0]; // Get root value data[0] = data[manyItems - 1]; manyItems--; fixHeap(); return maxValue; } public void add(int element) { int position; if (manyItems == data.length) ensureCapacity((manyItems + 1) * 2); data[manyItems] = element; manyItems++; position = manyItems - 1; while (position > 0 && data[position] > data[(position - 1) / 2]) { swap(position, (position - 1) / 2); position = (position - 1) / 2; } } // Print all heap values with array indexes public void print() { for (int i = 0; i < manyItems; i++) System.out.println("[" + i + "] " + data[i]); } public void ensureCapacity(int minimumCapacity) { int[] biggerArray; if (data.length < minimumCapacity) { biggerArray = new int[minimumCapacity]; System.arraycopy(data, 0, biggerArray, 0, manyItems); data = biggerArray; } } public static void main(String[] args) { Heap h = new Heap(); System.out.println("Adding values..."); h.add(1); h.add(3); h.add(2); h.add(4); h.add(6); h.add(7); h.add(8); h.add(5); h.print(); System.out.println("Removing... "); h.removeMax(); h.removeMax(); h.removeMax(); h.removeMax(); h.removeMax(); h.removeMax(); h.print(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
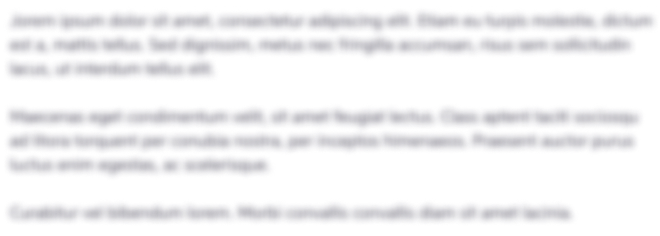
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started