Question
I need help fixing the following program: Descriptions for Project #4: Modify your project #3 by implementing the following: Create two and three-dimensional arrays to
I need help fixing the following program:
Descriptions for Project #4: Modify your project #3 by implementing the following:
Create two and three-dimensional arrays to store all input and output data and fill these arrays by the data read from the input file and by the computed data. The data of all employees will be stored in these arrays before writing it to the output file.
As you know, all elements of an array must be of same type. Therefore, you will be creating different arrays to store different types of data. For an example you could have the following array declarations:
A two-dimensional array NonNumeric of type string to store headings, Employee name, and supervisors name. Employee ID, Employee Telephone, Employee Address, warning/appreciation letter, and Note for n employees. For three employees it may look like NonNumeric1 [3] [8]
A three-dimensional array Numeric1 of type double to store three years and three semesters input data read from the input file for n employees. For three employees it may look like Numeric1 [3] [3] [3]
A two-dimensional array Numeric2 of type double to store Final Weighted Evaluation, 2013, Final Weighted Evaluation, 2014, Final Weighted Evaluation, 2015, Total Final Weighted Evaluation, Average Final Weighted Evaluation, Current Salary, Salary Raise in %, Salary Raise in Dollars, Salary in dollars with the raise. For three employees it may look like Numeric2 [3] [9].
At the end when all the input and output data has been stored in arrays, you will generate all reports and write them to the output file.
Of course, you will need nested loops in the program.
You must use named constants to declare the sizes of arrays.
In addition to input validation, you should also validate if the file opened correctly.
In your loops, you should also check that arrays do not get out of bound
Program:
/* Mitchell Malfitano Id #: mam861 Project #2 Evaluation Report/Raise Last Modified 2/20/2018 */ #include #include #include #include
using namespace std;
int main() {
ifstream inFile; inFile.open ( "Project4_mam861_Input.txt" ); if ( !inFile ) { cout << "Unable to open file." << endl << endl;
system ("PAUSE>NUL");
return -1; }
ofstream fout; fout.open ( "Project4_mam861_Output.txt" );
if ( !fout ) { cout << endl << endl << "Program Terminated." << endl << endl << "Unable to open file." << endl;
system ("PAUSE>NUL");
return -1; } int const SIZEI = 5, SIZEN = 10; string INFO [SIZEI], letter;
double const spring_eval = .37, summer_eval = .24, fall_eval = .39;
double NUM [SIZEN], Salary_Raise, Weighted_eval2015, Weighted_eval2016, Weighted_eval2017, total_weighted, Final_Weighted_Evaluation, total_raise, new_pay;
int i = 0, e = 0, n = 3, j ;
cout << "Number of employees being evaluated is 3! " << endl;
while (i < n ) { getline(inFile, INFO [0]); getline(inFile, INFO [1]); getline(inFile, INFO [2]); getline(inFile, INFO [3]); getline(inFile, INFO [4]); inFile.ignore(1,' ');
for (int e = 0; e < 1; e++ ) { inFile >> NUM [0] >> NUM [1] >> NUM [2] >> NUM [3] >> NUM [4] >> NUM [5] >> NUM [6] >> NUM [7] >> NUM [8] >> NUM [9]; //input checks for numbers and employee information //if ( for (j = 0; j <1; j++) {
fout << fixed << setprecision(2); fout << setw(60) << "Texas State University, San Marcos " << endl << setw(74) << "Annual Employee Evaluation Report From January 1, 2015 to December 31, 2017" << endl << endl << setw(39) << "Name of the Employee: " << setw(15) << INFO [0] << endl << setw(39) << "Name of the Supervisor: " << setw(15) << INFO [1] << endl << setw(39) << "Employee Id#: " << setw(16) << INFO [2] << endl << setw(39) << "Telephone Number: " << setw(16) << INFO [3] << endl << setw(39) << "Address: " << setw(38) << INFO [4] << endl << setw(38) << "Spring Semester Evaluation 2015:" << setw(9) << NUM [0] << endl << setw(38) << "Summer Semester Evaluation 2015:" << setw(9) << NUM [1] << endl << setw(38) << "Fall Semester Evaluation 2015:" << setw(9) << NUM [2] << endl << setw(38) << "Spring Semester Evaluation 2016:" << setw(9) << NUM [3] << endl << setw(38) << "Summer Semester Evaluation 2016:" << setw(9) << NUM [4] << endl << setw(38) << "Fall Semester Evaluation 2016:" << setw(9) << NUM [5] << endl << setw(38) << "Spring Semester Evaluation 2017:" << setw(9) << NUM [6] << endl << setw(38) << "Summer Semester Evaluation 2017:" << setw(9) << NUM [7] << endl << setw(38) << "Fall Semester Evaluation 2017:" << setw(9) << NUM [8] << endl;
Weighted_eval2015 = ( ( NUM [2] * fall_eval ) + ( NUM [0] * spring_eval ) + ( NUM [1] * summer_eval ) ); fout << setw(38) << "Final Weighted Evaluation 2015:" << setw(9) << Weighted_eval2015 << endl;
Weighted_eval2016 = ( ( NUM [5] * fall_eval ) + ( NUM [3] * spring_eval ) + ( NUM [4] * summer_eval ) ); fout << setw(38) << "Final Weighted Evaluation 2016:" << setw(9) << Weighted_eval2016 << endl;
Weighted_eval2017 = ( ( NUM [8] * fall_eval ) + ( NUM [6] * spring_eval ) + ( NUM [7] * summer_eval ) ); fout << setw(38) << "Final Weighted Evaluation 2017:" << setw(9) << Weighted_eval2017 << endl;
total_weighted = ( Weighted_eval2015 + Weighted_eval2016 + Weighted_eval2017); fout << setw(38) << "Total Final Weighted Evaluation:" << setw(10) << total_weighted << endl;
Final_Weighted_Evaluation = ( ( Weighted_eval2015 + Weighted_eval2016 + Weighted_eval2017) / 3 ); if (Final_Weighted_Evaluation < 70 ) { letter = "This is a Warning to assure you that you are in the Red zone and if you fail to improve termination may be a consequence"; } else if (Final_Weighted_Evaluation >= 95 ) letter = "Thank you, please keep up the outstanding performance"; else if (Final_Weighted_Evaluation) letter = "You are doing ok";
if (Final_Weighted_Evaluation < 70 ) Salary_Raise = 0;
if (Final_Weighted_Evaluation > 75 && Final_Weighted_Evaluation <= 80 ) Salary_Raise = 1;
if (Final_Weighted_Evaluation > 80 && Final_Weighted_Evaluation <= 90 ) Salary_Raise = 3;
if (Final_Weighted_Evaluation > 90 && Final_Weighted_Evaluation <= 100 ) Salary_Raise = 5;
if (Final_Weighted_Evaluation > 100 ) Salary_Raise = 10;
total_raise = ( Salary_Raise / 100 ) * NUM [9]; new_pay = total_raise + NUM [9]; fout << setw(38) << "Average Final Weighted Evaluation:" << setw(9) << Final_Weighted_Evaluation << endl << setw(39) << "Current Salary: " << setw(4) << "$" << NUM [9] << endl << setw(39) << "Salary Raise in %: " << setw(7) << Salary_Raise << endl << setw(39) << "Salary Raise in $: " << setw(10) << total_raise << endl << setw(39) << "Salary in dollars with the raise: " << setw(4) << "$" << new_pay << endl << endl << " " << letter << endl << " Note: This report for " << INFO [0] << " was prepared according to the fair practice of the University." << endl << " Any discrepancies must be reported by " << INFO [0] << " to their supervisor, " << INFO [1] << "." << endl << endl; } } i++; } inFile.close (); fout.close();
system ("PAUSE>NUL");
return 0;
}
Here is my input file
Nancy Price John Albert A-5971-359182 (512)354-9855 510 Albatross Dr., Austin, TX 78310 80.00 75.00 90.00 83.00 78.00 93.00 86.00 79.00 95.00 90000.00 Spartacus Gladiator Oenameous Doctorie A-1234-567890 (555)654-9871 511 Albatross Dr., Austin TX 78310 100.00 100.00 100.00 100.00 100.00 100.00 100.00 100.00 100.00 80000.00 Asher Gladiator Oenameous Doctorie A-1234-567889 (555)654-9870 509 Albatross Dr., Austin TX 78310 55.00 40.00 60.00 50.00 62.00 53.00 42.00 37.00 54.00 100000.00
Step by Step Solution
There are 3 Steps involved in it
Step: 1
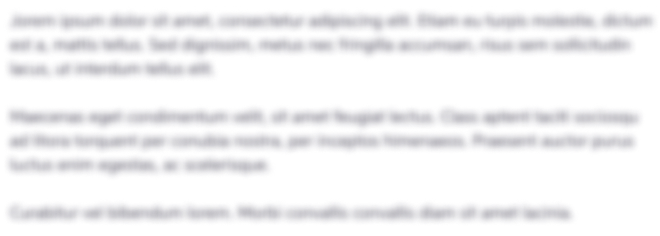
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started