Question
*** I need help identifying my incoming parameters, outgoing parameters, and return values in the C++ code below. There are 4 functions in the code
*** I need help identifying my incoming parameters, outgoing parameters, and return values in the C++ code below. There are 4 functions in the code below and the 3 fields for each function are bolded. Should one of the items not exist, put N/A. ****
#include #include using namespace std;
int input(long long); // function to Input of Base 2 value to enforce the entry of only 1's and 0's int input2(double); int Base10toBase2(long long); // Base 10 to Base 2 int Base2toBase10(long long); //Base 2 to Base 10
int main() { long long n, o; while (1) { cout << "Enter a positive integer in Base 10: "; double x; cin >> x; if (input2(x)) { n=x; break; } else { cout << "Entered number is not a positive integer. Please try again." << endl; } }
while (1) { cout << "Enter a binary number: "; cin >> o; if (input(o)) { break; }
else { cout << "Entered number is not a binary number. Please try again." << endl; } }
cout << endl; cout << n << " in decimal = " << Base10toBase2(n) << " in binary" << endl; cout << o << " in binary = " << Base2toBase10(o) << " in decimal"; return 0;
}
//*********************************************** // Function Name: Base10toBase2 // Incoming Parameters: // Outgoing Parameters: // Return Value: //*********************************************** int Base10toBase2(long long n) { long long BinaryNo = 0; int remainder, i = 1; while (n != 0) { remainder = n % 2; n /= 2; BinaryNo += remainder * i; i *= 10; }
return BinaryNo; }
//*********************************************** // Function Name: Base2toBase10 // Incoming Parameters: // Outgoing Parameters: // Return Value: //*********************************************** int Base2toBase10(long long o) { int DecimalNo = 0, i = 0, remainder; while (o != 0) { remainder = o % 10; o /= 10; DecimalNo += remainder * pow(2, i); ++i; }
return DecimalNo; }
//*********************************************** // Function Name: input - input of Base 2 value to enforce the entry of only 1s and 0s // Incoming Parameters: // Outgoing Parameters: // Return Value: //*********************************************** int input(long long num) { long long x; while (num) { x = num % 10; if ((x == 0) || (x == 1)) { num = num / 10; }
else { return 0; } } return 1; }
//*********************************************** // Function name: input2 - ensures the Base 10 number entered by the user is a positive integer // Incoming Parameters: // Outgoing Parameters: // Return Value: //*********************************************** int input2(double x){ if(x<0) return 0; if(1000*x!=(1000*int(x))) return 0; return 1; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
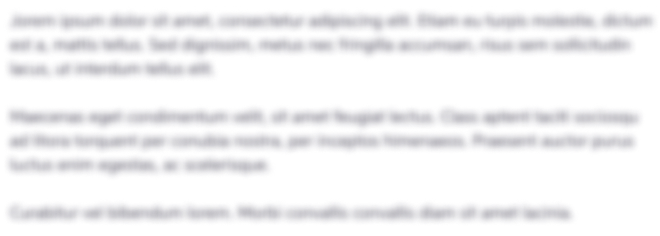
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started