Question
I need help implementing the following program using the guidelines and following the class.py and deck.py files included at the bottom. CARD.PY: Define the Card
I need help implementing the following program using the guidelines and following the class.py and deck.py files included at the bottom.
CARD.PY:
"""Define the Card class."""
class Card: """Model a playing card.
Rank is an int (1-13), where aces are 1 and kings are 13. Suit is an int (1-4), where clubs are 1 and spades are 4. Value is an int (1-10), where aces are 1 and face cards are 10. """
# List to map int rank to printable character (index 0 used for no rank) RANK = ["x", "A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"]
# List to map int suit to printable character (index 0 used for no suit) # 1 is clubs, 2 is diamonds, 3 is hearts, and 4 is spades SUIT = ["x", "\u2663", "\u2666", "\u2665", "\u2660"]
def __init__(self, rank: int = 0, suit: int = 0) -> None: """Initialize card to specified rank (1-13) and suit (1-4).
Args: rank (int, optional): The card's rank. Defaults to 0. suit (int, optional): The card's suit. Defaults to 0. """ self.__rank = 0 self.__suit = 0 self.__face_up = None
# Verify that rank and suit are integers and that they are within range # (1-13 and 1-4), then update instance variables if valid. if isinstance(rank, int) and isinstance(suit, int): # if type(rank) == int and type(suit) == int: if rank in range(1, 14) and suit in range(1, 5): self.__rank = rank self.__suit = suit self.__face_up = True
def __eq__(self, other) -> bool: """Compare two cards.
Args: other (Card): An instance of a Card.
Returns: Boolean: True, if both Cards are of equal rank; False otherwise. """ if not isinstance(other, Card): return False
return self.rank() == other.rank() and self.suit() == other.suit()
def __gt__(self, other) -> bool: """Compute if the rank of this card is greater than the rank of the other card.
Args: other (Card): An instance of a Card.
Returns: bool: True if the rank of this card is greater than other's rank. """ if not isinstance(other, Card): return False
return self.rank() > other.rank()
def __hash__(self) -> int: """Compute the hash of the object.
Returns: int: A unique value representing the card. """ return hash(self.__key())
def __key(self) -> tuple[int, int]: """Generate a unique key value for the card.
Returns: tuple[int, int]: A value that represents the card. """ return (self.__rank, self.__suit)
def __lt__(self, other) -> bool: """Compute if the rank of this card is less than the rank of the other card.
Args: other (Card): An instance of a Card.
Returns: bool: True if the rank of this card is less than other's rank. """ if not isinstance(other, Card): return False
return self.rank()
def __repr__(self) -> str: """Generate a string representation of the card.
The output of repr() is meant to aid debugging. Is should uniquely identify the object and it should be possible to instantiate a new object using the returned value.
Returns: str: A string from which the card could be instantiated. """ return f"Card(rank = {self.__rank}, suit = {self.__suit})"
def __str__(self) -> str: """Generate a string representation of the card.
The output of str() is meant to be human-readable.
Returns: str: A string representation of the card. """ # Use rank to index into rank_list; use suit to index into suit_list. if self.__face_up: return f"{self.RANK[self.__rank]}{(self.SUIT)[self.__suit]}"
return "XX"
def flip_card(self) -> None: """Flip card between face-up and face-down.""" self.__face_up = not self.__face_up
def is_face_up(self) -> bool | None: """Return True if card is facing up.
Returns: Boolean: True if the card is face up; False, otherwise. """ return self.__face_up
def rank(self) -> int: """Get the rank of the card.
The card's rank is an integer in the range 1 (Ace) to 13 (King).
Returns: int: The card's rank. """ return self.__rank
def suit(self) -> int: """Get the suit of the card.
The card's suit is an integer 1 (clubs), 2 (hearts), 3 (diamonds), or 4 (spades).
Returns: int: The card's suit. """ return self.__suit
def value(self) -> int: """Get the value of the card.
The card's value (1 for aces, 2-9, 10 for face cards).
Returns: int: The card's value. """ return self.__rank if self.__rank
DECK.py:
#!/usr/bin/env python3 """Define the Deck class.""" import random
from card import Card
class Deck: """Model a deck of 52 playing cards.
Implement the deck as a list of cards. The last card in the list is defined to be at the top of the deck. """
def __init__(self) -> None: """Initialize deck.
Ace of clubs on bottom, King of spades on top. """ self.__deck = [Card(r, s) for s in range(1, 5) for r in range(1, 14)]
def __len__(self) -> int: """Return number of cards remaining in deck.
Returns: int: The number of cards remaining in the deck. """ return len(self.__deck)
def __repr__(self) -> str: """Generate a string representation of the deck.
The output of repr() is meant to aid debugging. Is should uniquely identify the object.
Returns: str: A string from which the card could be instantiated. """ return str(self)
def __str__(self) -> str: """Generate a string representation of the deck.
Returns: str: A string representation of the deck. """ return ", ".join([str(card) for card in self.__deck])
def shuffle(self) -> None: """Shuffle the deck in place.""" random.shuffle(self.__deck)
def deal(self) -> Card: """Deal a card from the deck.
Return top card from deck; None, if the deck is empty.
Returns: Card: An instance of the Card class. """ return self.__deck.pop()
def display(self, cols: int = 13) -> None: """Display the entire deck.
Compute a column-oriented display of deck.
Args: cols (int, optional): A string representing the cards in the deck in order. Defaults to 13 columns. """ for index, card in enumerate(self.__deck): if not index % cols: print() print(f"{card!s:4}", end="") print(" " * 2)
def is_empty(self) -> bool: """Return True if the Deck is empty.
Returns: Boolean: True if the Deck is empty; False, otherwise. """ return len(self.__deck)
def pretty_print(self, column_max=10): """Column-oriented printing of a deck.""" for index, card in enumerate(self.__deck): if index % column_max == 0: print() print(f"{str(card):4s}", end="") print(" " * 2)
This assignment focuses on the design, implementation, and testing of a Python program that uses classes to solve the problem described below. You would be wise to familiarize yourself with the rules by actually playing the game. One website that allows you to play FreeCell online: http://games.aarp.org/games/freecell/freecell.aspx The general rules of the game are: - Start: - All 52 cards are dealt into eight columns (the tableau), where four of the columns contain seven cards each and four of the columns contain six cards each. - All of the cards are visible. - Objective: - The object of the game is to build up each foundation, in sequence, from Ace to King. - The player wins if they are able to move all 52 cards to the four foundations. - Only one card may be moved at a time. - Only the bottom card of each of the eight tableau columns and any cards in the free cells are eligible to be moved. - Once a card is moved onto a foundation pile, it cannot be moved again. - Any card may be moved from the tableau to an empty free cell (but each free cell may only hold one card at a time). - Any card may be moved from the tableau or the free cells to an empty column in the tableau. - The game is over when the player wins (by moving all 52 cards to the foundations), loses (can no longer make any moves), or resigns. Your program will permit the user to play FreeCell and will enforce the game rules. 1. Your program will use the card. py and card. py files provided with the assignment. You may not modify the contents of these files. 3. Your program must be subdivided into meaningful methods. 4. Your program will recognize the following menu of options: t2f T F -- move from Tableau T to Foundation F t2c T C -- move from Tableau T to Cell C t2t T1 T2 -- move from Tableau T1 to Tableau T2 c2t C T -- move from Cell C to Tableau T c2f C F -- move from Cell C to Foundation F h-- help (displays the menu of options) q-- quit All foundations and tableau columns will be entered as integer numbers. 5. Your program will detect, report, and recover from the following errors: a. Attempt to move a card in violation of the game rules b. Invalid menu option c. Invalid operands for menu options Notes: This assignment focuses on the design, implementation, and testing of a Python program that uses classes to solve the problem described below. You would be wise to familiarize yourself with the rules by actually playing the game. One website that allows you to play FreeCell online: http://games.aarp.org/games/freecell/freecell.aspx The general rules of the game are: - Start: - All 52 cards are dealt into eight columns (the tableau), where four of the columns contain seven cards each and four of the columns contain six cards each. - All of the cards are visible. - Objective: - The object of the game is to build up each foundation, in sequence, from Ace to King. - The player wins if they are able to move all 52 cards to the four foundations. - Only one card may be moved at a time. - Only the bottom card of each of the eight tableau columns and any cards in the free cells are eligible to be moved. - Once a card is moved onto a foundation pile, it cannot be moved again. - Any card may be moved from the tableau to an empty free cell (but each free cell may only hold one card at a time). - Any card may be moved from the tableau or the free cells to an empty column in the tableau. - The game is over when the player wins (by moving all 52 cards to the foundations), loses (can no longer make any moves), or resigns. Your program will permit the user to play FreeCell and will enforce the game rules. 1. Your program will use the card. py and card. py files provided with the assignment. You may not modify the contents of these files. 3. Your program must be subdivided into meaningful methods. 4. Your program will recognize the following menu of options: t2f T F -- move from Tableau T to Foundation F t2c T C -- move from Tableau T to Cell C t2t T1 T2 -- move from Tableau T1 to Tableau T2 c2t C T -- move from Cell C to Tableau T c2f C F -- move from Cell C to Foundation F h-- help (displays the menu of options) q-- quit All foundations and tableau columns will be entered as integer numbers. 5. Your program will detect, report, and recover from the following errors: a. Attempt to move a card in violation of the game rules b. Invalid menu option c. Invalid operands for menu options Notes
Step by Step Solution
There are 3 Steps involved in it
Step: 1
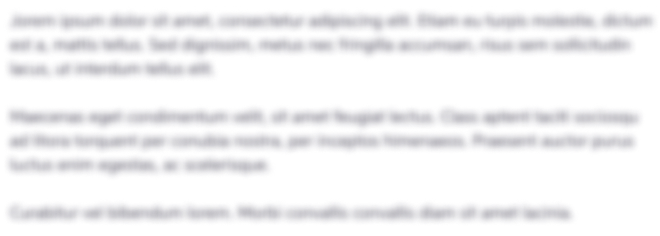
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started