Question
I need help on creating a priority queue implementing a binary heap in C++... Any help would be greatly appreciated. For this assignment, you will
I need help on creating a priority queue implementing a binary heap in C++... Any help would be greatly appreciated.
For this assignment, you will write three implementations of a Priority Queue. For this ADT, removal operations always return the object in the queue of highest priority, and if multiple objects have the same priority, the one in the queue the longest shall be returned. That is, no object of a given priority is ever removed as long as the queue contains one or more object of a higher priority. Within a given priority FIFO order must be preserved. This property is called stable sorting in a sorting algorithm, where two objects with equal keys will need to appear in the same sorted order as they appear in the unsorted order. Your implementations will be: Binary Heap
All implementations must have identical behavior, and must implement the PriorityQueue interface (provided). The implementations must have two constructors, a default constructor with no arguments that uses the DEFAULT_MAX_CAPACITY constant from PriorityQueue.h, and a constructor that takes a single integer parameter that represents the maximum capacity of the priority queue. The PriorityQueue interface follows:
#ifndef PA2_PRIORITY_QUEUE_PRIORITYQUEUE_H #define PA2_PRIORITY_QUEUE_PRIORITYQUEUE_H
#include
// The default max_capacity that must be used for default constructors. #define DEFAULT_MAX_CAPACITY 1000
// Struct Element that is inserted/removed from PriorityQueue typedef struct Element { // Name of element std::string name; // Priority of element int priority; // Time of insertion int fifo = 0;
// Default constructor of struct Element Element() : name("None"), priority(-1) {}; // Parameterized construct of struct Element Element(std::string s, int p) : name(std::move(s)), priority(p) {}; } Element;
// Node Struct to be used in PQLinkedList typedef struct Node { Element data; Node* next = nullptr; } Node;
class PriorityQueue { public:
// The maximum number of elements allowed in PriorityQueue int max_capacity;
// pq contains a smart pointer to an array of type struct Element // pq is used for PQOrdered and PQHeap, pq is NOT used for PQLinkedList std::unique_ptr
// Pointer to head Node for PQLinkedList // Use Node *head for PQLinkedList, Node *head is NOT used for PQHeap or PQOrdered Node* head;
public: // Default constructor PriorityQueue();
// Parameterized constructor explicit PriorityQueue(int size);
// Inserts a new object into the priority queue. Returns true if // the insertion is successful. If the PQ is full, the insertion // is aborted, and the method returns false. bool insert(Element& element);
// The object of the highest priority must be the one returned by the // remove() method; and if multiple objects have the same priority, // the one in the queue the longest shall be returned, ie, FIFO // return order must be preserved for objects of identical priority. // Returns the default constructed Element if the PQ is empty. Element remove();
// Deletes all instances of the parameter from the PQ if found, and // returns true. Returns false if no match to the parameter obj is found. bool del(Element& element);
// Returns the object of highest priority in the PQ; if multiple // objects have the same highest priority, return the one that has // been in the PQ the longest, but does NOT remove it. // Returns default constructed Element if the PQ is empty. Element peek();
// Returns true if the priority queue contains the specified element // false otherwise. bool contains(Element& element);
// Returns the number of objects currently in the PQ. int size();
// Returns the PQ to its default state. void clear();
// Returns true if the PQ is empty, otherwise false. bool isEmpty();
// Returns true if the PQ is full, otherwise false. bool isFull(); };
#endif //PA2_PRIORITY_QUEUE_PRIORITYQUEUE_H
Step by Step Solution
There are 3 Steps involved in it
Step: 1
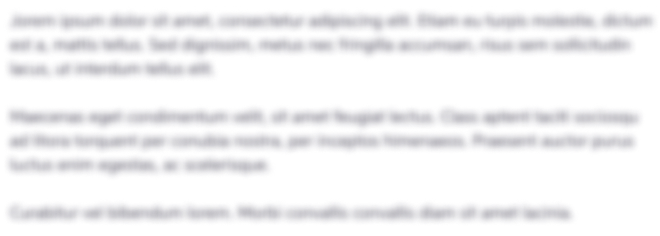
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started