Question
I need help programming this question using C. The code must follow these parameters and include the code below in bold. No global variables, no
I need help programming this question using C. The code must follow these parameters and include the code below in bold. No global variables, no arrays, and no recursive functions are supposed to be used.
int menu(); /* #1 */ void new_accounts(int *, float *, int *, float *); /* #2 */ void summary(int, float, int, float); /* #3 */ void withdraw(int, float *, float); /* #4 */ void deposit(int, float *, float); /* #5 */ void transfer(int, float *, int, float *, float); /* #6 */ void exchange(int, float *, int, float *); /* #7 */ int *high_balance(int *, float, int *, float, float *); /* #8 */ void reset(float *, float *); /* #9 */ void change(int *, int);
The program calls menu() to display possible transactions in the beginning and whenever a transaction is completed until Exit is selected.
The menu() always displays the menu as shown above and returns the selection (1, 2, ..., 9, 0). Every selection, except 0, can be repeated immediately, for instance, user can withdraw again right after a withdraw, or, create another two accounts to replace current ones.
The program stops execution when 0 is selected. In this case, GOOD BYE! is printed before end.
New Accounts. When 1 is selected, it calls new_account() to create two accounts. Existing accounts will be overridden by this transaction. At end, it prints a message as shown below to confirm both accounts are created.
- Account number is randomly picked between 55 and 59 but must not duplicate another account number.
- Initial balance of each account is also randomly determined when an account is created. Its value must between -100.00 and 999.99. Hint: first get a random integer between -10000 and 99999, then, divide it by 100.0 so it has two decimal digits but still a random number.
All Accounts. Whenever 2 is selected, it calls summary() to print each account's current information in the format as shown below.
Withdraw. When 3 is selected, the program first asks which account to withdraw. Note that both existing account numbers are included in the message prompt for user's convenience. Entering a non-existing account number results an error message (see the lower part in the figure below.) The program then asks the total amount to withdraw (i.e., the first big yellow part in the figure below.) Note that here user enters only the withdraw amount, not including '$'.
Once a valid account number and amount to withdraw are ready, the program calls withdraw() by passing values of these two numbers plus the account's balance. Because the balance is going to be updated after withdraw, it must be passed by reference to the withdraw() function so the updated value is accessible to the program. The withdraw result must be printed by this function, too (i.e., the blue part shown in the figure below.)
There is no upper/lower limit of withdraw amount and withdrawing from an account with negative balance is never a concern to this application.
Deposit. When 4 is selected at any time, the program performs exactly the same as Withdraw except it calls deposit() function instead of withdraw(). No screenshot here because of the similarity with the previous transaction.
Transfer Fund. When the selection is 5, the program transfers specified amount from one to the other account. It first asks to enter the source (or FROM) account and the destination (or TO) account, separated by at least one space. Like in deposit and withdraw, entering one or two non-existing account numbers results an error message as shown in pink of the figure below. Transferring from and to the same account also result an error as shown in blue of the figure below.
The last figure above shows '2' is selected to view the current accounts information (in green), followed by '5' to request a transfer of $7.27 from account 59 to 58.
When all input data are identified, the program calls transfer() by passing values of these three numbers plus each account's balance. Because the balance of each account is going to be updated after transfer, they must be passed by reference to the transfer() function so the updated values are accessible to the program. The transfer result of each account must also be printed by this function, too (i.e., the blue part shown in the figure below.)
Like in withdraw, there is no upper/lower limit of transfer amount and transferring from an account with negative balance is never a concern to this application.
Exchange Balance. When the selection is 6, user wants the balance of each account to be exchanged. Because this is treated as a serious transaction, the program requires user to confirm (see the yellow part below). When confirmed by 'y' or 'Y', the program calls exchange() to complete the job. Since both balances are affected, they need to be passed to this function by reference so their updated values can be accessible to the program. This function will also print the exchange result with account numbers (see the blue part below), therefore, they only need to be passed by value. Here passing account numbers by reference is not necessary because they are not modified. User can repeat this transaction immediately to undo or recover an exchange which is done by mistake.
High Balance. When the selection is 7, the program calls high_balance() to search if there is an account whose balance is $200 or higher than the other's. This is a special function that is different from all others of this program. It is required to return a pointer that points to the account which satisfies the search condition. Because of this, it requires to have both account numbers passed by reference such that the address (i.e., reference) of one of them can be returned. Please refer to section 11.5, p.251, Pointers as Return Values for an example that you can use to make this function.
The prototype of high_balance() shown in Coding Requirements tells it has a fifth parameter of float pointer. This is actually the amount of difference between the two accounts' balances. Because its value needs to be accessible to the caller, main(), it must be a parameter passed by reference.
If neither account's balance is $200 or higher than the other's, this high_balance() returns NULL as a null pointer that points to nowhere.
When the function ends, the program prints the search result (see the yellow part in both figures below), depending on what pointer it received from the function. Note that printed message also includes the balance of each account and the difference between the two balances indicated by pink underlines.
Both cases shown below begin with '2' to view the current account information. To test this transaction during program development, you can deposit to or withdraw or transfer from an account to set up a desired case for testing your high_balance() function.
Reset All Balance. When the selection is 8, the program requires user to confirm the request as in the exchange transaction. Once confirmed, it calls reset() to bring both balances down to zero. This function is the smallest and easiest function compared to others in the program. After resetting, it should also print the message as shown in blue below to confirm the transaction.
Change Account Number. When the selection is 9, the program first asks which account to re-assign its account number. Note that both account numbers are included in the message prompt for user's convenience. Entering a non-existing account number will result an error message (not shown below) exactly the same as in deposit or withdraw or other transactions.
Once an account is identified, the program calls change() to re-assign it a new account number. Because the same rule of no duplicate account numbers applies here, this function needs to know the other account number, although it is not the target to change. As a result, the account that is going to change is passed by reference while the other is passed by value. This is handled by the program when the function is called, depending on which one is entered by user for a new account number. Whichever is changed, the function must show a message to conclude it (i.e., the blue part below).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
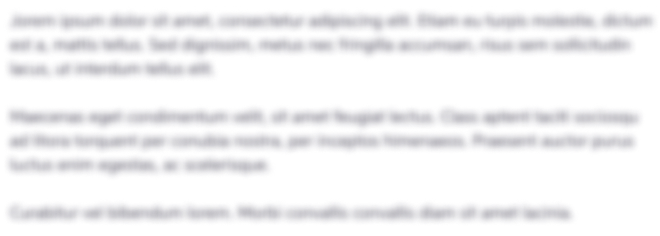
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started