Question
I need help using JavaFX Static Classes The code for Assignment 6 has already been completed, which I included, but need help improving upon in
I need help using JavaFX Static Classes
The code for Assignment 6 has already been completed, which I included, but need help improving upon in Assignment 7.
Here is a description for what this code does:
The purpose of this assignment is to get you familiar with the basics of the JavaFX Events. In particular you will need to be familiar with ActionEvents and MouseClicked eventsThis game begins with all three cards showing the card back and it being right's turn to select a card. A card is selected by clicking the mouse on the middle card image. A card is randomly generated and assignment to the right label. The next card selected is assigned to the left. The two cards are then compared and the score updated on which ever card had a higher value. Ties are awarded no points but I should point out that in the deck the Ace of diamonds has a smaller value than the ace of spades.
In order for the program to run properly, you will need to download the card images. These images can be downloaded here:
https://mega.nz/#!tBJD3YLZ!YjGYuP7d4-6Etq1J_i4o3XTNVqjqjyaIhIxkGgaSLGw
The program that I already wrote, Assignment 6 is separated into four Java files: Card.java, Deck.java, Lab6.java, and Suit.java.
Here is the code for Lab6.java:
import javafx.scene.text.*; import javafx.application.Application; import javafx.event.*; import javafx.scene.image.*; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.scene.layout.*; import javafx.stage.Stage;
import java.util.Random;
import javafx.scene.paint.Color; import javafx.geometry.*; import javafx.scene.input.MouseEvent;
public class Lab6 extends Application {
public boolean rightsTurn = true; int leftCounter = 0; int rightCounter = 0; int rightVal = 0; int leftVal = 0;
TextField tf1 = new TextField(); TextField tf2 = new TextField();
Card cardLeft; Card cardDeck; Card cardRight;
Label testNum1 = new Label(""); Label testNum2 = new Label("");
public void start(Stage primaryStage) {
// Creates card object that contains a label with the image from the // file passed in the setImage method cardLeft = new Card(); cardDeck = new Card(); cardRight = new Card();
Font font = new Font("Courier", 25); Font font2 = new Font("Courier New", 20); Color clr = Color.web("#0076a3"); Color clr2 = Color.web("#FF0000");
Label lblscore = new Label(); lblscore.setFont(font); lblscore.setTextFill(clr); lblscore.setText("Score: ");
Label left = new Label("Left:"); Label right = new Label("Right:");
Button btnreset = new Button(); btnreset.setText("reset");
int num; String filename = null; int num2; String filename2 = null; btnreset.setOnAction(new EventHandlerImpl());
BorderPane root = new BorderPane(); GridPane top = new GridPane(); GridPane middle = new GridPane(); root.setTop(top); root.setCenter(middle); root.setBottom(btnreset); top.setHgap(20); top.setVgap(10); top.add(lblscore, 0, 0); top.add(new Label("left:"), 0, 2); top.add(new Label("right:"), 2, 2); top.add(tf1, 1, 2); top.add(tf2, 3, 2);
// Again the label is inside the card object middle.add(cardLeft.getCard(), 0, 0); middle.add(cardDeck.getCard(), 1, 0); middle.add(cardRight.getCard(), 2, 0);
middle.setHgap(20); middle.setAlignment(Pos.CENTER); Scene scene = new Scene(root, 600, 450); primaryStage.setTitle("Lab 5df"); primaryStage.setScene(scene); primaryStage.show(); this.resetCardImages(cardLeft.getCard(), cardDeck.getCard(), cardRight.getCard());
cardDeck.getCard().setOnMouseClicked(new EventHandler() {
@Override public void handle(MouseEvent event) { System.out.println("A"); Random random = new Random(); if (rightsTurn == true) { int num = random.nextInt(154 - 101 + 1) + 101;
String filename = Integer.toString(num) + ".gif"; // Instead of labels we use cards now which contain their // own label cardRight = new Card("file:img\\" + filename); rightVal = cardRight.getValue(); } else { int num2 = random.nextInt(154 - 101 + 1) + 101; String filename2 = Integer.toString(num2) + ".gif"; cardLeft = new Card("file:img\\" + filename2); leftVal = cardLeft.getValue();
}
if (rightVal > leftVal) { rightCounter = rightCounter + rightVal; String rightScore = Integer.toString(rightCounter); tf2.setText(rightScore);
} else if (leftVal > rightVal) { leftCounter = leftCounter + leftVal; String leftScore = Integer.toString(leftCounter); tf1.setText(leftScore);
}
rightsTurn = !rightsTurn; System.out.println(rightsTurn);
middle.getChildren().remove(cardLeft.getCard()); middle.getChildren().remove(cardRight.getCard());
middle.add(cardLeft.getCard(), 0, 0);
middle.add(cardRight.getCard(), 2, 0); }
/* * * @Override public void handle(Event event) { throw new * UnsupportedOperationException("Not supported yet."); } */ }); }
public void resetCardImages(Label x, Label y, Label z) {
// Sets the default image in the card and then sets the cards label to // the correct image cardLeft = new Card(); cardRight = new Card();
}
public static void main(String[] args) { launch(args); }
private class EventHandlerImpl implements EventHandler {
public EventHandlerImpl() { }
@Override public void handle(ActionEvent event) { rightVal = 0; leftVal = 0; rightCounter = 0; leftCounter = 0; tf1.setText("0"); tf2.setText("0"); rightsTurn = true;
resetCardImages(cardLeft.getCard(), cardDeck.getCard(), cardRight.getCard()); }
/* * public void handle(Event event) { throw new * UnsupportedOperationException("Not supported yet."); } */ } }
Here is the code for Card.java:
import java.util.Arrays;
import com.sun.org.apache.xalan.internal.xsltc.compiler.Pattern;
import javafx.scene.control.Label; import javafx.scene.image.Image; import javafx.scene.image.ImageView;
public class Card { private int value; private Image imgCard; //Cards Image private Label lblCard; //Cards label which contains the image private String path; //path to image private Suit suit; //Overloaded constructor public Card(String path) { setImage(path); } //Default constructor public Card() { setImage("file:img\\155.gif"); } //Takes a string with the path of the image and loads the image into the label public boolean loadCard(String path){ imgCard = new Image(path); lblCard = new Label("",new ImageView(imgCard)); lblCard.setGraphic(new ImageView(imgCard)); getCardValue(path); return true; } //Sets the image for this card public void setImage(String path){ this.path = path; loadCard(path); } public void getCardValue(String path){ String[] splitDot = path.split("\\."); //Splits the String at the dot //Takes the first part of the string (before the dot) and splits it at the backslash String[] splitSlash = splitDot[0].split("\\\\"); //The number part of the path String fileNumber = splitSlash[1]; //Converts the number string to an int int cardSuit = Integer.parseInt(fileNumber); //Selects the suit based on the cards filename if(cardSuit <= 113){ suit = Suit.Diamonds; } else if(cardSuit <= 126){ suit = Suit.Clubs; } else if(cardSuit <= 139){ suit = Suit.Hearts; } else { suit = suit.Spades; } //Divides the cards number by 100 to turn it into a number from 0 to 52 int cardValue = Integer.parseInt(fileNumber) % 100; //Divides by 13 to get the cards value from 1 to 13 regardless of suit cardValue %= 13; //Calculates the value based on filename if(cardValue > 1 && cardValue <= 10){ value = cardValue; } else if(cardValue == 1){ value = 11; } else if(cardValue > 10 && cardValue <=13){ value = 10; } else { value = 0; } System.out.println(value); } public Suit getSuit(){ return suit; } public int getValue(){ return value; } public Label getCard(){ return lblCard; } @Override public String toString() { return path; }
}Here is the code for Deck.java:
import java.util.ArrayList; import java.util.Collections;
public class Deck { int index; // The current card to be dealt final int LAST_CARD = 45; //After this index reshuffle ArrayList deck = new ArrayList<>(); //List of game cards //Overloaded constructor public Deck(String pathToCards) { loadCards(pathToCards); } //Default constructor loads ("file:img\\") public Deck() { loadCards("file:img\\"); }
public void shuffle(){ Collections.shuffle(deck); } //Creates each card and loads it with the correct image,then adds it to the deck public void loadCards(String pathToCards){ for(int i = 101; i <= 152; i++){ Card currentCard = new Card(pathToCards + i + ".gif"); deck.add(currentCard); System.out.println(currentCard); } } //returns a card that was dealt and increments the index so it doesnt return the same //card every time public Card deal(){ if(index > LAST_CARD){ shuffle(); } index++; return deck.get(index); }
}
Here is the code for Suit.java:
public enum Suit { //Enum with all possible Suits
Diamonds, Hearts, Spades, Clubs }
Here are the instructions for Assignment 7 (The changes that I need help making to this code):
Purpose
The purpose of this assignment is to show an understanding of the use of static classes.
In this assignment you will create a static class that will be used to keep track of the score for the simplified war game you have been working on.
Instructions
Create a class called Score. This class should have two static int variables to keep track of the score for each side right and left.
Inside the Score class create static over loaded mutator methods called setRightScore. One of these methods should take as an argument an int. The other method should take a string as an argument. These methods should increase the score by the amount passed in, It is important to note that the class that takes the String needs to convert the String to a number.
In addition, create overloaded mutator methods called setLeftScore. These methods should work the same as the methods setRightScore except they should increment the field variable used to keep track of the left players score.
Create accessor methods called getRightScore and getLeftScore that will simply produce the score for each side.
Create a method called resetScore that sets the field variables to 0.
Modify The Game
Inside the main file you need to modify the game so that it uses the static class you have just created. This means anywhere you update the score and output the score you should use the Score class.
Notes
This is a static class and it should be used as such.
At this point the Score class only keeps track of the score but it may be used later on for further functionality
Any help is greatly appreciated. Thank you so much!!! :)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
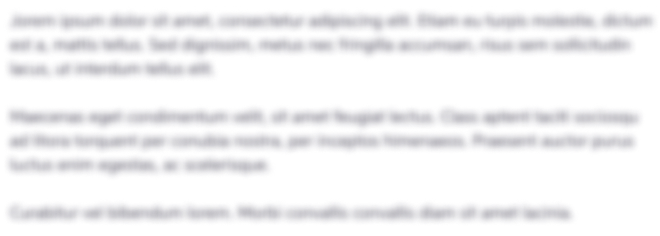
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started