Question
I need help with a java program. The job is to write the definition of these two methods: 1. static String[] upSize( String[] fullArray );
I need help with a java program. The job is to write the definition of these two methods:
1. static String[] upSize( String[] fullArray ); // incoming array is FULL. It needs it's capacity doubled
2. static String[] downSize( String[] array, int count ); // incoming array most likely NOT full and needs down-sized to be just big enough to hold only the initialized elements. Like shrink wrapping the array to eliminate those wasted empty elements. Makes .length == count.
The program you are given to start with: Project6.java reads a file of Strings into an array but it has a problem. The array is dimensioned with an initial capacity of only ten elements. Inside the while loop is an if test that compares the current wordCount to the current capacity (.length) of the array. If the array is full, a method named upSize( String[] fullArr) (which you must write) is called that performs the following:
1. allocates a new array that is twice the capacity of the one passed in that got full
2. copies all the values from the full array into the new (bigger) array
3. returns the address (reference) of the new (bigger) array
Each time this upSize(...) method returns, the capacity of the new (bigger) array will be printed out. The file you are given will do all the output for you. Please do not write any output statements. After the loop terminates the program will call a similar method called downSize( String[] arr,int count) (which you must write) that performs the following:
1. allocates a new array that is just big enough to hold all the data from the arrays passed in.
2. copies all the values from the array passed in, into the new array that is just big enough to hold them all
3. return the address (reference) of the new (shrink wrapped) array.
After the array is downsized to shrink-wrap fit the data, the new .length (capacity) and wordCount will be reported. The file you are given will do all the output for you. Please do not write any output statements.
Here is the starter file!
/* Project6.java Resizes an array every time it fills up while reading a large file. */ import java.io.*; import java.util.*; public class Project6 { static final int INITIAL_CAPACITY = 5; public static void main (String[] args) throws Exception { // ALWAYS TEST FIRST TO VERIFY USER PUT REQUIRED INPUT FILE NAME ON THE COMMAND LINE if (args.length < 1 ) { System.out.println(" usage: C:\\> java Project6 dictionary.txt "); System.exit(0); } String[] wordList = new String[INITIAL_CAPACITY]; int wordCount = 0; BufferedReader infile = new BufferedReader( new FileReader(args[0]) ); int numOfUpsizes=0; while ( infile.ready() ) // i.e. while there are more lines of text in the file { String word = infile.readLine(); if ( wordCount == wordList.length ) // an array's .length() is its CAPACITY, not count { wordList = upSize( wordList ); System.out.format( "after resize#%d length=%d, count=%d ",++numOfUpsizes,wordList.length,wordCount ); } wordList[wordCount++] = word; // Now wordList has enough capacity } //END WHILE INFILE READY infile.close(); System.out.format( "after file closed: length=%d, count=%d ",wordList.length,wordCount ); wordList = downSize( wordList, wordCount ); System.out.format( "after final down size: length=%d, count=%d ",wordList.length,wordCount ); printFirstTenWords( wordList ); // IF THERE ARE LESS THAN 10 IN THE ARRAY THEN JUST PRINT THOSE printLastTenWords( wordList ); // IF THERE ARE LESS THAN 10 IN THE ARRAY THEN JUST PRINT THOSE } // END MAIN // = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = // DO NOT MODIFY THESE TWO METHODS static void printFirstTenWords( String[] wordList ) { final int MAX_WORDS_PER_LINE = 10; int count = wordList.length
Step by Step Solution
There are 3 Steps involved in it
Step: 1
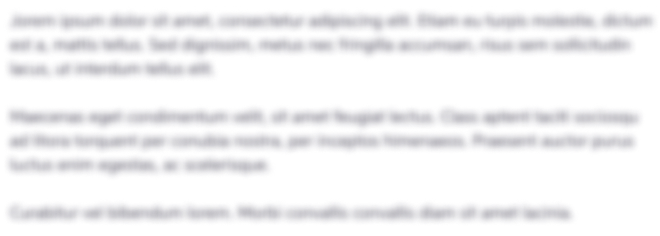
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started