Question
I need help with my C++ code! My teacher has created an assignment that detects if a triangle is EQUILATERAL, ISOCELES, RIGHT, OTHER, ERROR... I
I need help with my C++ code! My teacher has created an assignment that detects if a triangle is EQUILATERAL, ISOCELES, RIGHT, OTHER, ERROR... I have passed all his testfiles except for these:
Input:
.5 .4 .3
output:
right
Input:
1 1 0.00000000000001
Output: isoceles
My Code:
//YOU: York, Anderson #include
//Enum is useful when you want to make large numbers of mutually exclusive constants //This creates 5 constant integers, named EQUILATERAL (= 0), ISOCELES (= 1), etc. //It also creates a new integer type that can only hold a value from 0 to 4. enum triangle_t { EQUILATERAL, ISOCELES, RIGHT, OTHER, ERROR };
/* This is equivalent, kinda, to: const int EQUILATERAL = 0; const int ISOCELES = 1; const int RIGHT = 2; const int OTHER = 3; const int ERROR = 4; */
triangle_t triangle_tester(float side1, float side2, float side3) { //YOU: Do your work right here if ((!cin) || (side1 <= -1) || (side2 <= -1) || (side3 <= - 1) || (side1 + side2 <= side3) || (side1 + side3 <= side2) || (side2 + side3 <= side1)) return ERROR;
if (side1 == side2 and side2 == side3) return EQUILATERAL; else if (side1 == side2 or side2 == side3 or side1 == side3) return ISOCELES; else if (pow(side1, 2) + pow(side2, 2) == pow(side3, 2) || pow(side1, 2) + pow(side3, 2) == pow(side2, 2) || pow(side2, 2) + pow(side3, 2) == pow(side1, 2)) return RIGHT;
return OTHER; }
int main() { float side1 = 0.0, side2 = 0.0, side3 = 0.0; cin >> side1 >> side2 >> side3;
triangle_t result = triangle_tester(side1, side2, side3);
if (result == EQUILATERAL) cout << "equilateral"; else if (result == ISOCELES) cout << "isoceles"; else if (result == RIGHT) cout << "right"; else if (result == OTHER) cout << "other"; else if (result == ERROR) cout << "error"; else cout << "This is unpossible!"; cout << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
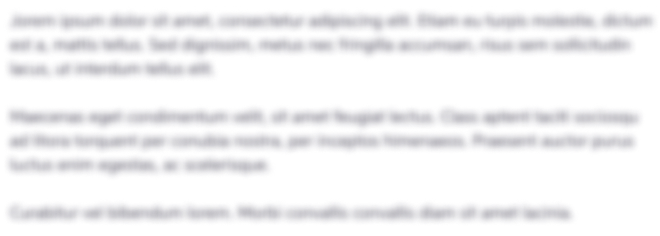
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started