Question
I need help with my Java project. We had to create individual properties files (not text files) for our directions except for unknown in a
I need help with my Java project.
We had to create individual "properties" files (not text files) for our directions except for "unknown" in a folder called "Resources" in the project, mine read as n.properties has n=north, down.properties has down=d and so on for all of the directions you will see in my enum declaration (N, S, E, W, NE, NW, SE, SW, UP, DOWN, UNKNOWN). I have them listed below as how they are named and what the key=value that is in them.
After creating the .properties files with our directions, we had to create an Enum Direction with the enum directions, a way to read our properties files into an array list, and then two methods boolean isSynonym(String possibleAlt) and Direction findSynonym(String possibleAlt)- the way my methods are set up are how I am supposed to have them set up, BUT I am having a problem running my tests.
Lastly, we had to create a DirectionTest with JUnit tests- currently my testIsSynonym1() and testIsSynonym2() are failing and I can't figure out what to do- the test comes back saying "Null Pointer Exception".
What I know:
My properties files are reading into the arraylist and when I print them- it works. Meaning I think that my problem is cpmparing to my Enum constants- you will see a commented out if statement in the first line of my boolean method because I cannot figure out what will work for my if(____.equalsIgnoreCase(possibleAlt) return true statement- I THINK (but am not sure) this is a line that needs to be fixed and added for my code to work.
I have provided my codes for the enum Direction and DirectionTest below and have bolded where I THINK my problem is and the two tests that are currently failing.
Direction:
import java.util.ArrayList; import java.util.List; import java.io.File; import java.io.FileInputStream; import java.util.Scanner; import java.io.FileNotFoundException; import java.io.IOException; import java.util.Properties;
public enum Direction { /** * We are creating an enum to hold constants for our directions, * and be able to read from our properties files to determine synonyms for each direction * so that we may define all possible directions that a player can travel. * */ N(), S(), E(), W(), NE(), NW(), SE(), SW(), UP(), DOWN(), UNKNOWN();//Declare enum constants String dir; Direction (String dir) { this.dir= dir; } private ArrayList
/** * public static Direction findSynonym(java.lang.String possibleAlt) * * enum-wide method to provide a more friendly valueOf method. * We take a String representation of an enum value or synonym and, ignoring case, * return the corresponding Direction enum value. This method steps through every possible enum value, * which it can do using values(). It then tests whether possibleAlt matches one of those values. * (See isSynonym) * Parameters: * possibleAlt - String representing a case-insensitive, synonym-based Direction value * Returns: * the Direction value associated with possibleAlt, or Direction.UNKNOWN * @return */ public static Direction findSynonym(String possibleAlt) { for(Direction s: values()) { if(s.isSynonym(possibleAlt)) return s; } return Direction.UNKNOWN; } /** * public boolean isSynonym(java.lang.String possibleAlt) * Determine whether a given String value represents this Direction value ignoring case, * or one of its synonyms ignoring case. * Parameters: * possibleAlt - a possible String representation of this Direction or one of its synonym values * Returns: * true if possibleAlt is a valid String value for this Direction or one of its synonyms, all ignoring case */ public boolean isSynonym(String possibleAlt) { //if(Direction.equalsIgnoreCase(possibleAlt)) //return true; for(String s: directions) { if(s.equalsIgnoreCase(possibleAlt)) return true; } return false; } }
Direction Test:
@Test public void testIsSynonym0() { Direction d = Direction.findSynonym("misspelled"); assertEquals(Direction.UNKNOWN, d); } /** * Test that Direction.findSynonym("n") returns Direction.N * */ @Test public void testIsSynonym1() { Direction d = Direction.findSynonym("n"); assertEquals(Direction.N, d); } /** * Test that Direction.findSynonym("down") returns Direction.DOWN * */ @Test public void testIsSynonym2() { Direction d = Direction.findSynonym("down"); assertEquals(Direction.DOWN, d); } /** * Test that Direction.findSynonym("d") returns Direction.DOWN * */ @Test public void testIsSynonym3() { Direction d = Direction.findSynonym("d"); assertEquals(Direction.DOWN, d); } /** * Test that Direction.N.isSynonym("North") is true * */ @Test public void testIsSynonym4() { Direction d = Direction.N; assertTrue(d.isSynonym("North")); }
Properties Files that are stored in a Resources folder within my project:
n.properties n=north
s.properties s=south
w.properties w=west
e.properties e=east
ne.properties ne=northeast
nw.properties nw=northwest
se.properties se=southeast
sw.properties sw=southwest
up.properties up=u
down.properties down=d
Step by Step Solution
There are 3 Steps involved in it
Step: 1
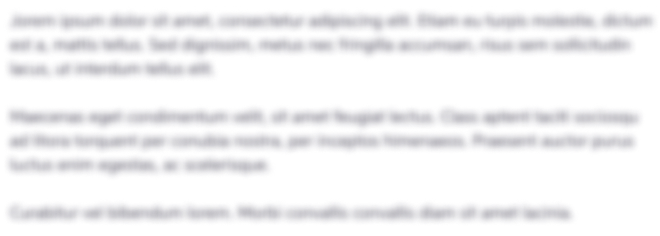
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started