Question
I need help with Q4 and 5. Attached below is the question. Thanks My CODE: //Q1: Set up a grid on the canvas. Draw a
I need help with Q4 and 5. Attached below is the question. Thanks
My CODE:
//Q1: Set up a grid on the canvas. Draw a "mine" cell // and a "number of mines nearby" cell. //Q2: Add functions to draw the grid, a list of mines and // a list of number cells.
final int CELLSIZE = 50; final int NUM_ROWS = 10; final int NUM_COLS = 12; final int MIN_MINES=10; final int MAX_MINES=15;
//global arrays to hold mine column and row nos int[] mineX= new int[MAX_MINES]; //column no of mine int[] mineY= new int[MAX_MINES]; //row no of mine
void setup() { size(600, 500); //size MUST be (NUM_COLS*CELLSIZE) by (NUM_ROWS*CELLSIZE); int mineCount= (int) random (MIN_MINES, MAX_MINES+1); generateMines(mineCount); }
void draw() { drawGrid(); drawMines(mineX, mineY, 6); //fill the cells with mines }
//to search the first n positions in the arrays named x and y boolean search(int[]x, int[]y, int n, int col, int row) { for (int i=0; i //to fill arrays with randomly generated row and column numbers, making sure that each position is unique void generateMines(int noOfMines) { int randRow, randCol; for (int i=0; i insert(mineX, mineY, i, randCol, randRow); } } //to insert postion(col,row) into given arrays as long as ther is room int insert(int[]x, int[]y, int n, int col, int row) { int minLen= Math.min(x.length, y.length); if (n==minLen) { return n; } x[n]= col; y[n]= row; return n+1; } //**add your drawGrid function here** void drawGrid() { final int HEIGHT=700; //draw the grid for (int i=0; i //**add your drawMines function here** void drawMines(int[] cols, int[] rows, int entries) { for (int i=0; i //**add your drawNums function here** void drawNums(int[] cols, int[] rows, int []vals, int entries) { for (int i=0; i //**paste your drawMine and drawNum functions from Q1 here** //**add your drawMine function here** void drawMine(int row, int col) { int stroke=3; //draws mine in specified cell //draw mine design fill(255); stroke(0); strokeWeight(1); rect(CELLSIZE*row, CELLSIZE*col, CELLSIZE, CELLSIZE); fill(0); ellipse(CELLSIZE*row + (CELLSIZE/2), CELLSIZE*col + (CELLSIZE/2), 3*NUM_ROWS, 3*NUM_ROWS); stroke(255, 0, 255); strokeWeight(stroke); line(CELLSIZE*row + stroke, CELLSIZE * col + (CELLSIZE/2), CELLSIZE * row + CELLSIZE- stroke, CELLSIZE * col + (CELLSIZE/2)); line(CELLSIZE*row + (CELLSIZE/2), CELLSIZE * col + stroke, CELLSIZE*row + (CELLSIZE/2), CELLSIZE*col + CELLSIZE- stroke); stroke(255); strokeWeight(0); fill(128, 128, 128); ellipse(CELLSIZE*row + (CELLSIZE/2), CELLSIZE * col + (CELLSIZE/2), 2*NUM_ROWS, 2*NUM_ROWS); fill(0, 255, 0); ellipse(CELLSIZE*row + (CELLSIZE/2), CELLSIZE*col + (CELLSIZE/2), NUM_ROWS, NUM_ROWS); } //**add your drawNum function here** void drawNum(int row, int col, int val) { final int OFFSETX= 15; final int OFFSETY= 39; //draw and center text and place in box fill(255); stroke(0); strokeWeight(1); rect(row*CELLSIZE, col*CELLSIZE, CELLSIZE, CELLSIZE); fill(0, 0, 255); textSize(35); text(val, row * CELLSIZE+OFFSETX, col * CELLSIZE + OFFSETY); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
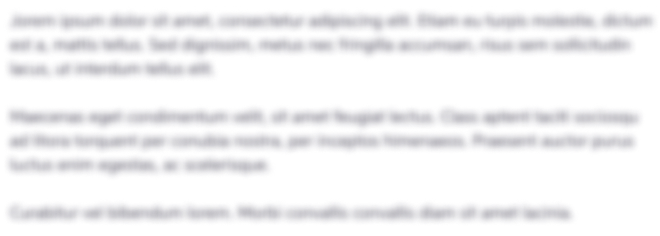
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started