Question
I need help with the flowchart, pseudocode, and UML diagram. Circle2D.java public class Circle2D { private double x; private double y; private double radius; public
I need help with the flowchart, pseudocode, and UML diagram.
Circle2D.java |
public class Circle2D { private double x; private double y; private double radius; public Circle2D() { this.x = 0; this.y = 0; this.radius = 1.0; } public Circle2D(double x, double y, double radius) { this.x = x; this.y = y; this.radius = radius; } public double getX() { return this.x; } public double getY() { return this.y; } public double getRadius() { return this.radius; } public double getPerimeter() { return 2*Math.PI*this.radius; } public double getArea() { return Math.PI*(this.radius*this.radius); } public boolean contains(double x, double y) { double d = Math.sqrt(Math.pow((x - this.x), 2) + Math.pow((y - this.y), 2)); if(d < this.radius) return true; else return false; } public boolean contains(Circle2D circle) { double d = Math.sqrt(Math.pow((circle.x - this.x), 2) + Math.pow((circle.y - this.y), 2)); if(d + circle.radius < this.radius) return true; else return false; } public boolean overlaps(Circle2D circle) { double d = Math.sqrt(Math.pow((circle.x - this.x), 2) + Math.pow((circle.y - this.y), 2)); if(d <= circle.radius + this.radius) return true; else return false; } } |
TestCircle2D.java |
public class TestCircle2D { public static void main(String arg[]) { Circle2D c1 = new Circle2D(2, 2, 5.5); System.out.println("Area: " + c1.getArea() + " sq units"); System.out.println("Perimeter: " + c1.getPerimeter() + " units"); System.out.println("c1.contains(new Circle2D(2, 2, 5.5)): " + c1.contains(new Circle2D(2, 2, 5.5))); System.out.println("c1.overlaps(new Circle2D(4, 5, 10.5)): " + c1.overlaps(new Circle2D(4, 5, 10.5))); } } |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
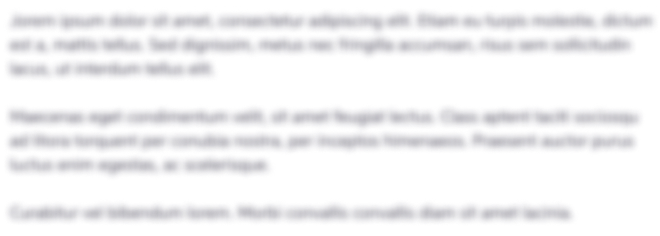
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started