Question
I need help with this java assigment, I have my code provided and documents to help. Im not sure how to do GUIs package lab1in;
I need help with this java assigment, I have my code provided and documents to help. Im not sure how to do GUIs
package lab1in;
import java.awt.Color; import java.awt.Frame; import java.awt.GridBagConstraints; import java.awt.GridBagLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener;
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel;
public class ClientGUI extends JFrame {
// Variables declaration private JButton connect; private JPanel north; private JLabel output; private JPanel south; private JLabel status; private JButton stop; private JButton submit; //contructor that passes one parameter public ClientGUI(String title) { this.setTitle(title); //set the title GridBagConstraints gridBagConstraints;
north = new JPanel(); status = new JLabel(); output = new JLabel(); south = new JPanel(); connect = new JButton(); submit = new JButton(); stop = new JButton();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); java.awt.GridBagLayout layout = new java.awt.GridBagLayout(); layout.columnWidths = new int[] {0, 15, 0, 15, 0, 15, 0, 15, 0, 15, 0, 15, 0, 15, 0, 15, 0, 15, 0, 15, 0}; layout.rowHeights = new int[] {0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0, 10, 0}; getContentPane().setLayout(layout);
status.setText("Status"); north.add(status);
output.setText("output"); output.setName("lblOutput"); output.setForeground(Color.RED); north.add(output);
//set the button label to the grid panel gridBagConstraints = new GridBagConstraints(); gridBagConstraints.gridx = 6; gridBagConstraints.gridy = 0; getContentPane().add(north, gridBagConstraints); gridBagConstraints = new GridBagConstraints(); gridBagConstraints.gridx = 2; gridBagConstraints.gridy = 0; getContentPane().add(south, gridBagConstraints);
connect.setText("Connect"); connect.setName("connect"); connect.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent evt) { connectActionPerformed(evt); System.out.println("Connect button pressed"); } }); gridBagConstraints = new GridBagConstraints(); gridBagConstraints.gridx = 6; gridBagConstraints.gridy = 32; getContentPane().add(connect, gridBagConstraints);
submit.setText("Submit"); submit.setName("submit"); submit.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent evt) { submitActionPerformed(evt); System.out.println("Submit button pressed"); } }); gridBagConstraints = new GridBagConstraints(); gridBagConstraints.gridx = 10; gridBagConstraints.gridy = 32; getContentPane().add(submit, gridBagConstraints);
stop.setText("Stop"); stop.setName("stop"); stop.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent evt) { stopActionPerformed(evt); System.out.println("Stop button pressed"); } }); gridBagConstraints = new GridBagConstraints(); gridBagConstraints.gridx = 14; gridBagConstraints.gridy = 32; getContentPane().add(stop, gridBagConstraints);
pack(); } //connect button press event private void connectActionPerformed(ActionEvent evt) { String show="Connect Button Pressed"; output.setText(show); }
//submit button press event private void submitActionPerformed(ActionEvent evt) { String show="Submit Button Pressed"; output.setText(show); }
//stop button press event private void stopActionPerformed(ActionEvent evt) { String show="Stop Button Pressed"; output.setText(show); }
//main method public static void main(String args[]) { ClientGUI cg = new ClientGUI(args[0]); cg.setVisible(true); } }
(30 Points) An interface for the Client process of our Chat system is required. Modify the Java Class named I (started in lablin) so its GUI now appears as shown below: Client #1 Status: Not C (not editable) Client ID (editable) Server URL Server Port Enter Clent Data (should be contained within a ISCrollPane so scroll bars can be added automatically as needed) Label Received Server JTcxtArea (should be contained within a ISCrollPane so scroll bars can be added as needed) This should not be editable. Connect Submit Stop The following datafields should now be declared in the ClientGUl class started in lablin private La tatus; //From Lab 1 In private 3Button connec private JButton submit; //From Lab 1 In private Button stop; private String[] labels ("Client ID", "Server URL", "Server Port"; private TextField[] textElelds- new TextField[Labelsdength]; private JTextAcea, lisntAcea. t; I/From Lab 1 In //From Lab 1 In (Feel free to implement other data fields if you like) Where each data field corresponds to a newly added user interface component. The TextEields, should be implemented as an array. Use the labels array to set the corresponding JLabel preceding each ITextField. The JTextArea beneath the label "Enter Client Data Below" corresponds to the clientArea JTextArea The JTextArea JTextArea beneath the label Received Server Data" corresponds to the serverArea. These data fields should be initialized in the ClientGIconstructor (started in lablin) whose method header is shown below (same as lablin): public Glientdut(String title) Note: The "Client ID" field is not editable. In a future lab this client id will automatically be assigned by the Server once the client has connected to the Server. The JTextArea beneath the "Received Server Data" should also be set to not be editable ClientU is a public class and should extend the JErame class (same as lablin) ClientGUI Class Methods The ClientGUI class should implement the following methods (feel free to implement other methods if you like): Constructor public ClientGUI(String title) Constructor for ClientGUIl class. This method creates and displays the interface on page 1. The title is used for setting the title of the JErame created by this method. Event Handling for JButtons The same event handler used in lablin (e.g., EventHandler class) can also be used for lablout For now, the following actions should occur when pressing any of the JButtons: When pressing Connect display "Connect Button Pressed" on the Console (same as lablin) When pressing Submit display the data entered in the JTextArea corresponding to the client data (corresponding to the clientData data field) preceded by the phrase "Client Data:" on the Console Example Console output: Client Data: Entered Data in Client Area This text was entered in the clientData's JTextArea When pressing Stop display "Stop Button Pressed" on the Console Server GUIGuidelines An interface also needs to be implemented for the Server process of our Chat system. An example for the Server GUI interface is shown below: JLabel TxtField TcxtField Server Status: Not Connected Port # Timeout Server Log Below JLabel " (should be contained within a JSCrollPane so scroll bars can be added as needed) Listen Close You need to create the following class that extends JFrame public class ServerGUI extends 3Ecane //Data Fields go here //Methods go here Data Fields You should declare data fields that correspond to the status label, textEields, and the as shown below (feel free to implement any other data fields that you like): extatea private Label status; //Initialized to "Not Connected" private string[] labels ("Port #", "Timeout"); private TextArea log; Methods You should implement the following methods (feel free to implement any other methods that you like): public ServerUString title) Constructor - creates the Server GUI shown above. Input parameter ttle is used for setting the title bar of the Server GUI. Event Handling for JButtons. For now, the following actions should occur when pressing the Buttons For now, the following actions should occur when pressing the Buttons When pressing Listen display "Listen Button Pressed" on the Console When pressing Close display "Close Button Pressed" on the Console When pressing Stop display "Stop Button Pressed When pressing Quit close the GUI (same as pressing the Red X in upper right corner) Guidelines for Main ClientGUI and ServerGUI must have their own main methods. The main methods only need to instantiate an object pertaining to each respective class. The Clientaul's main needs at a minimum the following statement new Slientuase]); Where args[0] corresponds to the one command line argument pertaining to the desired title of the Client's GUI The ServerGUl's main needs at a minimum the following statement new Serveae]); Where args[0] corresponds to the one command line argument pertaining to the desired title of the Server's GUIStep by Step Solution
There are 3 Steps involved in it
Step: 1
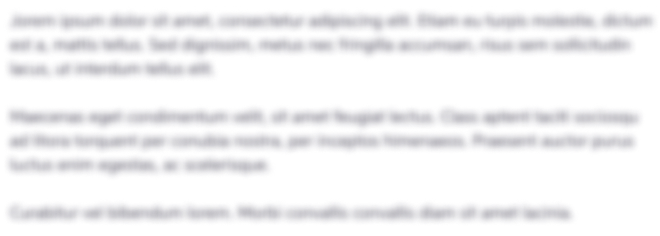
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started