Question
I need help with this Java code. If I can at least get some help starting this. Code: public class ReverseInt { private static MyQueue
I need help with this Java code. If I can at least get some help starting this.
Code:
public class ReverseInt
{
private static MyQueue que = new MyQueue();
private static MyStack stk = new MyStack();
public static void main (String[] args)
{
int num = 1234567; // original number
int rev = 0; // reversed number
// (1) Call revIntRec passing in num
revIntRec (num);
// (2) Loop through queue:
// Dequeue each digit and multiply by the appropriate power of 10
// Start with the highest power of ten, use queue size-1
// Add these terms to get the reversed number which you
// should store in rev.
//todo
System.out.println("Original: " + num + " Reversed: " + rev);
// (3) Call revIntStk passing in reversed number - revIntRec (int num)
// This is the number you built in step 2.
revIntRec (rev);
// (4) Loop through stack:
// Pop each digit and multiply by the appropriate power of 10
// Start with the lowest power of ten
// Add these terms to get the original number
//todo
System.out.println("Reversed: " + rev + " Original: " + num);
}
// Using a loop:
// Remove each last digit from num using the % operator
// Add each last digit to the stack
// With each round of the loop reduce num by dividing by 10
// Base case is when num is less than 10
public static void revIntStk (int num)
{
//todo
}
// Remove each last digit from num using the % operator
// Add each last digit to the queue
// With each round of recursion reduce num by dividing by 10
// Base case is when num is less than 10
public static void revIntRec (int num)
{
// Base case: If num < 10
// Enqueue last digit and return
// todo
// else Not Base case:
// Enqueue last digit and recurse
//todo
}
}
MyStack code:
import java.util.ArrayList;
public class MyStack
public int getSize() { return list.size(); }
public E peek() { return list.get(getSize() - 1); }
public void push(E o) { list.add(o); }
public E pop() { E o = list.get(getSize() - 1); list.remove(getSize() - 1); return o; }
public boolean isEmpty() { return list.isEmpty(); }
public int compareTo(MyStack
public ArrayList
@Override public String toString() { return "stack: " + list.toString(); } }
MyQueue code:
import java.util.LinkedList;
public class MyQueue
public void enqueue(E e) { list.addLast(e); }
public E dequeue() { return list.removeFirst(); }
public int getSize() { return list.size(); }
@Override public String toString() { return "Queue: " + list.toString(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
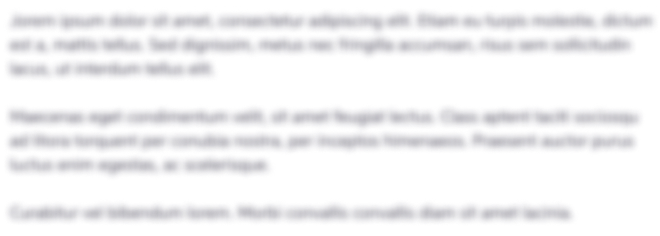
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started