Question
I need help with this one please. I need the validatePosInteger.hpp and .cpp, LineupPool.hpp and .cpp, LineupQueue.hpp and .cpp, LoserStack.hpp and .cpp. The other codes
I need help with this one please. I need the "validatePosInteger.hpp and .cpp", "LineupPool.hpp and .cpp", "LineupQueue.hpp and .cpp", "LoserStack.hpp and .cpp".
The other codes and criteria are below. In this C++ project, you will create a simple class hierarchy as the basis for a fantasy combat game. Your universe contains Vampires, Barbarians, Blue Men, Medusa and Harry Potter. Each has characteristics for attack, defense, armor, and strength points as follows. Type Attack Defense Armor Strength Points Vampire1 1d12 1d6* Charm 1 18 Barbarian2 2d6 2d6 0 12 Blue Men3 2d10 3d6 3 12 *Mob Medusa4 2d6* Glare 1d6 3 8 Harry Potter5 2d6 2d6 0 10/20*Hogwarts Suave, debonair, but vicious and surprisingly resilient! Think Conan or Hercules from the movies. Big sword, big muscles, bare torso. They are small (6 tall), fast and tough. So they are hard to hit and can take some damage. As for the attack value, you can do a LOT of damage when you can crawl inside the armor or clothing of your opponent. Scrawny lady with snakes for hair. They help with fighting. Just dont look at her! Why are you reading this? How can you not know who Harry Potter is? 3d6 is rolling three 6-sided dice, 2d10 is rolling two 10-sided dice, etc. *Charm: Vampires can charm an opponent into not attacking. For a given attack there is a 50% chance that their opponent does not actually attack them. *Glare: If a Medusa rolls a 12 in attack, then the target has looked her in the eyes and is turned to stone. The Medusa wins! If Medusa uses Glare on Harry Potter on his first life, then Harry Potter get back to life. If the Vampire's Charm ability activates versus Medusa's Glare, the Vampire's Charm trump the Glare. *Mob: The Blue Men are actually a swarm of small individuals. For every 4 points of damage (round down), they lose one defense die. For example, when they reach strength of 8 they only have 2d6 for defense. *Hogwarts: If Harry dies (i.e. strength <=0), he immediately recovers and his total strength becomes 20. If he were to die again, then hes dead. //-------------------------------------------------------------------- Goals Use linked structures to hold and manipulate data Modify an existing parent abstract class Project In this project, you will write a program to run a tournament. Start with your project 3 program for creatures. If you had issues with project 3 and need to begin fresh, reference the creature descriptions from project 3 to build your fighters. This is a one-user-two-players game. A user will enter the number of fighters both players will use. (You can limit the number of fighters in each team, but set it a little bit larger, like 10, or 20 to allow the enough testing.) The user should also enter the type of creatures and fighter names. You can give fighters different names. So player As first fighter could by Harry Potter and he could name the Harry Potter Creature "Larry Snotter" or just Harry Potter No.1. One team could have more than 1 of the same character (e.g. Team A has 3 members: 2 barbarians and 1 harry potter). The first player supplies a lineup of creatures in order. The second player then supplies his lineup of creatures in order. By lineup I mean something like a batting order in baseball or softball. The head of each lineup fight in the same way they fight in project 3. The winner gets put at the back of her/his teams lineup; the loser goes to the container for those who lost (there should be only one loser pile shared between both sides). The lineup order cannot be changed once the players are entered. Similarly, the loser pile should have the last loser at the top and the first loser at the bottom. Even if a creature wins, she/he may have taken damage. You should restore some percentage of the damage when they get back in line. Make this simple as you are adding a new member function to the parent class. Some examples are: generate a random integer in some ranges, e.g., on a roll of 5 they recover 50% of the damage they lost, or some scheme of your own. If you want to use a different recovery function for each character, that is fine. Remember to use polymorphism if the recovery is different for different creatures! After each round, you will display which type of creatures fought and which won on screen (For example, Round 1: Team A Blue man No.1 VS. Team B Harry Potter No.1, Harry Potter No.1 won!). At the end of the tournament (when one team or both run out of fighters in the lineup), your program will display final total points for each team (you can determine how to score each round of fight, for example, winner team +2, loser team -1, tie +1). You must display which team won the tournament. This is another element you must define in the analysis and design stage. You must have a method to determine the winner and you can determine it by yourself. Also provide an option at the end of the tournament to display the contents of the loser pile, i.e. print them out in order with the loser of the first round displayed last. You should be using polymorphism and all creatures will inherit from the Creature class. Each object should be instantiated and put into their lineup. You will only use pointers to creatures in your program. A creature pointer can then be used to indicate an object of a subclass and polymorphism will ensure the correct function is called. Ask your TA if you are uncertain what this means. NOTE: Depending upon your implementation in Project 3, you could have ties. How does that tie change your end of match logic? What do you do if the final match is a tie? Specifically, where do you put the fighters? For this program, you should use stack-like or queue-like structures to hold the lineups and the loser pile. Which will you use for the lineup? Which will you use for the loser pile? This is a programming assignment and not a complete game! Other than entering the lineup, the players just wait for the results. They should take no further actions. Make sure you test your program with instances of all creature types youve created. You may not be able to do an exhaustive test, but make it as extensive as you can. In the menu, please add an option that ask players if they would like to play againonce the winner has been declared (it's nice for grading since we run it multiple times). For this project, 4 please use your own containers like queue and linked list instead of the STL containers.
I have the following code:
//--------------------------------------------------------------------------------------------- Tournament.hpp /* Class: Tournament * Description: The Tournament class represents a round-based tournament * between two lineups of creatures (creatures are derived from the Creature * type). The class has two objects of type LineupQueue to hold each player's * lineup, and object of type LoserStack to hold the creatures who lose each * round, and an object of type LineupPool from which players choose their * lineup. To run a tournament, the member functions createLineups, * game, and printTournamentResults should be called in that order. */
#ifndef TOURNAMENT_HPP #define TOURNAMENT_HPP
#include
class Tournament { public: /* The constructor takes as a parameter a reference to a mt19937 * pseudo-random number generator object. This object is used by the * class to produce dice rolls. The constructor gets user input to * populate the lineupPool object with Creature objects. */ Tournament(std::mt19937& generator);
/* Function: createLineups * Description: This function prompts the user to choose creatures from * lineupPool to populate each lineup with (p1Lineup and p2Lineup). * Creature objects were dynamically allocated and are at first pointed to * by the nodes of lineupPool. When a user selects a creature from the pool to add * to his/her lineup, the pointer in lineupPool is set to nullptr and the lineup * points to the object instead. The function takes no parameters and returns * nothing. */ void createLineups();
/* Function: game * Description: The head of each player's lineup fight each other. * If a creature wins, it is returned to the back of its lineup and * regenerates some strength. If a creature loses, it is sent to the * Losers stack. Wins and losses for each player are tallied accordingly. * The function returns nothing and takes no parameters. */ void game();
/* Function: printTournamentResults * Description: Prints the lineup that won the tournament. It also prints * the final wins and losses for each player. It gives the user the option * to print the creatures that lost each round. The function takes no parameters * and returns nothing. */ void printTournamentResults();
//The destructor deletes lineupPool, p1Lineup, p2Lineupm and Losers. ~Tournament(); private: std::mt19937* generator; int lineupLength; //number of creatures in each lineup int p1Wins; int p1Losses; int p2Wins; int p2Losses; int currentRound; LineupPool* lineupPool; LineupQueue* p1Lineup; LineupQueue* p2Lineup; LoserStack* Losers;
/* Function: fight * Description: The function takes two * references to Creature objects that represent the fighters, as well as * a reference to a mersenne twister generator object. The function * takes turns calling the attack and defend functions for the fighters and * ultimately returns a pointer to the Creature that won the fight. */ Creature* fight(Creature& attacker, Creature& defender, std::mt19937& generator);
/* Function: printRound * Description: Prints the results of each round fought. Prints the * who fought whom, who won and who lost. It takes as parameters * Creature pointers created in the game function. It returns nothing. */ void printRound(Creature* winner, Creature* loser) const; };
#endif
//barbarian.hpp #ifndef BARBARIAN_hpp #define BARBARIAN_hpp #include
//---------------------------------------------------------------------------------------- //bluemen.hpp #ifndef BLUEMEN_hpp #define BLUEMEN_hpp #include
//----------------------------------------------------------------------------------------------- //bluemen.cpp //#include "bluemen.hpp" BlueMen::BlueMen(int arm, int sp, string type, string name): Creature(arm, sp, type, name) { //SETS INHERITED MEMBERS :) } int BlueMen::attack() { int damage = 0; damage += rand()%10 + 1; damage += rand()%10 + 1; return damage; } void BlueMen::defense(int damage) { int damageINF = 0; int defense = 0; defense = mob(s_Point); //mob method damage_INF = damage - defense - armor; if(BB) { cout << "Defense roll: " << defense < //----------------------------------------------------------------------------------------- //creature.hpp #ifndef CREATURE_hpp #define CREATURE_hpp #include //--------------------------------------------------------------------------- //creature.cpp //#include "creature.hpp" Creature::Creature() { } Creature::Creature(int armor, int sPoints, string cType, string name) { set_Armor(armor); set_s_Point(s_Point); set_Creature_Type(c_Type); set_Name(name); gold_Points = 0; } int Creature::get_Armor() { return armor; } int Creature::get_s_Point() { return s_Point; } int Creature::get_Gold_Point() { return gold_Point; } string Creature::get_Creature_Type() { return creature_Type; } string Creature::get_Name() { return name; } int Creature::get_Type() { return type; } bool Creature::get_BB() { return BB; } int Creature::get_team() { return team; } void Creature::set_Armor(int a){ armor = a; } void Creature::set_s_Point(int s) { s_Point = s; } void Creature::set_Gold_Point(int gp) { gold_Point += gp; } void Creature::setCreatureType(string ct) { creature_Type = ct; } void Creature::setName(string c_Name) { name = cName; } void Creature::set_Type(int t) { type = t; } void Creature::set_BB(bool b) { BB = b; } void Creature::set_team(int t) { team = t; } void Creature::roll_Gold() { int gold; gold = rand()%5 + 1; set_Gold_Point(gold); } void Creature::restore_Point() { int point_Restored; point_Restored = get_s_Point() + (((rand()%5 + 1)/10)*get_s_Point()); if(point_s_Restored < 0) { point_s_Restored = point_s_Restored * -1; } set_s_Points(point_s_Restored); } //------------------------------------------------------------------------------------------- //main.cpp #include this->Losers = new LoserStack; this->lineupPool = new LineupPool; this->p1Lineup = new LineupQueue; this->p2Lineup = new LineupQueue; bool continueAddingCreatures = true; int numInPool = 0; std::string name; std::cout << " * Ok, each lineup will have " << lineupLength << " creature(s) in it. "; std::cout << "* You will now fill the pool with creatures. "; std::cout << "* The pool needs at least as many creatures"; std::cout << " as the number of creatures in both lineups. "; do { std::cout << "********************************************************* "; std::cout << "* Choose the type of creature to add to the lineup pool. "; std::cout << "* (Select option 6 when you are done adding to the pool). "; std::cout << "1. Barbarian 2. Blue Men 3. Harry Potter 4. Medusa "; std::cout << "5. Vampire 6. Done adding creatures. "; std::cout << "********************************************************* "; int option = 0; while (option < 1 || option > 6) { option = validatePosInteger(); if (option < 1 || option > 6) { std::cout << "* Enter one of the options above. "; } } if (option >= 1 && option < 6) { do { std::cout << "* Enter a name for this creature: "; std::getline(std::cin, name); } while (name.empty()); } switch (option) { case 1: this->lineupPool->addNode(BARBARIAN, name); ++numInPool; break; case 2: this->lineupPool->addNode(BLUE_MEN, name); ++numInPool; break; case 3: this->lineupPool->addNode(HARRY_POTTER, name); ++numInPool; break; case 4: this->lineupPool->addNode(MEDUSA, name); ++numInPool; break; case 5: this->lineupPool->addNode(VAMPIRE, name); ++numInPool; break; case 6: if (numInPool < lineupLength * 2) { std::cout << "* There are not enough creatures in the pool to fill both lineups. "; std::cout << " * Please enter at least " << (lineupLength * 2) - numInPool << " more. "; continueAddingCreatures = true; } else { continueAddingCreatures = false; } break; } if(option != 6) { std::cout << "* " << name << " was added to the lineup pool. "; } } while (continueAddingCreatures); } void Tournament::createLineups() { std::cout << " ******************************************* "; std::cout << "* Tournament Player Lineup Creation Phase * "; std::cout << "******************************************* "; std::cout << "* Player 1 will now choose their lineup from the pool of available creatures. "; for (int i = 0; i < this->lineupLength; ++i) { std::string creatureName; std::cout << " * Enter the name of the creature you want to add to your team"; std::cout << " from the creatures below: "; this->lineupPool->printPool(); Creature* searchResults; do { std::getline(std::cin, creatureName); searchResults = this->lineupPool->searchPool(creatureName); if (searchResults == nullptr) { std::cout << "* There is no creature with that name. "; std::cout << "Enter another name. "; } } while (searchResults == nullptr); this->p1Lineup->enqueue(searchResults, "P1"); } std::cout << " * Player 2 will now choose their lineup from the pool of available creatures. "; for (int i = 0; i < this->lineupLength; ++i) { std::string creatureName; std::cout << "* Enter the name of the creature you want to add to your team"; std::cout << " from the creatures below: "; this->lineupPool->printPool(); Creature* searchResults; do { std::getline(std::cin, creatureName); searchResults = this->lineupPool->searchPool(creatureName); if (searchResults == nullptr) { std::cout << "* There is no creature with that name. "; std::cout << "Enter another name. "; } } while (searchResults == nullptr); this->p2Lineup->enqueue(searchResults, "P2"); } } void Tournament::game() { std::cout << " * Show updated score tally after each round? (y/n). "; char showRound; do { std::cin >> showRound; std::cin.clear(); std::cin.ignore(std::numeric_limits while (!this->p1Lineup->isEmpty() && !this->p2Lineup->isEmpty()) { ++this->currentRound; Creature* p1Fighter = this->p1Lineup->getFront(); Creature* p2Fighter = this->p2Lineup->getFront(); Creature* loser = nullptr; Creature* winner = this->fight(*p1Fighter, *p2Fighter, *this->generator); if (winner == p1Fighter) { loser = p2Fighter; } else { loser = p1Fighter; } this->printRound(winner, loser); if (winner->getPlayer() == "P1") { ++this->p1Wins; ++this->p2Losses; this->p1Lineup->frontToBack(); //front to back calls healing function this->p2Lineup->dequeue(); this->Losers->pushBack(loser); } else { ++this->p2Wins; ++this->p1Losses; this->p2Lineup->frontToBack(); this->p1Lineup->dequeue(); this->Losers->pushBack(loser); } if (showRound == 'y') { std::cout << "* Round " << currentRound << " winner: " << winner->getName(); std::cout << " * P1 Wins: " << p1Wins; std::cout << ". * P1 Losses: " << p1Losses; std::cout << ". * P2 Wins: " << p2Wins; std::cout << ". * P2 Losses: " << p2Losses << ". "; } } } void Tournament::printTournamentResults() { std::cout << " **************************** "; std::cout << "* TOURNAMENT RESULTS * "; std::cout << "**************************** "; if (this->p1Wins > this->p2Wins) { std::cout << "* Player 1's lineup won the tournament. "; } else { std::cout << "* Player 2's lineup won the tournament. "; } std::cout << "* The final tally of points is as follows: * "; std::cout << "******************************************** "; std::cout << "* P1 wins: " << this->p1Wins << ". "; std::cout << "* P1 losses: " << this->p1Losses << ". "; std::cout << "* P2 wins: " << this->p2Wins << ". "; std::cout << "* P2 losses: " << this->p2Losses << ". "; std::cout << "******************************************** "; std::cout << "* Would you like to see the defeated creatures? (y/n) "; char seeLosers; do { std::cin >> seeLosers; std::cin.clear(); std::cin.ignore(std::numeric_limits } while (seeLosers != 'y' && seeLosers != 'n'); if (seeLosers == 'y') { this->Losers->printStack(); } } Creature* Tournament::fight(Creature& attacker, Creature& defender, std::mt19937& generator) { //simulate a coin flip (or roll of 2-sided die) to choose who will attack first int roll = Die(1, 2).rollDie(generator); int turn; if (roll == 1) { turn = 0; } else { turn = 1; } int defenderStr = defender.getStrength(), attackerStr = attacker.getStrength(); int atk = 0; //have fighters take turn attacking and defending do { if (turn % 2 == 0) { atk = attacker.attack(defender, generator); defenderStr = defender.defend(atk, attacker, generator); ++turn; } else { atk = defender.attack(attacker, generator); attackerStr = attacker.defend(atk, defender, generator); ++turn; } } while (defenderStr > 0 && attackerStr > 0); //return winner if (attacker.getStrength() <= 0) { return &defender; } else { return &attacker; } } void Tournament::printRound(Creature* winner, Creature* loser) const { std::cout << " * Round " << this->currentRound << " Results * "; std::cout << "********************************************** "; std::cout << "* " << loser->getPlayer() << "\'s " << loser->getType() << ", " << loser->getName(); std::cout << ", fought " << winner->getPlayer() << "\'s " << winner->getType() << ", " << winner->getName(); std::cout << ". " << winner->getName() << " won the round. "; } Tournament::~Tournament() { delete this->Losers; delete this->lineupPool; delete this->p1Lineup; delete this->p2Lineup; } //------------------------------------------------------------------------------------------ HarryPotter.cpp //This is the implementation for the HarryPotter class. //Please see the corresponding header file for details about how to use it. #include "HarryPotter.hpp" #include "Die.hpp" #include HarryPotter::HarryPotter(std::string name) { this->name = name; this->numDeaths = 0; this->type = "Harry Potter"; this->armor = 0; this->maxStrength = 10; this->strength = 10; this->defDieSides = 6; this->numDefDie = 2; this->atkDieSides = 6; this->numAtkDie = 2; this->attackPrevented = false; //changes during fighting this->defenseCircumvented = false; //changes during fighting } HarryPotter::SecondWindResult HarryPotter::secondWind() { if (this->strength <= 0 && this->numDeaths == 0) { ++this->numDeaths; this->strength = 20; return SECOND_WIND; } else { return DEAD; } } int HarryPotter::defend(int atk, Creature& attacker, std::mt19937& generator) { int dmg{ 0 }; if (attacker.getAttackPrevented()) { attacker.setAttackPrevented(false); dmg = 0; } else if (this->defenseCircumvented) { this->setDefenseCircumvented(false); this->strength = 0; } else { dmg = atk - Die(this->numDefDie, this->defDieSides).rollDie(generator) - this->armor; if (dmg >= 0) { this->strength -= dmg; } } secondWind(); return this->strength; } HarryPotter.hpp /* * This is the header file for the class HarryPotter. HarryPotter inherits * publicly from the Creature class, but has several specialized functions. * If a HarryPotter object is defeated once, it can come back to life via the * secondWind function. */ #ifndef HARRY_POTTER_HPP #define HARRY_POTTER_HPP #include "Creature.hpp" class HarryPotter : public Creature { public: //enum for debugging secondWind() enum SecondWindResult{SECOND_WIND, DEAD}; //default constructor HarryPotter(std::string name); /* * The defend function is very similar to the parent function * it overrides. It differs in that it calls the secondWind function * defined below after all logic of the parent function has been * moved through. If the HarryPotter object's strength reaches * 0 for the first time, its strength is set to 20. Like its parent * function, it takes an integer that represents damage dealt by the * attacker, a reference to a Creature object that represents the attacker * being defended against, and a reference to a mersenne twister generator * object. */ int defend(int atk, Creature& attacker, std::mt19937 & generator); protected: int numDeaths; /* * The function secondWind is meant to be called inside a HarryPotter * object's defend function at the end of the function. The function * checks the object's strength and the numDeaths variable. If numDeaths * is 0 and the object's strength is less than 0, then numDeaths is * incremented and the object's strength is set to 20. */ SecondWindResult secondWind(); }; #endif Medusa.cpp /This is the implementation for the Medusa class. //Please see the corresponding header file for details about how to use it. #include "Medusa.hpp" #include "Die.hpp" Medusa::Medusa(std::string name) { this->name = name; this->type = "Medusa"; this->armor = 3; this->maxStrength = 8; this->strength = 8; this->defDieSides = 6; this->numDefDie = 1; this->atkDieSides = 6; this->numAtkDie = 2; this->attackPrevented = false; //changes during fighting this->defenseCircumvented = false; //changes during fighting } int Medusa::attack(Creature& defender, std::mt19937 & generator) { int atk{ Die(this->numAtkDie, this->atkDieSides).rollDie(generator) }; if (atk == 12) { defender.setDefenseCircumvented(true); } return atk; } Medusa.hpp /* * This is the head file for the Medusa class, which inherits publicly * from the Creature class and differs only in its constructor and attack * function */ #ifndef MEDUSA_HPP #define MEDUSA_HPP #include "Creature.hpp" class Medusa : public Creature { public: //default constructor Medusa(std::string name); /* * The attack function overrides the attack function from the Creature * parent class. It differs in that if the function rolls a 12, the * defender's defenseCircumvented variable is set to true. Then, the next * time the defender calls defend during a fight, its strength is set to 0 * unless the attacker's attackPrevented variable is also set to true. */ virtual int attack(Creature& defender, std::mt19937& generator); }; #endif //-----------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
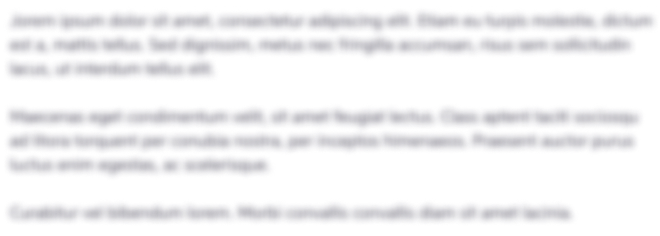
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started