Question
I need help with this programming assignment. It is to be done in Python programming language. P.S. Please adhere to all of the detailed requirements
I need help with this programming assignment. It is to be done in Python programming language.
P.S. Please adhere to all of the detailed requirements and instructions. Your help will be most appreciated. Thanks
So one of the previous responses to this question did not adhere to the requirements which was to create the seperate code three distinct python files XXmain.py, XXtriangles.py & XXpoints.py.
PLEASE WRITE THE CODE AS THREE DISTINCT PROGRAMS; WITH THE TRIANGLES AND POINTS INPUTTED INTO THE MAIN PROGRAM. THANKS!
Essentially the XXpoints.py will create a point object when given 2 values. These will be stored in the attributes named x and y. There will be two methods: getX and getY. There will be no setters; points will only be created with a constructor that receives an x and a y value.
and the XXtriangles.py will create a triangle object when given 3 point objects. Its attributes will be these three points, which can be retrieved with the getter getVertices, which will return a list of three points. It will also, within the constructor, determine the three side lengths and store those as side1, side2, and side3. (This means that the method sideLength can be in the constructor.) These values can be retrieved with the getter getSides, which will return a list of the three floats.
XXpoints.py and XXtriangles needs to implemented in the XXmain.py to produce the final output XXoutput6.txt from the input file input6.txt.
Also please use the value of epsilon in the file to be 0.0001 when evaluating features (VERY IMPORTANT)
...
Here is the previous response or code that you can modify to better suit the requirements detailed above. Thanks a lot. Also it is not necesarry that you use the code since I haven't checked it for errors.
import math
def sideLength(x1,y1,x2,y2):
return math.sqrt((x1-x2)**2+(y1-y2)**2)
def duplicatePts(x1,y1,x2,y2,x3,y3):
if( ((x1==x2)and(y1==y2)) or ((x1==x3)and(y1==y3)) or ((x2==x3)and(y2==y3))):
return True
else:
return False
def collinear(x1,y1,x2,y2,x3,y3):
if((x1*(y2-y3)+x2*(y3-y1)+x3*(y1-y2))==0):
return True
else:
return False
def findShortest(x1,y1,x2,y2,x3,y3):
l1 = sideLength(x1,y1,x2,y2)
l2 = sideLength(x1,y1,x3,y3)
l3 = sideLength(x2,y2,x3,y3)
return min(l1,l2,l3)
def perimeter(x1,y1,x2,y2,x3,y3):
l1 = sideLength(x1,y1,x2,y2)
l2 = sideLength(x1,y1,x3,y3)
l3 = sideLength(x2,y2,x3,y3)
return l1+l2+l3
def area(x1,y1,x2,y2,x3,y3):
return (x1*(y2-y3)+x2*(y3-y1)+x3*(y1-y2))*0.5
def right(x1,y1,x2,y2,x3,y3):
l1 = sideLength(x1,y1,x2,y2)
l2 = sideLength(x1,y1,x3,y3)
l3 = sideLength(x2,y2,x3,y3)
if((l1*l1==l2*l2+l3*l3) or (l2*l2==l1*l1+l3*l3) or (l3*l3==l2*l2+l1*l1) ):
return True
else:
return False
def acute(x1,y1,x2,y2,x3,y3):
l1 = sideLength(x1,y1,x2,y2)
l2 = sideLength(x1,y1,x3,y3)
l3 = sideLength(x2,y2,x3,y3)
if((l1*l1 return True else: return False def obtuse(x1,y1,x2,y2,x3,y3): l1 = sideLength(x1,y1,x2,y2) l2 = sideLength(x1,y1,x3,y3) l3 = sideLength(x2,y2,x3,y3) if((l1*l1>l2*l2+l3*l3) or (l2*l2>l1*l1+l3*l3) or (l3*l3>l2*l2+l1*l1) ): return True else: return False def scalene(x1,y1,x2,y2,x3,y3): l1 = sideLength(x1,y1,x2,y2) l2 = sideLength(x1,y1,x3,y3) l3 = sideLength(x2,y2,x3,y3) if(l1!=l2 and l2!=l3 and l3!=l1): return True else: return False def isosceles(x1,y1,x2,y2,x3,y3): l1 = sideLength(x1,y1,x2,y2) l2 = sideLength(x1,y1,x3,y3) l3 = sideLength(x2,y2,x3,y3) if(l1==l2 or l2==l3 or l3==l1): return True else: return False def equilateral(x1,y1,x2,y2,x3,y3): l1 = sideLength(x1,y1,x2,y2) l2 = sideLength(x1,y1,x3,y3) l3 = sideLength(x2,y2,x3,y3) if(l1==l2 and l2==l3): return True else: return False def main(): f = open('input4.txt', 'r') for line in f: coord = [] for word in line.split(): coord.append(float(word)) if(duplicatePts(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5])): print("Duplicate Points") elif(collinear(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5])): print("Points are collinear") else: minside = findShortest(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5]) per = perimeter(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5]) are = area(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5]) print('Vertices is: ({},{}),({},{}),({},{})\t Shortest side is {}'.format(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5],round(minside,2))) print('Perimeter is: {}\t Area is {}'.format(round(per,2),round(are,2))) if(equilateral(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5])): print("Equilateral",end='\t') elif(isosceles(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5])): print("Isosceles",end='\t') else: print("Scalene", end = '\t') if(right(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5])): print("Right",end=' ') elif(obtuse(coord[0],coord[1],coord[2],coord[3],coord[4],coord[5])): print("Obtuse",end=' ') else: print("Acute", end = ' ')
Step by Step Solution
There are 3 Steps involved in it
Step: 1
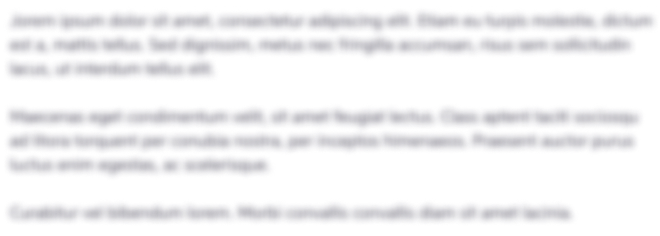
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started