Question
I need help with why my code doesn't work. If someone could type my code it and resolve it from there that would be great.
I need help with why my code doesn't work. If someone could type my code it and resolve it from there that would be great. Send back the final product along with the output. Thank you.
I have 5 classes and 1 interface: (each section separated with "=")
==========================
================
===========
import java.util.Arrays;
public class ArrayBag
public ArrayBag() { this(DEFAULT_CAPACITY); }
@SuppressWarnings("unchecked") public ArrayBag(int initialCapacity) { numberOfEntries = 0; list = (E[]) new Object[initialCapacity]; }
public int getCurrentSize() { return numberOfEntries; }
@Override public boolean isEmpty() { return numberOfEntries == 0; }
@Override public boolean add(E newEntry) { if (numberOfEntries == list.length) { list = Arrays.copyOf(list, 2 * list.length); } list[numberOfEntries++] = newEntry; return true; }
@Override public E remove() { E removedEntry = null; if (numberOfEntries > 0) { numberOfEntries--; removedEntry = list[numberOfEntries]; list[numberOfEntries] = null; } return removedEntry; }
@Override public boolean remove(E anEntry) { int index = getIndexOf(anEntry); E removed = removeEntry(index); return removed != null; }
private E removeEntry(int index) { E removed = null; if (!isEmpty() && index >= 0) { removed = list[index]; numberOfEntries--; list[index] = list[numberOfEntries]; list[numberOfEntries] = null; } return removed; }
public int getIndexOf(E anEntry) { int index = -1; for (int i = 0; i
@Override public void clear() { while (!isEmpty()) { remove(); } }
@Override public int getFrequencyOf(E anEntry) { int frequency = 0; for (int i = 0; i
@Override public boolean contains(E anEntry) { return getIndexOf(anEntry) > -1; }
public E[] toArray() { return Arrays.copyOf(list, numberOfEntries); } }
==========
import java.util.Random;
public class LinkedBag
public LinkedBag() { list = new SinglyLinkedList
public LinkedBag(int initialCapacity) { this(); }
@Override public int getCurrentSize() { return list.size(); }
@Override public boolean isEmpty() { return list.isEmpty(); }
@Override public boolean add(E newEntry) { return list.add(newEntry); }
@Override public E remove() { if (isEmpty()) { return null; } else { Random rand = new Random(); int index = rand.nextInt(getCurrentSize()); return list.remove(index); } }
@Override public boolean remove(E anEntry) { return list.remove(anEntry); }
@Override public void clear() { list.clear(); }
@Override public int getFrequencyOf(E anEntry) { int frequency = 0; for (int i = 0; i
@Override public boolean contains(E anEntry) { return list.contains(anEntry); }
@Override public E[] toArray() { return list.toArray(); } }
=========
import java.util.Random;
public class Client { public static void main(String[] args) { // Create an instance of ArrayBag for men's football team ArrayBag mensTeam = new ArrayBag();
// Add 8 players to the men's team mensTeam.add(new Player("John Doe", "Quarterback", 22)); mensTeam.add(new Player("Jane Doe", "Running Back", 21)); mensTeam.add(new Player("Jim Smith", "Wide Receiver", 24)); mensTeam.add(new Player("Sarah Johnson", "Tight End", 20)); mensTeam.add(new Player("Mark Davis", "Offensive Lineman", 25)); mensTeam.add(new Player("Laura Wilson", "Defensive Back", 23)); mensTeam.add(new Player("Andrew Lee", "Linebacker", 26)); mensTeam.add(new Player("Emily Brown", "Defensive Lineman", 22));
// Display the contents of the men's team System.out.println("Men's football team: "); System.out.println(mensTeam);
// Remove a random player from the men's team Random rand = new Random(); int index = rand.nextInt(mensTeam.getCurrentSize()); boolean removedPlayer = mensTeam.remove(index); System.out.println("Removed player: " + removedPlayer);
// Display the contents of the men's team after removing a player System.out.println("Men's football team after removing a player: "); System.out.println(mensTeam);
// Get (but do not remove) a reference to the 5th item in the bag Player fifthPlayer = (Player) mensTeam.get(4); System.out.println("5th player in the team: " + fifthPlayer);
// Add another player mensTeam.add(new Player("Michael Jackson", "Running Back", 23)); System.out.println("Men's football team after adding another player: "); System.out.println(mensTeam);
// Remove the 5th player mensTeam.remove(fifthPlayer); System.out.println("Men's football team after removing the 5th player: "); System.out.println(mensTeam);
// Create an instance of ArrayBag for courses ArrayBag courses = new ArrayBag();
// Add courses to the bag courses.add("CSci 161"); courses.add("CSci 162"); courses.add("CSci 175"); courses.add("CSci 180");
// Display the contents of the courses bag System.out.println("Courses: "); System.out.println(courses);
// Remove a random course index = rand.nextInt(courses.getCurrentSize()); boolean removedCourse = courses.remove(index); System.out.println("Removed course: " + removedCourse);
// Display the contents of the courses bag after removing a course System.out.println("Courses after removing a course: "); System.out.println(courses); LinkedBag womensTeam = new LinkedBag(); // add eight players to womensTeam womensTeam.add(new Player("John Doe ", "PG", 22)); womensTeam.add(new Player("John Doe ", "PG", 22)); womensTeam.add(new Player("John Doe ", "PG", 22)); womensTeam.add(new Player("John Doe ", "PG", 22)); womensTeam.add(new Player("John Doe ", "PG", 22)); womensTeam.add(new Player("John Doe ", "PG", 22)); womensTeam.add(new Player("John Doe ", "PG", 22)); womensTeam.add(new Player("John Doe ", "PG", 22)); System.out.println("Women's football team: "); System.out.println(womensTeam); } }
// Based on Code Fragments 3.14&3.15 from textbook "Data Structures and Algorithm Analysis in Java" by Mark Allen Weiss \} // Bag interface for Lablo3 assignm // Based on the Lablo2 assignment public interface Bag E> Transcribed by [Your Name] int size (); boolean isEmpty(); void clear(); int getFrequencyOf (E e); boolean contains (E e); void add (E e); E remove (E e); E remove (); E get (int i); String tostring(); boolean equals (Object o)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
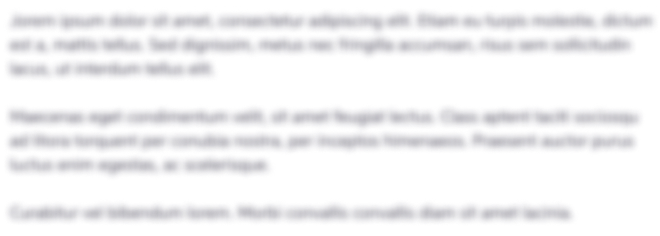
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started