Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I NEED IT IN JAVA The main idea of this assignment is to work with classes and methods. You will create classes and methods to










I NEED IT IN JAVA
The main idea of this assignment is to work with classes and methods. You will create classes and methods to complete a dice game. In this game, we will use dices with 6 faces. In each round, two dices will be rolled and each player will bet on the outcome. Prep Work 1. Look at the UML class diagram below. It describes three different classes that work together, along with the fields and methods that are in each class. 2. Create a new Java project in NetBeans. The project name must follow this format: LastnameFirstnameAssignment1. So, if Professor Cao was doing the assignment, the project would be named: CaoLijuanAssignment1. Make sure to uncheck the "Create Main Class" checkbox before creating the project. Part 1: Player class (10%) 1. Add a new Java class to your project. Name your new class Player. This class stores information for the game players. 2. Add fields to your newly created class according to the UML class diagram. Initialize INITIAL_BALANCE to 500. players. 2. Add fields to your newly created class according to the UML class diagram. Initialize INITIAL_BALANCE to 500. 3. Add and implement the constructor. The constructor will initialize the name field and initialize balance to the INITIAL_BALANCE. 4. Add and implement the getters and the setters. Part 2: DiceGame class (40%) 1. Add a new Java class to your project. Name this class DiceGame. This class handles all of the game logistics. 2. Add fields to your newly created class according to the UML class diagram. 3. Add a constructor. In the constructor, call setUpGame method. 4. Add and implement the getters. 5. Add the method stub for the rest of the methods in this class. 6. Now add code to the setUpGame method. In this method, 1) ask how many people are going to play. Use the scanner's nextlnt() method to get the number they enter. Assign the input to the numOfPlayers field; 2) create an array of Player objects and assign that to the players field; 3) ask for each player's name and use that to create a new Player object with that name and add that Player object to the array of players; 4) call the displayRules() method; 7. Implement displayRules method. This method displays the follow message when it is called. RULES Each player player places a bet and cheoses a nuber betveen 2 and 12. The total of all the bets forms a "pot". Then twe dices are relled. If one of the players bet on the result cerrectly, she er he wins the entire pot. If more than one player bet on that nunber, the one whe bet the mest wins the entire pot. If there is a tie, theye If nobody bet en that the money renains in the pet fer the sext raund. ayers; 4) call the displayRules() method; 7. Implement displayRules method. This method displays the follow message when it is called. RULES Each player player places a bet and chooses a nuber beteen 2 and 12. The total of ali the bets forms a "pot". Then twe dices are rolled. If one of the players bet on the result correctly, she or he wins the entire pat. If more than one player bet on that number, the one who bet the most wins the entire pot. there is a tie, they split the pot. If nobody bet on that number, the noney remains in the pet for the next round. The game is over if one of the players run out of noney. 8. Implement playGame method. In this method, 1) loop through each player in the array, call the playTurn method with each player. Further, accumulate each player's bet amount to the pot; 2) display the balance in the pot; 3) randomly generate two integers between 1 and 6 to simulate the outcome of rolling two dices. Add these two integers together and assign the value to a local variable named outcome; 4) call checkWinner(outcome); 5) loop through each player to check if any player's balance is 0. Set gameOver to true if any player's balance is 0. 9. Implement playTurn method. In this method: 1) Tell the player his or her balance, and ask him or her to enter the amount to bet. If the amount exceeds the balance, output "Bet balance exceeds available funds" and ask the player to enter the amount of the bet. Repeat until the player's bet is less or equal to the bet balance. Assign the input to the player's betAmount field. Update the player's balance by subtracting the betAmount. 2) ask the player to enter his or her guess. Assign the input to his or her guess field. Your program should not accept the input if it is not in the range of 2 and 12. 10. Implement checkWinner method. In this method, you will find out if any player correctly guessed the outcome and update each player's balance and the balance of the pot accordingly. Here are the rules you apply: if no one made the correct guess, all of the players bets will be kept in the pot. If one player made the correct guess, he or she wins everything in the pot. If more than one player made the correct guess, the player withigh in werything in the input to his or her guess field. Your program should not accept the input if it is not in the range of 2 and 12. 10. Implement checkWinner method. In this method, you will find out if any player correctly guessed the outcome and update each player's balance and the balance of the pot accordingly. Here are the rules you apply: if no one made the correct guess, all of the players bets will be kept in the pot. If one player made the correct guess, he or she wins everything in the pot. If more than one player made the correct guess, the player with the highest bet wins everything in the pot; if multiple winning players have the highest bet, they will evenly split the balance in the pot. This method should also display how much each player won or lost in this round. Part 3: Test your project (0%) 1. Add a new Java class to your project. Name your new class Start. This class will start your game. 2. Copy paste the following lines to your main method: System.out.println("Welcome to the Dice Game!"); DiceGame dg = new DiceGame(); int round = 1; while(!dg.isGameOver() { System.out.println("********************** System.out.println(" ROUND" + round); System.out.println("***** System.out.println(); dg.playGame(); round++; System.out.println("Game is over. Everyone's balance is as below." players. 2. Add fields to your newly created class according to the UML class diagram. Initialize INITIAL_BALANCE to 500. 3. Add and implement the constructor. The constructor will initialize the name field and initialize balance to the INITIAL_BALANCE. 4. Add and implement the getters and the setters. Part 2: DiceGame class (40%) 1. Add a new Java class to your project. Name this class DiceGame. This class handles all of the game logistics. 2. Add fields to your newly created class according to the UML class diagram. 3. Add a constructor. In the constructor, call setUpGame method. 4. Add and implement the getters. 5. Add the method stub for the rest of the methods in this class. 6. Now add code to the setUpGame method. In this method, 1) ask how many people are going to play. Use the scanner's nextlnt() method to get the number they enter. Assign the input to the numOfPlayers field; 2) create an array of Player objects and assign that to the players field; 3) ask for each player's name and use that to create a new Player object with that name and add that Player object to the array of players; 4) call the displayRules() method; 7. Implement displayRules method. This method displays the follow message when it is called. RULES Each player player places a bet and cheoses a nuber betveen 2 and 12. The total of all the bets forms a "pot". Then twe dices are relled. If one of the players bet on the result cerrectly, she er he wins the entire pot. If more than one player bet on that nunber, the one whe bet the mest wins the entire pot. If there is a tie, theye If nobody bet en that the money renains in the pet fer the sext raund. input to his or her guess field. Your program should not accept the input if it is not in the range of 2 and 12. 10. Implement checkWinner method. In this method, you will find out if any player correctly guessed the outcome and update each player's balance and the balance of the pot accordingly. Here are the rules you apply: if no one made the correct guess, all of the players bets will be kept in the pot. If one player made the correct guess, he or she wins everything in the pot. If more than one player made the correct guess, the player with the highest bet wins everything in the pot; if multiple winning players have the highest bet, they will evenly split the balance in the pot. This method should also display how much each player won or lost in this round. Part 3: Test your project (0%) 1. Add a new Java class to your project. Name your new class Start. This class will start your game. 2. Copy paste the following lines to your main method: System.out.println("Welcome to the Dice Game!"); DiceGame dg = new DiceGame(); int round = 1; while(!dg.isGameOver()) { System.out.printin(" System.out.printin(" ROUND"+ round); .****"); System.out.println("****** System.out.println(0: ")B dg.playGame(); round++; } System.out.println("Game is over. Everyone's balance is as bel input to his or her guess field. Your program should not accept the input if it is not in the range of 2 and 12. 10. Implement checkWinner method. In this method, you will find out if any player correctly guessed the outcome and update each player's balance and the balance of the pot accordingly. Here are the rules you apply: if no one made the correct guess, all of the players bets will be kept in the pot. If one player made the correct guess, he or she wins everything in the pot. If more than one player made the correct guess, the player with the highest bet wins everything in the pot; if multiple winning players have the highest bet, they will evenly split the balance in the pot. This method should also display how much each player won or lost in this round. Part 3: Test your project (0%) 1. Add a new Java class to your project. Name your new class Start. This class will start your game. 2. Copy paste the following lines to your main method: System.out.println("Welcome to the Dice Game!"); DiceGame dg = new DiceGame(); int round = 1; while(!dg.isGameOver() { System.out.println("********************** System.out.println(" ROUND" + round); System.out.println("***** System.out.println(); dg.playGame(); round++; System.out.println("Game is over. Everyone's balance is as below." round++; } System.out.println("Game is over. Everyone's balance is as below: "); for(int i = 0; i Step by Step Solution
There are 3 Steps involved in it
Step: 1
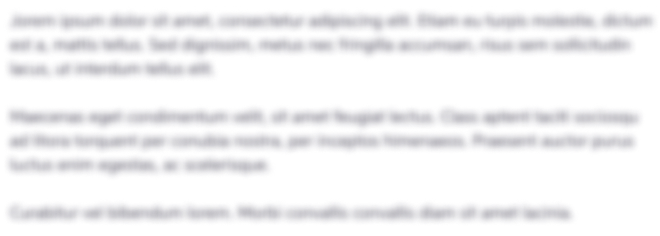
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started