Question
I need little bit of help this code I am not sure if the custom methods are correct or not because it is not adding
I need little bit of help this code I am not sure if the custom methods are correct or not because it is not adding the recipe names to the arraylist listOfRecipes ...I am using the main method to print a menu for the user to toggle between the menu. 1 = Add Recipe 2= PrintAllRecipeDetails 3= PrintAllRecipeNames... I have added the recipe1() method because it was giving me error that unable to find the method... If anyone can help me with the code I would appreciate it.
package SteppingStones;
import java.util.ArrayList;
import java.util.Scanner;
/**
*
* @author
*/
public class SteppingStone6_RecipeBox {
//Declaring instance variables private ArrayList of the type SteppingStone5_Recipe
private ArrayList
//Accessors and Mutators for listOfRecipes
/**
* @return the listOfRecipes
*/
public ArrayList
return listOfRecipes;
}
/**
* @param listOfRecipes the listOfRecipes to set
*/
public void setListOfRecipes(ArrayList
this.listOfRecipes = listOfRecipes;
}
/**
* @param listOfRecipes
**/
//Contstructors fo SteppingStone6_RecipeBox()
public SteppingStone6_RecipeBox(ArrayList
this.listOfRecipes = listOfRecipes;
}
public SteppingStone6_RecipeBox() {
this.listOfRecipes = new ArrayList<>();
}
//Custom method to printAllRecipeDetails
void printAllRecipeDetails(String selectedRecipe){
for (SteppingStone5_Recipe recipe : listOfRecipes) {
if(recipe.getRecipeName().equalsIgnoreCase(selectedRecipe)) {
recipe.printRecipe();
return;
}
}
System.out.println("No Recipe found with name: " + selectedRecipe);
}
//Custom method for PrintAllRecipeNames
void printAllRecipeNames(){
for (SteppingStone5_Recipe selectedRecipe : listOfRecipes) {
System.out.println(selectedRecipe.getRecipeName());
}
}
//Custom method to AddRecipe
public void addRecipe(SteppingStone5_Recipe tempRecipe) {
SteppingStone5_Recipe.addRecipe();
listOfRecipes.add(tempRecipe);
}
/**
* A variation of following menu method should be used as the actual main
* once you are ready to submit your final application. For this
* submission and for using it to do stand-alone tests, replace the
* public void menu() with the standard
* public static main(String[] args)
* method
*/
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
// Create a Recipe Box
SteppingStone6_RecipeBox myRecipeBox = new SteppingStone6_RecipeBox();
Scanner menuScnr = new Scanner(System.in);
//Printing mennu for user to select the available options
System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:");
while (menuScnr.hasNextInt() || menuScnr.hasNextLine()) {
System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:");
int input = menuScnr.nextInt();
/**
* The code below has two variations:
* 1. Code used with the SteppingStone6_RecipeBox_tester.
* 2. Code used with the public static main() method
*
* One of the sections should be commented out depending on the use.
*/
/**
* This could should remain uncommented when using the
* SteppingStone6_RecipeBox_tester.
*
* Comment out this section when using the
* public static main() method
*/
/** if (input == 1) {
recipe1();
} else if (input == 2) {
System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
printAllRecipeDetails(selectedRecipeName);
} else if (input == 3) {
for (int j = 0; j < listOfRecipes.size(); j++) {
System.out.println((j + 1) + ": " + listOfRecipes.get(j).getRecipeName());
}
} else {
System.out.println(" Menu " + "1. Add Recipe " + "2. Print Recipe " + "3. Adjust Recipe Servings " + " Please select a menu item:");
}
**/
/**
* This could should be uncommented when using the
* public static main() method
*
* Comment out this section when using the
* SteppingStone6_RecipeBox_tester.
*
**/
//Using if-else to select three custom methods.
if (input == 1) {
myRecipeBox.recipe1();
} else if (input == 2) {
System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
myRecipeBox.printAllRecipeDetails(selectedRecipeName);
} else if (input == 3) {
for (int j = 0; j < myRecipeBox.listOfRecipes.size(); j++) {
System.out.println((j + 1) + ": " + myRecipeBox.listOfRecipes.get(j).getRecipeName());
}
} else {
System.out.println(" Menu " + "1. Add Recipe " + "2. Print Recipe " + "3. Adjust Recipe Servings " + " Please select a menu item:");
}
System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:");
}
}
public void recipe1() {
double totalRecipeCalories = 0.0;
ArrayList
boolean addMoreIngredients = true;
Scanner scnr = new Scanner(System.in);
System.out.println("Please enter the recipe name: "); //prompt user to enter recipe name.
String recipeName = scnr.nextLine(); //checks if valid input was entered.
System.out.println("Please enter the number of servings: "); //prompt user to enter number of servings for recipe.
int servings = scnr.nextInt(); //checks if valid input was entered.
do {
//prompt user to enter ingredients or type end to finish loop.
System.out.println("Please enter the ingredient name or type end if you are finished entering ingredients: ");
String ingredientName = scnr.next().toLowerCase();
//if user type end loop will end and print the recipe with ingredient, serving and calories.
if (ingredientName.toLowerCase().equals("end")) {
addMoreIngredients = false;
}
else {
//if user enter the ingredient loop will start ask for ingredient details.
recipeIngredients.add(ingredientName); //creating array list for recipe ingredients.
//prompt user to enter ingredient amount
System.out.println("Please enter an ingredient amount: ");
float ingredientAmount = scnr.nextFloat();
//prompt user to enter measuring unit of ingredient
System.out.println("Please enter the measurement unit for an ingredient: ");
String unitMeasurement = scnr.next();
//promt user to enter ingredient calories
System.out.println("Please enter ingredient calories: ");
int ingredientCalories = scnr.nextInt();
//Expression to calculate total calories
totalRecipeCalories = (ingredientCalories * ingredientAmount);
//prints the ingredient name with required amount, measuring unit and total calories.
System.out.println(ingredientName + " uses " + ingredientAmount + " " + unitMeasurement + " and has " + totalRecipeCalories + " calories.");
} //end else loop.
} while (addMoreIngredients);
}
}
/**
*
* Final Project Details:
*
* For your final project submission, you should add a menu item and a method
* to access the custom method you developed for the Recipe class
* based on the Stepping Stone 5 Lab.
*
*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
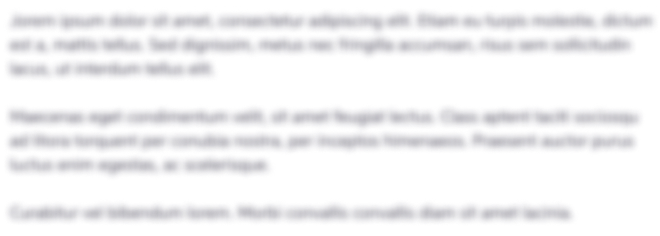
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started