Question
I need this done in C++ Can someone help me get my code from crashing. The code compiles but it can be easily crashed with
I need this done in C++
Can someone help me get my code from crashing. The code compiles but it can be easily crashed with user input. The rubric and code below
Instructions:
Write a program that scores the following data about a soccer player in a structure:
Players Name
Players Number
Points Scored by Player
The program should keep an array of 12 of these structures. Each element is for a different player on a team. When the program runs, it should ask the user to enter the data for each player. It should then show a table that lists each players number, name, and points scored. The program should also calculate and display the total points earned by the team. The number and name of the player who has earned the most points should also be displayed.
no break statements, infinite loops, or go-to labels
#include
// Declaration of the Player structure
struct Player { char name[45]; // Player's name int number; // Player's number int points; // Points scored by the player };
const int numPlayers = 12; // The number of players
// Function prototypes void getPlayerInfo(Player &); void showInfo(Player); int getTotalPoints(Player[], int); void showHighest(Player[], int);
//*********************************************** // Function main * //***********************************************
int main() { Player team[numPlayers]; int index;
for (index = 0; index < 12; index++) { cout << " PLAYER #" << (index + 1) << " "; cout << "--------- "; getPlayerInfo(team[index]); cin.get(); }
cout.width(20); cout.setf(ios::left); cout << " NAME"; cout.width(10); cout << "NUMBER"; cout.width(10); cout << "POINTS SCORED "; for (index = 0; index < 12; index++) showInfo(team[index]); cout << "TOTAL POINTS: " << getTotalPoints(team, numPlayers) << endl; showHighest(team, numPlayers); }
//*********************************************** // Function getPlayer * // This function accepts a reference to a Player* // structure variable. The user is asked to * // enter the player's name, number, and the * // number of points scored. This data is stored * // in the reference parameter. * //***********************************************
void getPlayerInfo(Player &p) { while (p.points < 0) { cout << p.points << endl; cout << "Player name: "; cin.getline(p.name, 45); cout << "Player's number: "; cin >> p.number; cout << "Points scored: "; cin >> p.points; } }
//*********************************************** // Function showInfo * // This function displays the data in the Player* // structure variable passed into the parameter.* //***********************************************
void showInfo(Player p) { cout << setw(20) << p.name; cout << setw(10) << p.number; cout << setw(10) << p.points << endl; }
//*********************************************** // Function getTotalPoints * // This function accepts an array of Player * // structure variables as its argument. The * // function calciulates and returns the total * // of all the players points in the array. * //***********************************************
int getTotalPoints(Player p[], int size) { int total = 0; for (int index = 0; index < size; index++) total += p[index].points; return total; }
//*********************************************** // Function showHighest * // This function accepts an array of Player * // structure variables. It displays the name * // of the player who scored the most points. * //***********************************************
void showHighest(Player p[], int size) { int highest = 0, highPoints = p[0].points;
for (int index = 1; index < size; index++) { if (p[index].points > highPoints) { highest = index; highPoints = p[index].points; } } cout << "The player who scored the most points is: "; cout << p[highest].name << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
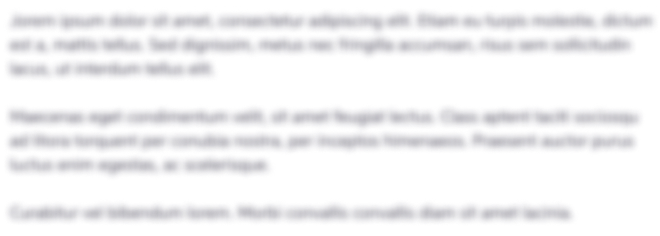
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started