Question
I need to fill in two methods within the Expression class. One which will populate the premade array lists for integers and arrays respectively. The
I need to fill in two methods within the "Expression" class. One which will populate the premade array lists for integers and arrays respectively.
The classes we were given are as follows:
package app;
/**
* This class holds a (name, array of integer values) pair for an array.
* The name is a sequence of one or more letters.
*
* @author ru-nb-cs112
*
*/
public class Array {
/**
* Name, sequence of letters
*/
public String name;
/**
* Array of integer values
*/
public int[] values;
/**
* Initializes with name, and sets values to null.
*
* @param name Name of array
*/
public Array(String name) {
this.name = name;
values = null;
}
/* (non-Javadoc)
* @see java.lang.Object#toString()
*/
public String toString() {
if (values == null || values.length == 0) {
return name + "=[ ]";
}
StringBuilder sb = new StringBuilder();
sb.append(name);
sb.append("=[");
sb.append(values[0]);
for (int i=1; i < values.length; i++) {
sb.append(',');
sb.append(values[i]);
}
sb.append(']');
return sb.toString();
}
/* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
public boolean equals(Object o) {
if (o == null || !(o instanceof Array)) {
return false;
}
Array as = (Array)o;
return name.equals(as.name);
}
}
ackage app;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Scanner;
public class Evaluator {
/**
* @param args
*/
public static void main(String[] args) throws IOException {
Scanner sc = new Scanner(System.in);
while (true) {
System.out.print(" Enter the expression, or hit return to quit => ");
String expr = sc.nextLine();
if (expr.length() == 0) {
break;
}
ArrayList
ArrayList
Expression.makeVariableLists(expr, vars, arrays);
System.out.print("Enter variable values file name, or hit return if no variables => ");
String fname = sc.nextLine();
if (fname.length() != 0) {
Scanner scfile = new Scanner(new File(fname));
Expression.loadVariableValues(scfile, vars, arrays);
}
System.out.println("Value of expression = " + Expression.evaluate(expr,vars,arrays));
}
sc.close();
}
}
package app;
/**
* This class holds a (name, integer value) pair for a simple (non-array) variable.
* The variable name is a sequence of one or more letters.
*
* @author ru-nb-cs112
*
*/
public class Variable {
/**
* Name, sequence of letters
*/
public String name;
/**
* Integer value
*/
public int value;
/**
* Initializes with name, and zero value
*
* @param name Variable name
*/
public Variable(String name) {
this.name = name;
value = 0;
}
/* (non-Javadoc)
* @see java.lang.Object#toString()
*/
public String toString() {
return name + "=" + value;
}
/* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
public boolean equals(Object o) {
if (o == null || !(o instanceof Variable)) {
return false;
}
Variable ss = (Variable)o;
return name.equals(ss.name);
}
}
package structures;
import java.util.ArrayList;
import java.util.NoSuchElementException;
/**
* A generic stack implementation.
*
* @author ru-nb-cs111
*
* @param
*/
public class Stack
/**
* Items in the stack.
*/
private ArrayList
/**
* Initializes stack to empty.
*/
public Stack() {
items = new ArrayList
}
/**
* Pushes a new item on top of stack.
*
* @param item Item to push.
*/
public void push(T item) {
items.add(item);
}
/**
* Pops item at top of stack and returns it.
*
* @return Popped item.
* @throws NoSuchElementException If stack is empty.
*/
public T pop()
throws NoSuchElementException {
if (items.isEmpty()) {
//return null;
throw new NoSuchElementException("can't pop from an empty stack");
}
return items.remove(items.size()-1);
}
/**
* Returns item on top of stack, without popping it.
*
* @return Item at top of stack.
* @throws NoSuchElementException If stack is empty.
*/
public T peek()
throws NoSuchElementException {
if (items.size() == 0) {
//return null;
throw new NoSuchElementException("can't peek on an empty stack");
}
return items.get(items.size()-1);
}
/**
* Tells if stack is empty.
*
* @return True if stack is empty, false if not.
*/
public boolean isEmpty() {
return items.isEmpty();
}
/**
* Returns number of items in stack.
*
* @return Number of items in stack.
*/
public int size() {
return items.size();
}
/**
* Empties the stack.
*/
public void clear() {
items.clear();
}
}
package app;
import java.io.*;
import java.util.*;
import java.util.regex.*;
import structures.Stack;
public class Expression {
public static String delims = " \t*+-/()[]";
/**
* Populates the vars list with simple variables, and arrays lists with arrays
* in the expression. For every variable (simple or array), a SINGLE instance is created
* and stored, even if it appears more than once in the expression.
* At this time, values for all variables and all array items are set to
* zero - they will be loaded from a file in the loadVariableValues method.
*
* @param expr The expression
* @param vars The variables array list - already created by the caller
* @param arrays The arrays array list - already created by the caller
*/
public static void
makeVariableLists(String expr, ArrayList
/** COMPLETE THIS METHOD **/
/** DO NOT create new vars and arrays - they are already created before being sent in
** to this method - you just need to fill them in.
**/
}
/**
* Loads values for variables and arrays in the expression
*
* @param sc Scanner for values input
* @throws IOException If there is a problem with the input
* @param vars The variables array list, previously populated by makeVariableLists
* @param arrays The arrays array list - previously populated by makeVariableLists
*/
public static void
loadVariableValues(Scanner sc, ArrayList
throws IOException {
while (sc.hasNextLine()) {
StringTokenizer st = new StringTokenizer(sc.nextLine().trim());
int numTokens = st.countTokens();
String tok = st.nextToken();
Variable var = new Variable(tok);
Array arr = new Array(tok);
int vari = vars.indexOf(var);
int arri = arrays.indexOf(arr);
if (vari == -1 && arri == -1) {
continue;
}
int num = Integer.parseInt(st.nextToken());
if (numTokens == 2) { // scalar symbol
vars.get(vari).value = num;
} else { // array symbol
arr = arrays.get(arri);
arr.values = new int[num];
// following are (index,val) pairs
while (st.hasMoreTokens()) {
tok = st.nextToken();
StringTokenizer stt = new StringTokenizer(tok," (,)");
int index = Integer.parseInt(stt.nextToken());
int val = Integer.parseInt(stt.nextToken());
arr.values[index] = val;
}
}
}
}
/**
* Evaluates the expression.
*
* @param vars The variables array list, with values for all variables in the expression
* @param arrays The arrays array list, with values for all array items
* @return Result of evaluation
*/
public static float
evaluate(String expr, ArrayList
/** COMPLETE THIS METHOD **/
// following line just a placeholder for compilation
return 0;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
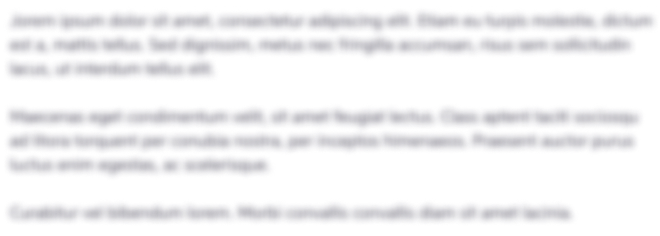
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started