Question
i need to finish the update student method. Should be able to list the students, the user picks the student they want to edit. it
i need to finish the update student method. Should be able to list the students, the user picks the student they want to edit. it then lists there courses. once that happens. there should be a sub menu where they can edit the name, or the class, delete a class and add a class. attached is all of the header files and there .cpp. should only need to work in the main.cpp but i cannot figure it out. THIS IS IN CPP AND I ONLY NEED THE UPDATE STUDENT METHOD COMPLETE. EVERYTHING ELSE WORKS.
MAIN.CPP:
#include "StudentDB.h" #include "Student.h" #include "Course.h" #include
void addStudentMenu(StudentDB& db); void mainMenu(StudentDB& db); void promptForCourseInfo(std::string& name, std::string& department, std::string& semester, int& grade);
void deleteStudentMenu(StudentDB& db); void updateStudentMenu(StudentDB& db); int main(){ StudentDB db;
mainMenu(db); return 0; }
void mainMenu(StudentDB& db){ int choice; while(true){ std::cout<< "MAIN MENU" << std::endl; std::cout << "1. Add a student" << std::endl; std::cout << "2. Delete a student" << std::endl; std::cout << "3. Update a student" << std::endl; std::cout << "4. Get number of students" << std::endl; std::cout << "5. Exit" << std::endl; std::cout << "Choice: "; std::cin >> choice; std::cin.ignore(); switch(choice){ case 1: addStudentMenu(db); break; case 2: deleteStudentMenu(db); break; case 3: updateStudentMenu(db); break; case 4: std::cout << "There are " << db.getNumberofStudents() << " students" << std::endl; break; case 5: return; default: std::cout << "Unkonwn Choice " << choice << std::endl; } } }
void addStudentMenu(StudentDB& db){ std::string name; int numCourses; std::cout << "What is the students name: "; getline(std::cin, name); std::cout << "How many courses do you want to enter for the student? "; std::cin >> numCourses; std::cin.ignore(); Student* student = db.createNewStudent(name); for(int i = 0; i < numCourses; ++i){ std::string name; std::string department; std::string semester; int grade; promptForCourseInfo(name, department, semester, grade); db.createNewClass(student, name, department, semester, grade); } }
void promptForCourseInfo(std::string& name, std::string& department, std::string& semester, int& grade){ std::cout << "Please enter the name of the course: "; getline(std::cin, name); std::cout << "Please enter the department of the course: "; getline(std::cin, department); std::cout << "Please enter the semester you took the course: "; getline(std::cin, semester); std::cout << "Please enter the number grade you got on the course: "; std::cin >> grade; std::cin.ignore(); } void deleteStudentMenu(StudentDB& db){ Student* temp = db.getFirstStudent(); while(temp != nullptr){ db.printStudent(temp, false); temp = temp->getNextStudent(); } std::string nameToDelete; std::cout << "Enter name of student you wish to delete: "; getline(std::cin, nameToDelete);
if(temp != nullptr){ db.deleteStudent(temp); std::cout << "Successfully deleted " << nameToDelete << std::endl; } else{ std::cout << "That student does not exist!" << std::endl; } }
void updateStudentMenu(StudentDB& db){ Student* temp = db.getFirstStudent(); Course* tempClass = nullptr; while(temp != nullptr){ db.printStudent(temp, false); temp = temp->getNextStudent(); } std::string nameToUpdate; std::cout << "Enter name of student you wish to Update: "; getline(std::cin, nameToUpdate);
temp = db.lookupStudent(nameToUpdate); tempClass = db.lookupClass(temp, nameToUpdate); db.printCourse(tempClass);
}
/* MAIN MENU: 1. Add a student 2. Delete a student 3. Update a student 4. Get number of students 5. Exit
-- 1. ADD A STUDENT name: number of courses taken: for loop to get all courses including semester taken and grade and department
-- 2. DELETE A STUDENT list just student names enter student name then it deletes them and there data -- 3. UPDATE A STUDENT list student names select student (type in name) -- Show student information SUB-MENU: STUDENT INFORMATIN 1. Edit Name => Prompt for new name 2. Edit Class => List classes and prompt user 3. Delete Class => List classes and prompt user 4. Add Class => prompts for relevent info
*/
STUDENTDB.CPP:
#include "StudentDB.h" #include "Student.h" #include "Course.h" #include
StudentDB::StudentDB(){ m_studentCounter = 0; m_firstStudent = nullptr; }
Student* StudentDB::getFirstStudent(){ return m_firstStudent; }
void StudentDB::updateStudent(Student* student, std::string name){ student->setName(name); }
void StudentDB::updateCourse(Course* course, std::string name, std::string department, std::string semester, int grade){ course->setName(name); course->setDepartment(department); course->setSemester(semester); course->setGrade(grade); }
Student* StudentDB::lookupStudent(std::string name){ Student* temp = m_firstStudent; while(temp != nullptr && temp->getName() != name){ temp = temp->getNextStudent(); } return temp; }
Course* StudentDB::lookupClass(Student* student, std::string name){ Course* temp = student->getFirstCourse(); while(temp != nullptr && temp->getName() != name){ temp = temp->getNextCourse(); } return temp; }
Student* StudentDB::createNewStudent(std::string name){ Student* student = new Student(name); if(m_firstStudent == nullptr){ m_firstStudent = student; } else{ student->setNextStudent(m_firstStudent); m_firstStudent = student; } m_studentCounter++; return student; }
Course* StudentDB::createNewClass(Student* student, std::string name, std::string department, std::string semester, int grade){ Course* course = new Course(name, department, semester, grade); student->addCourse(course); return course; }
void StudentDB::deleteStudent(Student* student) { // Find the student in the linked list // Keep track of its previous element as well // Point previous' next element to be
void StudentDB::printStudent(Student* student, bool printCourses) { std::cout << "name: " << student->getName() << std::endl; if(printCourses){ Course* temp = student->getFirstCourse(); while(temp != nullptr){ printCourse(temp); } } }
void StudentDB::printCourse(Course* course) { std::cout << "Course" << std::endl; std::cout << "-------" << std::endl; std::cout << "Name: " << course->getName() << std::endl; std::cout << "Department: " << course->getDepartment() << std::endl; std::cout << "Semester: " << course->getSemester() << std::endl; std::cout << "Grade: " << course->getGrade() << std::endl; }
int StudentDB::getNumberofStudents() { return m_studentCounter; }
STUDENTDB.H:
#ifndef STUDENTDB_H #define STUDENTDB_H
#include "Course.h" #include "Student.h" #include
class StudentDB{ private: Student* m_firstStudent; int m_studentCounter; public: StudentDB(); void updateStudent(Student*, std::string); void updateCourse(Course*, std::string, std::string,std::string, int); Student* getFirstStudent(); Student* lookupStudent(std::string); Course* lookupClass(Student*, std::string); Student* createNewStudent(std::string); Course* createNewClass(Student*, std::string, std::string, std::string, int); void deleteStudent(Student*); void printStudent(Student*, bool); void printCourse(Course*); int getNumberofStudents(); };
#endif
COURSE,CPP:
#include "Course.h" #include
Course::Course(std::string name, std::string department, std::string semester, int grade) { m_name = name; m_department = department; m_semester = semester; m_grade = grade; m_nextCourse = nullptr; }
Course* Course::getNextCourse() { return m_nextCourse; }
void Course::setNextCourse(Course* nextCourse) { m_nextCourse = nextCourse; }
void Course::setName(std::string name) { m_name = name;
} std::string Course::getName() { return m_name; }
void Course::setDepartment(std::string department) { m_department = department; } std::string Course::getDepartment() { return m_department; }
void Course::setSemester(std::string semester) { m_semester = semester; } std::string Course::getSemester() { return m_semester; }
void Course::setGrade(int grade) { m_grade = grade; } int Course::getGrade() { return m_grade; }
COURSE.H:
#ifndef COURSE_H #define COURSE_H
#include
class Course { private: std::string m_name; std::string m_department; std::string m_semester; int m_grade; Course *m_nextCourse; public: Course(std::string, std::string, std::string, int);
Course* getNextCourse(); void setNextCourse(Course*);
void setName(std::string); std::string getName();
void setDepartment(std::string); std::string getDepartment();
void setSemester(std::string); std::string getSemester();
void setGrade(int); int getGrade(); };
#endif
STUDENT.CPP:
#include "Student.h" #include "Course.h" #include
Student::Student(std::string name){ m_course = nullptr; m_name = name; m_nextStudent = nullptr; }
Course* Student::getFirstCourse(){ return m_course; }
void Student::setName(std::string name){ m_name = name; } std::string Student::getName(){ return m_name; }
void Student::addCourse(Course* course){ if(m_course == nullptr){ m_course = course; } else{ course->setNextCourse(m_course); m_course = course; } }
Course* Student::deleteCourse(Course* course){ Course* cursor = m_course; Course* previous = nullptr; while(cursor != nullptr && cursor != course){ previous = cursor; cursor = cursor->getNextCourse(); } if(cursor == nullptr) return nullptr; // We're removing the first element? if(previous == nullptr){ m_course = cursor->getNextCourse(); } else{ previous->setNextCourse(cursor->getNextCourse()); } return cursor; }
Student* Student::getNextStudent() { return m_nextStudent; }
void Student::setNextStudent(Student* nextStudent) { m_nextStudent= nextStudent; }
STUDENT.H:
#ifndef STUDENT_H #define STUDENT_H
#include "Course.h" #include
class Student{ private: std::string m_name; Course* m_course; Student* m_nextStudent; public: Student(std::string); Course* getFirstCourse(); void addCourse(Course*); Course* deleteCourse(Course*); Student* getNextStudent(); void setNextStudent(Student*); void setName(std::string); std::string getName(); };
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
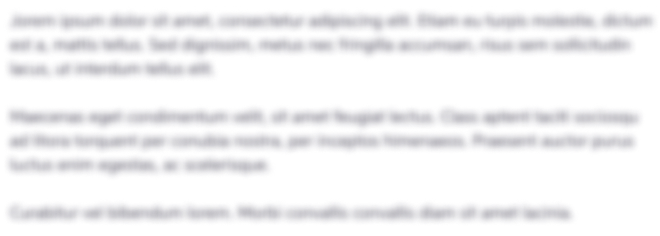
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started