Question
I need you to do Graphical User Interface to this program for instance: the program is allows you to play a guessing game. I will
I need you to do Graphical User Interface to this program for instance: the program is allows you to play a guessing game. I will think of a number between 1 and 100 and will allow you to guess until you get it. For each guess, I will tell you whether the right answer is higher or lower than your guess.
here is the code for the program :
import java.util.*;
public class lap1a {
public static void main(String[] args) {
giveIntro();
Scanner console = new Scanner(System.in);
char choice;
// pick a random number from 0 to 99 inclusive
Random rand = new Random();
do{ // iterates until user wants to quit
int given = rand.nextInt(100);
// get first guess
int guess = getGuess(console);
// give hints until correct guess is reached
while (guess != given) {
int numMatches = matches(given, guess);
if(numMatches < 0){ // if numMatches is less than 0, then the number is lower
System.out.println("It's lower");
}
else if(numMatches > 0){ // if numMatches is greater than 0, then the number is higher
System.out.println("It's higher");
}
guess = getGuess(console);
}
System.out.println("Congratulations! You win!");
System.out.print("Do you want to play again?" );
choice = console.next().charAt(0);
System.out.println();
}while(choice == 'y' || choice == 'Y');
}
// method to give introduction
public static void giveIntro() {
System.out.println("This program allows you to play a guessing game.");
System.out.println("I will think of a number between 1 and 100");
System.out.println("and will allow you to guess until you get it.");
System.out.println("For each guess, I will tell you whether the right");
System.out.println("answer is higher or lower than your guess.");
System.out.println();
}
// Returns -1 if number is less than guess, 1 if number is greater, 0 if equal
public static int matches(int given, int guess) {
int numMatches = 0;
if(given > guess){
numMatches = 1;
}
else if(given < guess){
numMatches = -1;
}
return numMatches;
}
// Re-prompts until a number in the proper range is entered.
// post: guess is between 0 and 99
public static int getGuess(Scanner console) {
int guess = getInt(console, "Your guess? ");
while (guess < 0 || guess >= 100) {
System.out.println("Out of range; try again.");
guess = getInt(console, "Your guess? ");
}
return guess;
}
// Re-prompts until a number is entered.
public static int getInt(Scanner console, String prompt) {
System.out.print(prompt);
while (!console.hasNextInt()) {
console.next();
System.out.println("Not an integer; try again.");
System.out.print(prompt);
}
return console.nextInt();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
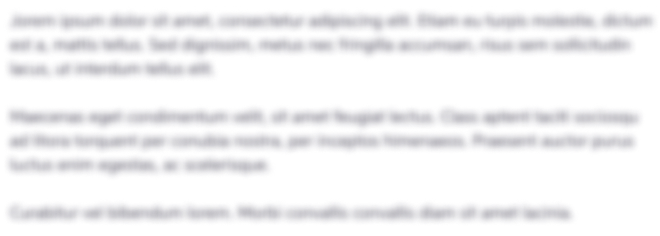
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started