Question
I needed help with this program. In this program, you will create an ordered singly linked list for managing timed events. You will simulate arrivals
I needed help with this program.
In this program, you will create an ordered singly linked list for managing timed events. You will simulate arrivals and departures at different "clock" times. You will advance a "clock" based on the next event time.
You have been furnished with an include file and a data file.
Examine the Person, Event, NodeLL, and Simulation typedefs in the cs2123p3.h include file. You may modify it to use a different representation of a linked list.
Input Data: (use stdin)
There are many records (terminated by EOF) that define arrivals:
szName iDeltaDepart iDeltaNextArrival
15s 3d 3d
Consider this input:
Ant,Adam 10 5
Mander,Sally 4 3
King,May 6 6
King,Joe 9 6
Graph,Otto 2 5
Based on that data, we have arrivals at these times:
Arrival Time | Person | Depart Delta | Next Arrival Delta |
0 | Ant,Adam | +10 | +5 |
5 | Mander,Sally | +4 | +3 |
8 | King,May | +6 | +6 |
14 | King,Joe | +9 | +6 |
20 | Graph,Otto | +2 | +5 |
25 | End of File (no arrival) | - | - |
For this program, there are only two event types (there will be different event types in Program #4):
EVT_ARRIVE - an arrival event
EVT_DEPART - a departure event
You are required to create a runSimulation(Simulation sim) function which is passed a sim (which points to a clock and a timed event list). runSimulation will loop through the timed events (which are placed in order by event time in the linked list) and set the clock to the time of the current timed event. Based on the event, (for now) it prints the time, person, and event. In program #4, the events will do more than just print a message.
(There are more events based on our input file than what is shown below.)
Time | Person | Event |
0 | Ant,Adam | Arrive |
5 | Mander,Sally | Arrive |
8 | King,May | Arrive |
9 | Mander,Sally | Depart |
10 | Ant,Adam | Depart |
14 | King,Joe | Arrive |
14 | King,May | Depart |
20 | Graph,Otto | Arrive |
22 | Graph,Otto | Depart |
23 | King,Joe | Depart |
23 | SIMULATION | TERMINATES |
Since there aren't any additional events, the simulation terminates.
Notice that some of the events are at the same time, but InsertOrderedLL in the notes didn't allow insertion of duplicates. You must change it.
Your output:
Print the time of each event, the person, and the event type (as text).
Turn in all of your C code, include file, and the output. As usual, your code must be written according to my standards.
***********************************************************************************************************************
/********************************************************************** cs2123p3.h Purpose: Defines constants: max constants error constants event type constants boolean constants Defines typedef for Token Person Event (instead of Element) For Linked List NodeLL LinkedListImp LinkedList For the simulation SimulationImp Simulation Protypes Functions provided by student Other functions provided by Larry previously (program 2) Utility functions provided by Larry previously (program 2) Notes: **********************************************************************/ /*** constants ***/ // Maximum constants #define MAX_TOKEN 50 // Maximum number of actual characters for a token #define MAX_LINE_SIZE 100 // Maximum number of character per input line // Error constants (program exit values) #define ERR_COMMAND_LINE 900 // invalid command line argument #define ERR_ALGORITHM 903 // Error in algorithm - almost anything else #define ERR_BAD_INPUT 503 // Bad input // Error Messages #define ERR_MISSING_SWITCH "missing switch" #define ERR_EXPECTED_SWITCH "expected switch, found" #define ERR_MISSING_ARGUMENT "missing argument for" // Event Constants #define EVT_ARRIVE 1 // when a person arrives #define EVT_DEPART 2 // when a person departs the simulation // exitUsage control #define USAGE_ONLY 0 // user only requested usage information #define USAGE_ERR -1 // usage error, show message and usage information // boolean constants #define FALSE 0 #define TRUE 1 /*** typedef ***/ // Token typedef used for operators, operands, and parentheses typedef char Token[MAX_TOKEN + 1]; // Person typedef typedef struct { char szName[16]; // Name int iDepartUnits; // time units representing how long he/she stays around } Person; // Event typedef (aka Element) typedef struct { int iEventType; // The type of event as an integer: // EVT_ARRIVE - arrival event // EVT_DEPART - departure event int iTime; // The time the event will occur Person person; // The person invokved in the event. } Event; // NodeLL typedef - these are the nodes in the linked list typedef struct NodeLL { Event event; struct NodeLL *pNext; // points to next node in the list } NodeLL; // typedefs for the ordered link list typedef struct { NodeLL *pHead; // Points to the head of the ordered list } LinkedListImp; typedef LinkedListImp *LinkedList; // typedefs for the Simulation typedef struct { int iClock; // clock time LinkedList eventList; } SimulationImp; typedef SimulationImp *Simulation; /********** prototypes ***********/ // linked list functions - you must provide the code for these (see course notes) int removeLL(LinkedList list, Event *pValue); NodeLL *insertOrderedLL(LinkedList list, Event value); NodeLL *searchLL(LinkedList list, int match, NodeLL **ppPrecedes); LinkedList newLinkedList(); NodeLL *allocateNodeLL(LinkedList list, Event value); // simulation functions - you must provide code for this void runSimulation(Simulation simulation); // functions in most programs, but require modifications void exitUsage(int iArg, char *pszMessage, char *pszDiagnosticInfo); // Utility routines provided by Larry (copy from program #2) void ErrExit(int iexitRC, char szFmt[], ...); char * getToken(char *pszInputTxt, char szToken[], int iTokenSize);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
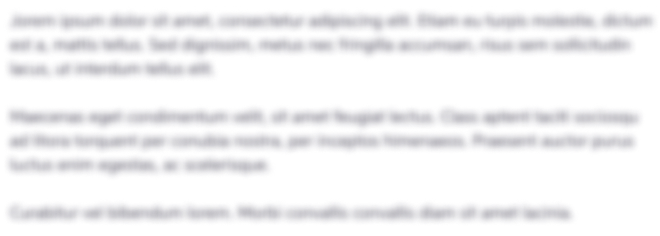
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started