I posted the same question 5 hours ago and didn't get an answer yet. Need an answer before tomorrow morning. Need help with this java program/ there is no input and output sample.
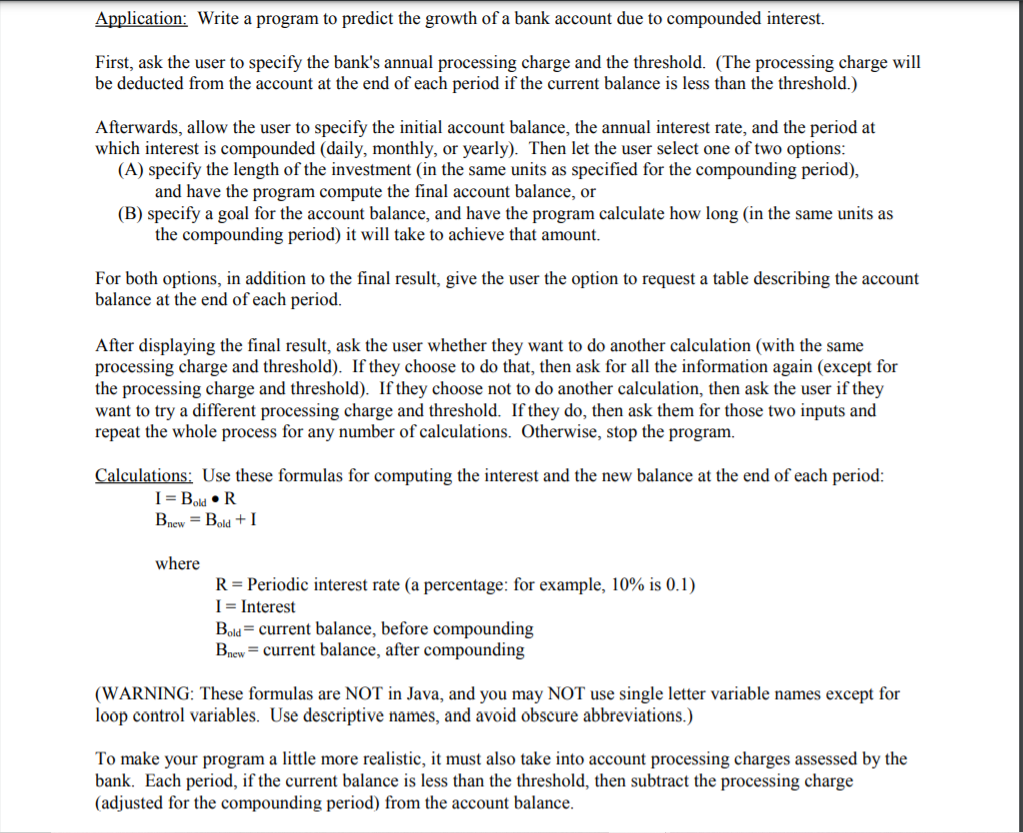
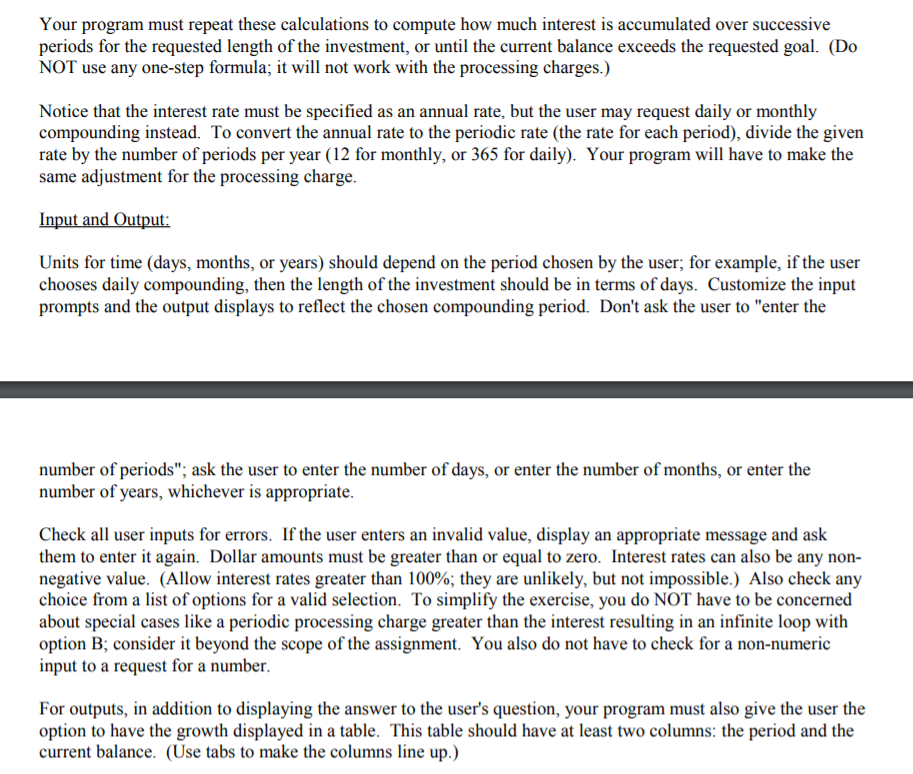
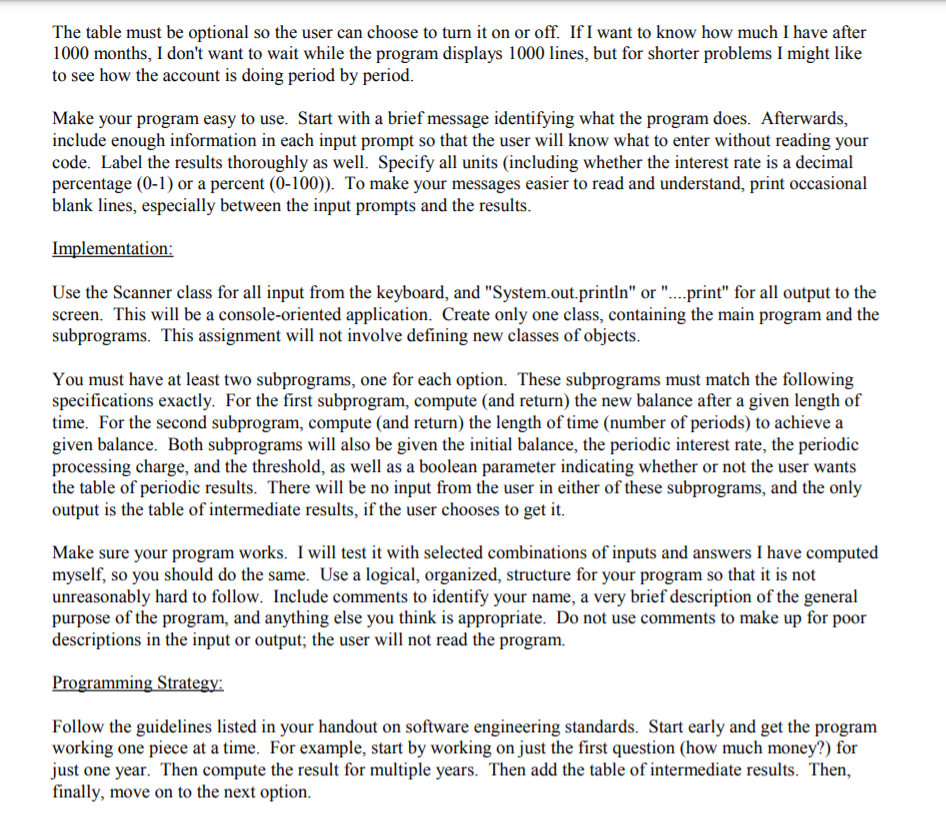
Application: Write a program to predict the growth of a bank account due to compounded interest. First, ask the user to specify the bank's annual processing charge and the threshold. (The processing charge will be deducted from the account at the end of each period if the current balance is less than the threshold.) Afterwards, allow the user to specify the initial account balance, the annual interest rate, and the period at which interest is compounded (daily, monthly, or yearly). Then let the user select one of two options: (A) specify the length of the investment in the same units as specified for the compounding period), and have the program compute the final account balance, or (B) specify a goal for the account balance, and have the program calculate how long (in the same units as the compounding period) it will take to achieve that amount. For both options, in addition to the final result, give the user the option to request a table describing the account balance at the end of each period. After displaying the final result, ask the user whether they want to do another calculation (with the same processing charge and threshold). If they choose to do that, then ask for all the information again (except for the processing charge and threshold). If they choose not to do another calculation, then ask the user if they want to try a different processing charge and threshold. If they do, then ask them for those two inputs and repeat the whole process for any number of calculations. Otherwise, stop the program. Calculations: Use these formulas for computing the interest and the new balance at the end of each period: I = Bold R Bnew = Bold + 1 where R= Periodic interest rate (a percentage: for example, 10% is 0.1) I = Interest Bold = current balance, before compounding Bnew = current balance, after compounding (WARNING: These formulas are NOT in Java, and you may NOT use single letter variable names except for loop control variables. Use descriptive names, and avoid obscure abbreviations.) To make your program a little more realistic, it must also take into account processing charges assessed by the bank. Each period, if the current balance is less than the threshold, then subtract the processing charge (adjusted for the compounding period) from the account balance. Your program must repeat these calculations to compute how much interest is accumulated over successive periods for the requested length of the investment, or until the current balance exceeds the requested goal. (Do NOT use any one-step formula, it will not work with the processing charges.) Notice that the interest rate must be specified as an annual rate, but the user may request daily or monthly compounding instead. To convert the annual rate to the periodic rate (the rate for each period), divide the given rate by the number of periods per year (12 for monthly, or 365 for daily). Your program will have to make the same adjustment for the processing charge. Input and Output: Units for time (days, months, or years) should depend on the period chosen by the user; for example, if the user chooses daily compounding, then the length of the investment should be in terms of days. Customize the input prompts and the output displays to reflect the chosen compounding period. Don't ask the user to "enter the number of periods"; ask the user to enter the number of days, or enter the number of months, or enter the number of years, whichever is appropriate. Check all user inputs for errors. If the user enters an invalid value, display an appropriate message and ask them to enter it again. Dollar amounts must be greater than or equal to zero. Interest rates can also be any non- negative value. (Allow interest rates greater than 100%; they are unlikely, but not impossible.) Also check any choice from a list of options for a valid selection. To simplify the exercise, you do NOT have to be concerned about special cases like a periodic processing charge greater than the interest resulting in an infinite loop with option B; consider it beyond the scope of the assignment. You also do not have to check for a non-numeric input to a request for a number. For outputs, in addition to displaying the answer to the user's question, your program must also give the user the option to have the growth displayed in a table. This table should have at least two columns: the period and the current balance. (Use tabs to make the columns line up.) The table must be optional so the user can choose to turn it on or off. If I want to know how much I have after 1000 months, I don't want to wait while the program displays 1000 lines, but for shorter problems I might like to see how the account is doing period by period. Make your program easy to use. Start with a brief message identifying what the program does. Afterwards, include enough information in each input prompt so that the user will know what to enter without reading your code. Label the results thoroughly as well. Specify all units (including whether the interest rate is a decimal percentage (0-1) or a percent (0-100)). To make your messages easier to read and understand, print occasional blank lines, especially between the input prompts and the results. Implementation: Use the Scanner class for all input from the keyboard, and "System.out.println" or "....print" for all output to the screen. This will be a console-oriented application. Create only one class, containing the main program and the subprograms. This assignment will not involve defining new classes of objects. You must have at least two subprograms, one for each option. These subprograms must match the following specifications exactly. For the first subprogram, compute (and return) the new balance after a given length of time. For the second subprogram, compute and return) the length of time (number of periods) to achieve a given balance. Both subprograms will also be given the initial balance, the periodic interest rate, the periodic processing charge, and the threshold, as well as a boolean parameter indicating whether or not the user wants the table of periodic results. There will be no input from the user in either of these subprograms, and the only output is the table of intermediate results, if the user chooses to get it. Make sure your program works. I will test it with selected combinations of inputs and answers I have computed myself, so you should do the same. Use a logical, organized, structure for your program so that it is not unreasonably hard to follow. Include comments to identify your name, a very brief description of the general purpose of the program, and anything else you think is appropriate. Do not use comments to make up for poor descriptions in the input or output; the user will not read the program. Programming Strategy: Follow the guidelines listed in your handout on software engineering standards. Start early and get the program working one piece at a time. For example, start by working on just the first question (how much money?) for just one year. Then compute the result for multiple years. Then add the table of intermediate results. Then, finally, move on to the next option. Application: Write a program to predict the growth of a bank account due to compounded interest. First, ask the user to specify the bank's annual processing charge and the threshold. (The processing charge will be deducted from the account at the end of each period if the current balance is less than the threshold.) Afterwards, allow the user to specify the initial account balance, the annual interest rate, and the period at which interest is compounded (daily, monthly, or yearly). Then let the user select one of two options: (A) specify the length of the investment in the same units as specified for the compounding period), and have the program compute the final account balance, or (B) specify a goal for the account balance, and have the program calculate how long (in the same units as the compounding period) it will take to achieve that amount. For both options, in addition to the final result, give the user the option to request a table describing the account balance at the end of each period. After displaying the final result, ask the user whether they want to do another calculation (with the same processing charge and threshold). If they choose to do that, then ask for all the information again (except for the processing charge and threshold). If they choose not to do another calculation, then ask the user if they want to try a different processing charge and threshold. If they do, then ask them for those two inputs and repeat the whole process for any number of calculations. Otherwise, stop the program. Calculations: Use these formulas for computing the interest and the new balance at the end of each period: I = Bold R Bnew = Bold + 1 where R= Periodic interest rate (a percentage: for example, 10% is 0.1) I = Interest Bold = current balance, before compounding Bnew = current balance, after compounding (WARNING: These formulas are NOT in Java, and you may NOT use single letter variable names except for loop control variables. Use descriptive names, and avoid obscure abbreviations.) To make your program a little more realistic, it must also take into account processing charges assessed by the bank. Each period, if the current balance is less than the threshold, then subtract the processing charge (adjusted for the compounding period) from the account balance. Your program must repeat these calculations to compute how much interest is accumulated over successive periods for the requested length of the investment, or until the current balance exceeds the requested goal. (Do NOT use any one-step formula, it will not work with the processing charges.) Notice that the interest rate must be specified as an annual rate, but the user may request daily or monthly compounding instead. To convert the annual rate to the periodic rate (the rate for each period), divide the given rate by the number of periods per year (12 for monthly, or 365 for daily). Your program will have to make the same adjustment for the processing charge. Input and Output: Units for time (days, months, or years) should depend on the period chosen by the user; for example, if the user chooses daily compounding, then the length of the investment should be in terms of days. Customize the input prompts and the output displays to reflect the chosen compounding period. Don't ask the user to "enter the number of periods"; ask the user to enter the number of days, or enter the number of months, or enter the number of years, whichever is appropriate. Check all user inputs for errors. If the user enters an invalid value, display an appropriate message and ask them to enter it again. Dollar amounts must be greater than or equal to zero. Interest rates can also be any non- negative value. (Allow interest rates greater than 100%; they are unlikely, but not impossible.) Also check any choice from a list of options for a valid selection. To simplify the exercise, you do NOT have to be concerned about special cases like a periodic processing charge greater than the interest resulting in an infinite loop with option B; consider it beyond the scope of the assignment. You also do not have to check for a non-numeric input to a request for a number. For outputs, in addition to displaying the answer to the user's question, your program must also give the user the option to have the growth displayed in a table. This table should have at least two columns: the period and the current balance. (Use tabs to make the columns line up.) The table must be optional so the user can choose to turn it on or off. If I want to know how much I have after 1000 months, I don't want to wait while the program displays 1000 lines, but for shorter problems I might like to see how the account is doing period by period. Make your program easy to use. Start with a brief message identifying what the program does. Afterwards, include enough information in each input prompt so that the user will know what to enter without reading your code. Label the results thoroughly as well. Specify all units (including whether the interest rate is a decimal percentage (0-1) or a percent (0-100)). To make your messages easier to read and understand, print occasional blank lines, especially between the input prompts and the results. Implementation: Use the Scanner class for all input from the keyboard, and "System.out.println" or "....print" for all output to the screen. This will be a console-oriented application. Create only one class, containing the main program and the subprograms. This assignment will not involve defining new classes of objects. You must have at least two subprograms, one for each option. These subprograms must match the following specifications exactly. For the first subprogram, compute (and return) the new balance after a given length of time. For the second subprogram, compute and return) the length of time (number of periods) to achieve a given balance. Both subprograms will also be given the initial balance, the periodic interest rate, the periodic processing charge, and the threshold, as well as a boolean parameter indicating whether or not the user wants the table of periodic results. There will be no input from the user in either of these subprograms, and the only output is the table of intermediate results, if the user chooses to get it. Make sure your program works. I will test it with selected combinations of inputs and answers I have computed myself, so you should do the same. Use a logical, organized, structure for your program so that it is not unreasonably hard to follow. Include comments to identify your name, a very brief description of the general purpose of the program, and anything else you think is appropriate. Do not use comments to make up for poor descriptions in the input or output; the user will not read the program. Programming Strategy: Follow the guidelines listed in your handout on software engineering standards. Start early and get the program working one piece at a time. For example, start by working on just the first question (how much money?) for just one year. Then compute the result for multiple years. Then add the table of intermediate results. Then, finally, move on to the next option