Question
I really need help with this: Overview: The program will create a Servlet that will respond to HTML requests with text streams. The servlet will
I really need help with this:
Overview: The program will create a Servlet that will respond to HTML requests with text streams. The servlet will have locally instantiated Expert System object. The website will allow a user to see results from the Expert System or add new data to the Expert System. Specifics: index.html Webpage that allows the user to select a link to go to the using page or a link to go to the training page. These buttons / links will call the servlet. The servlet will serve up either using.jsp (with appropriate data) or training.jsp. using.jsp Webpage that allows the user to query the Expert System using a drop down list of key words. Initially this page has no results, only the drop down list and a button. The button click will call the servlet. The servlet will serve up using.jsp with appropriate data. This page will also a button to go to the training page. training.jsp Webpage that allows the user to update the Expert System using two text fields. One is a key that may or may not already be in the Expert System. The other is a new value. The button click will call the servlet. The servlet will either add to an existing keys values (if the key already exists) or will add a new key / value pair. The servlet will serve up the training.jsp page. This page will also a button to go to the using page. DataServlet.java The servlet class that manages all of the requests and responses.
here is the code:
song.java
package project2; public class Song { private String name; private String itemCode; private String description; private String artist; private String album; private String price;
Song(String name, String itemCode, String description, String artist, String album, String price) { this.name = name; this.itemCode = itemCode; this.description = description; this.artist = artist; this.album = album; this.price = price; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getItemCode() { return itemCode; }
public void setItemCode(String itemCode) { this.itemCode = itemCode; }
public String getDescription() { return description; }
public void setDescription(String description) { this.description = description; }
public String getArtist() { return artist; }
public void setArtist(String artist) { this.artist = artist; }
public String getAlbum() { return album; }
public void setAlbum(String album) { this.album = album; }
public String getPrice() { return price; }
public void setPrice(String price) { this.price = price; }
}
songdatabase.java:
package project2;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Scanner;
import javax.swing.*;
public class SongDatabase extends JFrame implements ActionListener, ItemListener {
private JButton addButton, editButton, deleteButton, acceptButton, cancelButton, exitButton;// saveButton;
private JLabel lbSelectSong, lbSongCode, lbSongDescription, lbSongArtist, lbSongAlbum, lbSongPrice;
private JTextField tfSongCode, tfSongDescription, tfSongArtist, tfSongAlbum, tfSongPrice;
private JComboBox cbSelectSong;
ArrayList
boolean flag = false;
public static void main(String[] args) {
new SongDatabase();
}
public void readFile() {
String filename = "C:/Users/user/Desktop/playlist/songList.txt";
try
{
FileReader inputFile = new FileReader(filename);
BufferedReader bufferedReader=new BufferedReader(inputFile);
String line=null;
while((line=bufferedReader.readLine())!=null)
{
String lineInfo = line;
String[] data = lineInfo.split(",");
String name = data[0];
String itemCode = data[1];
String description = data[2];
String artist = data[3];
String album = data[4];
String price = data[5];
Song song = new Song(name, itemCode, description, artist, album, price);
songList.add(song);
}
bufferedReader.close();
inputFile.close();
}
catch (IOException e)
{
System.out.println(e);
}
}
public SongDatabase() {
songList = new ArrayList
readFile();
arrangePosition();
Container con = this.getContentPane();
con.setLayout(null);
lbSelectSong = new JLabel("Select Song");
lbSelectSong.setBounds(100, 30, 120, 25);
con.add(lbSelectSong);
cbSelectSong = new JComboBox();
for (int i = 0; i < songList.size(); i++) {
Song song = songList.get(i);
cbSelectSong.addItem(i + 1 + ". " + song.getName());
}
cbSelectSong.setBounds(200, 30, 200, 25);
cbSelectSong.addItemListener(this);
add(cbSelectSong);
lbSongCode = new JLabel("Item Code");
lbSongCode.setBounds(100, 70, 120, 25);
con.add(lbSongCode);
tfSongCode = new JTextField(20);
tfSongCode.setBounds(200, 70, 200, 25);
con.add(tfSongCode);
lbSongDescription = new JLabel("Description");
lbSongDescription.setBounds(100, 110, 120, 25);
con.add(lbSongDescription);
tfSongDescription = new JTextField(20);
tfSongDescription.setBounds(200, 110, 200, 25);
con.add(tfSongDescription);
lbSongArtist = new JLabel("Artist");
lbSongArtist.setBounds(100, 150, 120, 25);
con.add(lbSongArtist);
tfSongArtist = new JTextField(20);
tfSongArtist.setBounds(200, 150, 200, 25);
con.add(tfSongArtist);
lbSongAlbum = new JLabel("Album");
lbSongAlbum.setBounds(100, 190, 120, 25);
con.add(lbSongAlbum);
tfSongAlbum = new JTextField(20);
tfSongAlbum.setBounds(200, 190, 200, 25);
con.add(tfSongAlbum);
lbSongPrice = new JLabel("Price");
lbSongPrice.setBounds(100, 230, 120, 25);
con.add(lbSongPrice);
tfSongPrice = new JTextField(20);
tfSongPrice.setBounds(200, 230, 200, 25);
con.add(tfSongPrice);
addButton = new JButton("Add");
addButton.setBounds(10, 310, 90, 30);
addButton.addActionListener(this);
con.add(addButton);
editButton = new JButton("Edit");
editButton.setBounds(110, 310, 80, 30);
editButton.addActionListener(this);
con.add(editButton);
deleteButton = new JButton("Delete");
deleteButton.setBounds(210, 310, 80, 30);
deleteButton.addActionListener(this);
con.add(deleteButton);
acceptButton = new JButton("Accept");
acceptButton.setBounds(310, 310, 80, 30);
acceptButton.addActionListener(this);
con.add(acceptButton);
cancelButton = new JButton("Cancel");
cancelButton.setBounds(410, 310, 80, 30);
cancelButton.addActionListener(this);
con.add(cancelButton);
exitButton = new JButton("Exit");
exitButton.setBounds(170, 370, 80, 30);
exitButton.addActionListener(this);
con.add(exitButton);
if (songList.size() > 0) {
Song song = songList.get(0);
tfSongCode.setText(song.getItemCode());
tfSongDescription.setText(song.getDescription());
tfSongArtist.setText(song.getArtist());
tfSongAlbum.setText(song.getAlbum());
tfSongPrice.setText(song.getPrice());
}
tfSongCode.setEnabled(false);
tfSongDescription.setEnabled(false);
tfSongArtist.setEnabled(false);
tfSongAlbum.setEnabled(false);
tfSongPrice.setEnabled(false);
addButton.setEnabled(true);
editButton.setEnabled(true);
deleteButton.setEnabled(true);
acceptButton.setEnabled(false);
cancelButton.setEnabled(false);
exitButton.setEnabled(true);
setTitle("Song Database");
setSize(500, 500);
setVisible(true);
setResizable(true);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
}
public void arrangePosition() {
Toolkit tookit = Toolkit.getDefaultToolkit();
Dimension scDimentions = tookit.getScreenSize();
int scHeight = (int) scDimentions.getHeight();
int scWidth = (int) scDimentions.getWidth();
int x = (scWidth - 500) / 2;
int y = (scHeight - 600) / 2;
setLocation(x, y);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
Toolkit.getDefaultToolkit().beep();
flag = false;
editButton.setEnabled(false);
deleteButton.setEnabled(false);
acceptButton.setEnabled(true);
cancelButton.setEnabled(true);
tfSongCode.setText("");
tfSongDescription.setText("");
tfSongArtist.setText("");
tfSongAlbum.setText("");
tfSongPrice.setText("");
tfSongCode.setEnabled(true);
tfSongDescription.setEnabled(true);
tfSongArtist.setEnabled(true);
tfSongAlbum.setEnabled(true);
tfSongPrice.setEnabled(true);
}
if (e.getSource() == editButton) {
Toolkit.getDefaultToolkit().beep();
flag = true;
tfSongCode.setEnabled(false);
tfSongDescription.setEnabled(true);
tfSongArtist.setEnabled(true);
tfSongAlbum.setEnabled(false);
tfSongPrice.setEnabled(true);
addButton.setEnabled(false);
editButton.setEnabled(false);
deleteButton.setEnabled(false);
acceptButton.setEnabled(true);
cancelButton.setEnabled(true);
exitButton.setEnabled(true);
tfSongDescription.setText("");
tfSongArtist.setText("");
tfSongPrice.setText("");
if (tfSongDescription.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song description", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongArtist.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song artist", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongPrice.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song price", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
}
if (e.getSource() == deleteButton) {
if (tfSongCode.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song code", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongDescription.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song description", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongArtist.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song artist", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongAlbum.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song album", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongPrice.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song price", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
String name = tfSongDescription.getText();
String itemCode = tfSongCode.getText();
String description = tfSongDescription.getText();
String artist = tfSongArtist.getText();
String album = tfSongAlbum.getText();
String price = tfSongPrice.getText();
if (!flag) {
Song song = new Song(name, itemCode, description, artist, album, price);
songList.add(song);
cbSelectSong.addItem(song.getName());
JOptionPane.showMessageDialog(null, "song deleted into list", "Note", JOptionPane.INFORMATION_MESSAGE);
} else {
Song song = new Song(name, itemCode, description, artist, album, price);
int index = cbSelectSong.getSelectedIndex();
songList.set(index, song);
cbSelectSong.remove(index);
cbSelectSong.addItem(name);
JOptionPane.showMessageDialog(null, "new song updated successfully", "Note", JOptionPane.INFORMATION_MESSAGE);
}
}
if (e.getSource() == cancelButton) {
if (songList.size() > 0) {
cbSelectSong.setSelectedIndex(0);
Song song = songList.get(0);
tfSongCode.setText(song.getItemCode());
tfSongDescription.setText(song.getDescription());
tfSongArtist.setText(song.getArtist());
tfSongAlbum.setText(song.getAlbum());
tfSongPrice.setText(song.getPrice());
}
tfSongCode.setEnabled(false);
tfSongDescription.setEnabled(false);
tfSongArtist.setEnabled(false);
tfSongAlbum.setEnabled(false);
tfSongPrice.setEnabled(false);
addButton.setEnabled(true);
editButton.setEnabled(true);
deleteButton.setEnabled(true);
acceptButton.setEnabled(true);
cancelButton.setEnabled(false);
exitButton.setEnabled(true);
}
if (e.getSource() == acceptButton) {
if (tfSongCode.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song code", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongDescription.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song description", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongArtist.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song artist", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongAlbum.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song album", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
if (tfSongPrice.getText().length() == 0) {
JOptionPane.showMessageDialog(null, "Enter song price", "Note", JOptionPane.INFORMATION_MESSAGE);
return;
}
String name = tfSongDescription.getText();
String itemCode = tfSongCode.getText();
String description = tfSongDescription.getText();
String artist = tfSongArtist.getText();
String album = tfSongAlbum.getText();
String price = tfSongPrice.getText();
if (!flag) {
Song song = new Song(name, itemCode, description, artist, album, price);
songList.add(song);
cbSelectSong.addItem(song.getName());
JOptionPane.showMessageDialog(null, "New song added into list", "Note", JOptionPane.INFORMATION_MESSAGE);
} else {
Song song = new Song(name, itemCode, description, artist, album, price);
int index = cbSelectSong.getSelectedIndex();
songList.set(index, song);
cbSelectSong.remove(index);
cbSelectSong.addItem(name);
JOptionPane.showMessageDialog(null, "new song updated successfully", "Note", JOptionPane.INFORMATION_MESSAGE);
}
}
if (e.getSource() == cancelButton) {
if (songList.size() > 0) {
cbSelectSong.setSelectedIndex(0);
Song song = songList.get(0);
tfSongCode.setText(song.getItemCode());
tfSongDescription.setText(song.getDescription());
tfSongArtist.setText(song.getArtist());
tfSongAlbum.setText(song.getAlbum());
tfSongPrice.setText(song.getPrice());
}
tfSongCode.setEnabled(false);
tfSongDescription.setEnabled(false);
tfSongArtist.setEnabled(false);
tfSongAlbum.setEnabled(false);
tfSongPrice.setEnabled(false);
addButton.setEnabled(true);
editButton.setEnabled(true);
deleteButton.setEnabled(true);
acceptButton.setEnabled(false);
cancelButton.setEnabled(false);
exitButton.setEnabled(true);
}
if (e.getSource() == exitButton) {
Toolkit.getDefaultToolkit().beep();
System.exit(0);
}
}
@Override
public void itemStateChanged(ItemEvent ie) {
int index = cbSelectSong.getSelectedIndex();
Song song = songList.get(index);
tfSongCode.setText(song.getItemCode());
tfSongDescription.setText(song.getDescription());
tfSongArtist.setText(song.getArtist());
tfSongAlbum.setText(song.getAlbum());
tfSongPrice.setText(song.getPrice());
tfSongCode.setEnabled(false);
tfSongDescription.setEnabled(false);
tfSongArtist.setEnabled(false);
tfSongAlbum.setEnabled(false);
tfSongPrice.setEnabled(false);
}
}
songlist.txt:
Take five, BT012, jazz, dave brubeck,take five, 12.99 Round Midnight, BT015, jazz, Ella Fitzgerald, Midnight, 10.99 New York New York, BT016, New York New York, Frank Sinatra, Trilogy Past Present Future, 9.99 Viva la Vida, BT017, Viva la Vida, Coldplay, Viva la Vida, 12.99 eine kleine nachtmusik, BT018, classical music, mozart, not apllicable, 13.99 Don't leave me here, BT019, blues, keb' mo', don't leave me here, 1.99 Blue 1, BT020, Yellow Submarine, The Beatles, Beatles 1, 1.99 the thrill is gone, BT013, blues, BB king, the thrill is gone, 1.99 Beat it, BT014, Disco, Michael Jackson,Thriller, 1.99
Step by Step Solution
There are 3 Steps involved in it
Step: 1
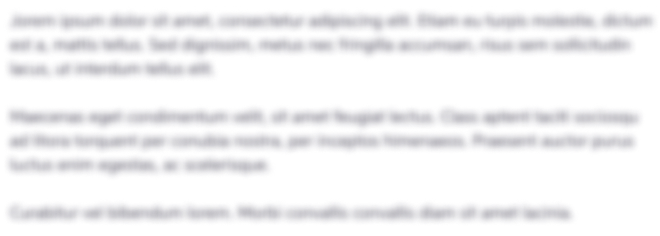
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started