Question
I still need to have more instruction on how to initialize features of a class. Like is it more complex than I think? because I
I still need to have more instruction on how to initialize features of a class. Like is it more complex than I think? because I keep getting syntax errors, they should be pretty simple functions.
__version__ = 1
import math
class RegularPolygon:
'''
This method initializes a regular polygon (a polygon in which all sides)
have equal lengths.The class saves the name of the polygon, the number
of sides, and the length of each side
'''
def __init__(self, name, numSides, sideLength):
self.__name = name
self.__numSides = numSides
self.__sideLength = sideLength
'''
This method computes the perimeter of the polygon by looping over the
number of sides, adding the side length to the sum on each iteration.It
is not relevant to what you need to complete in this program.
'''
def perimeter(self):
perimeter = 0
for i in range(self.__numSides):
perimeter += self.__sideLength
return perimeter
'''
An accessor function for the self.__sideLength variable, which is needed for
the area computations in the child classes
'''
def getSideLength(self):
return self.__sideLength
'''
This class creates a Square shape which inherits from the RegularPolygon class
'''
class Square(RegularPolygon):
'''
TODO:Fill in the details of this constructor so that all of the
parameters are passed to the constructor in the superclass
'''
def __init__(self, name, numSides, sideLength):
pass
'''
TODO:Fill in the details of this method, which should COMPUTE AND RETURN
the area of the Square given the information stored in the
RegularPolygon class (Hint: The area of a square is the side length
squared)
'''
def area(self):
pass
'''
This class creates an EquilateralTriangle shape which inherits from the
RegularPolygon class
'''
class EquilateralTriangle(RegularPolygon):
'''
TODO:Fill in the details of this constructor so that (1) all of the
parameters are passed to the constructor in the superclass, and then
(2) the computeBaseHeight() method is called to populate the
self.__base and self.__height variables
'''
def __init__(self, name, numSides, sideLength):
pass
'''
TODO:Fill in the details of this method, which should COMPUTE AND RETURN
the area of the EquilateralTriangle given the information stored
locally in the EquilateralTriangle class (Hint: The area of an
equilateral triangle is 1/2 * base * height)
'''
def area(self):
pass
'''
This method computes self.__base and self.__height for the equilateral
triangle given the side length saved in the parent class.Nothing is
returned
'''
def computeBaseHeight(self):
self.__base = self.getSideLength()
self.__height = math.sqrt(3)/2 * self.getSideLength()
######################################################################################
def main():
# You can test your solutions by calling them from here
pass
Step by Step Solution
There are 3 Steps involved in it
Step: 1
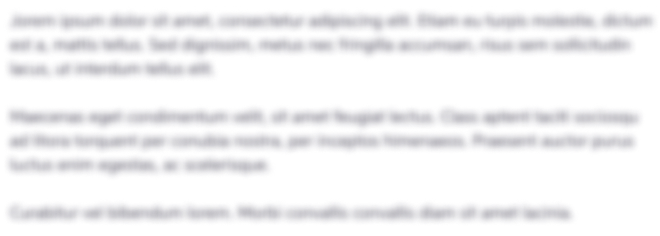
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started