Question
I think I have a good chunk of this figured out, but am running out of time and still have some coding to finish, any
I think I have a good chunk of this figured out, but am running out of time and still have some coding to finish, any help would be appreciated. This is for C++. The instructions for the lab where
Complete the following:
- In clockPart2.cpp: complete the code for all the methods that are not completely written
- In clockLabPart2Driver.cpp
- In void visualTestForSetAheadAnBackMembererFunctions()
write the test code for set hours ahead and back
- In void visualTestForConstructors()
write the test code for the argument constructor and the copy constructor
- Following the pattern given for the 2 visual test functions above
add the following 2 visual test functions to your code
1. write the test code for each visual test
2. call each function from the visualTests() function
void visualTestForSyncAndAssignmentOperator();
void visualTestForDifference();
Right now I am stuck on what to write for this section: void Clock::sync(Clock& right) const
and what to write for this section: int Clock::differenceInClocks(const Clock& right) const
Romoved most of the header file to get the 2 .cpp pages where i have the questions to fit since I'm not worried about having those wrong, Left the information I had prototyped in in case it helps
clockPart2.h
void sync(Clock& right) const; // parameter will have same time as invoking instance
int differenceInClocks(const Clock& right) const;
// will return in minutes the absolute value of the time difference between the
// invoking instance and the parameter
// HINT: instead of comparing 1 hour 55 minutes to 2 hours 12 minutes
// convert both times to minutes past midnight and work with those numbers
// overloaded = operator, study Lecture 3 for an example of the = operator
const Clock& operator=(const Clock& right);
clockPart2.cpp
#include "clockPart2.h"
#include
void Clock::setToCurrentTime()
{
int currentT = time(0);
currentT = currentT % (24 * 60 * 60); // convert to seconds past midnight
currentT = currentT / 60; // convert to minutes
int cHr, cMn;
cHr = currentT / 60; // get current hour
const int GMT_DIFFERENCE = -6; // for Central Standard Time compared to Greenwich Mean Time
cHr = (cHr + GMT_DIFFERENCE + 24) % 24;
cMn = currentT % 60; // get current minute
setHours(cHr);
setMinutes(cMn);
}
Clock::Clock(void)
{
setToCurrentTime();
setChimeOnHalfHour(false);
setChimeOnHour(false);
}
Clock::Clock(int hr, int m, bool chimeHalfHour, bool chimeHour)
{
setMinutes(m);
setHours(hr);
setChimeOnHalfHour(false);
setChimeOnHour(false);
}
Clock::Clock(const Clock& right)
{
setMinutes(right.getMinutes());
setHours(right.getHours());
setChimeOnHalfHour(right.getChimeOnHalfHour());
setChimeOnHour(right.getChimeOnHour());
}
Clock::~Clock()
{
}
void Clock::sync(Clock& right) const // parameter will have same time as invoking instance
{ // sync the time, ie both will have the same values
// but do not change the should chime fields
// students write the code
}
void Clock::setHoursAhead(int h)
{
hours = hours + h;
if (hours > 23)
{
hours = hours % 23;
setHoursAhead(1);
}
}
void Clock::setHoursBack(int h)
{
hours = hours - h;
if (hours < 0)
{
hours = hours + 23;
setHoursBack(1);
}
}
void Clock::setMinutesAhead(int m)
{
setMinutes((getMinutes() + m) % 60);
}
void Clock::setMinutesBack(int m)
{
setMinutes((getMinutes() - m + 60) % 60);
}
int Clock::differenceInClocks(const Clock& right) const
{
return 0; // Dummy Code in order to compile, students write the code
}
const Clock& Clock::operator=(const Clock& right)
{
hours = right.hours;
minutes = right.minutes;
return *this; // necessary for the code to compile
}
int Clock::getMinutes(void) const
{
return minutes;
}
int Clock::getHours(void) const
{
return hours;
}
bool Clock::getChimeOnHalfHour(void) const
{
return shouldChimeOnHalfHour;
}
void Clock::setMinutes(int m)
{
if (m >= 0 && m <= 59)
minutes = m;
else
minutes = 0;
}
void Clock::setHours(int h)
{
if (h >= 0 && h <= 23)
hours = h;
else
hours = 0;
}
void Clock::setChimeOnHalfHour(bool v)
{
shouldChimeOnHalfHour = v;
}
string Clock::chimeOnHalfHour(void) const
{
if (getChimeOnHalfHour() && getMinutes() == 30)
return "ding";
else
return "";
}
bool Clock::getChimeOnHour(void) const
{
return shouldChimeOnHour;
}
void Clock::setChimeOnHour(bool v)
{
shouldChimeOnHour = v;
}
void Clock::tick(void)
{
if (getMinutes() < 59)
setMinutes(getMinutes() + 1);
else
{
setMinutes(0);
if (getHours() < 23)
setHours(getHours() + 1);
setHours(0);
}
}
string Clock::chimeOnHour(void) const
{
if (this->getChimeOnHour() && this->getMinutes() == 0)
{
string answer = "";
int times;
int work = getHours();
if (work == 0) times = 12;
else if (work <= 12) times = work;
else times = work - 12;
for (int i = 1; i <= times; i++)
answer = answer + " dong";
return answer;
}
else
return "";
}
clockLabPart2Driver.cpp
#include "clockPart2.h"
void visualTests();
void visualTestForConstructors();
void visualTestForSetAheadAnBackMembererFunctions();
// Students - following the pattern given for the 2 visual test functions above
// add the following 2 visual test functions to your code
// 1. write the test code for each visual test
// 2. call each function from the visualTests() function
void visualTestForSyncAndAssignmentOperator();
void visualTestForDifference();
void computerTests();
void main()
{
visualTests();
computerTests();
}
void visualTests()
{
visualTestForConstructors();
visualTestForSetAheadAnBackMembererFunctions();
}
void visualTestForConstructors()
{
cout << " visualTestForConstructors" << endl;
cout << " Default Constructor" << endl;
Clock test;
cout << test.getMinutes() << " is the current minute" << endl;
cout << test.getHours() << " is the current hour" << endl;
// same test as above, but with pointers
Clock* testPointer;
testPointer = new Clock;
cout << testPointer->getMinutes() << " is the current minute" << endl;
cout << testPointer->getHours() << " is the current hour" << endl;
cout << " Argument Constructor" << endl;
// STUDENTS - COMPLETE THIS SECTION
cout << " Copy Constructor" << endl;
// STUDENTS - COMPLETE THIS SECTION
}
void visualTestForSetAheadAnBackMembererFunctions()
{
cout << " visualTestForSetAheadAnBackMembererFunctions" << endl;
cout << " Minutes ahead and back" << endl;
Clock test;
test.setMinutes(30);
test.setMinutesAhead(25);
cout << test.getMinutes() << " should be 55" << endl;
test.setMinutesAhead(38);
cout << test.getMinutes() << " should be 33 " << endl;
test.setMinutes(40);
test.setMinutesBack(23);
cout << test.getMinutes() << " should be 17 " << endl;
test.setMinutesBack(20);
cout << test.getMinutes() << " should be 57 " << endl;
test.setMinutesAhead(20);
cout << test.getMinutes() << " should be 17 " << endl;
cout << " Hours ahead and back" << endl;
// STUDENTS - COMPLETE THIS SECTION FOR set hours ahead and back
}
void computerTests()
{
// no compter tests at this time
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
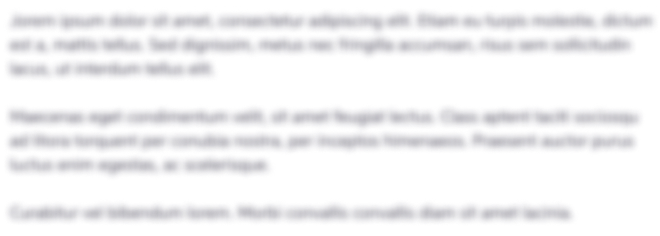
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started