Question
I thought I had a solution to the program, but it has produced errors only. Any help would be appreciated. The list of states and
I thought I had a solution to the program, but it has produced errors only. Any help would be appreciated. The list of states and populations is also attached. I appreciate your time and help. Thank you
"""
Manipulate lists of state populations
Author:
File: ch4_states_v5.py
"""
Manipulate lists of state populations
Author: replace with your name
File: ch4_states_v5.py
Course:
"""
# Now we start to look at small programs. Do not be intimidated... this program looks
# long, it is not. What I have done is include a MOUNTAIN of comments, explaining each
# part as we go. So, read this like a book. Start here, and work your way down. Each
# section explains what it is doing, and indicates where you should add your code to
# complete the program. You will find three items you need to address below.
#
# Most programs in the real world do not have this many comments.
# In a way, that is unfortunate because more often we as programmers are
# improving or fixing bugs in an existing program, rather than starting
# from scratch. Good comments are worth their weight in gold
# because they help you get up to speed quickly, understand what was intended, and describe what
# each section of the program is accomplishing. We will build up our skill in reading other people's
# programs over time, and for this course, you'll get pretty good at reading my programs.
# Okay, here we go
# I am creating a couple of lists that you will work with later in the code. You do not need
# to build these, I will build them for you. The information in these two lists will be supplied from a
# file on your local disk. Here are the names of the two lists:
state_names = [] # a list of all the state names as strings
state_populations = [] # a matching list of those state's populations as integers
# This is one form of debugging a program. I've added some code that will display additional
# information when this variable is set to True. After you complete the steps below, consider
# coming back here and setting this variable to True and see what happens.
print_internal_details = False
# FIRST STEP: Edit the data input file path
# Last week's Digging Deeper worked with a few US states and their populations. This week, we are going to use
# all 50 states. It is too much to type in, so we will start to use the computer to do the work for us.
# The information for all 50 states and their populations is stored in a file.
# This first step tells Python where to find that file.
# The next line of code (starting with INPUT_FILE =) you'll need to edit. Python uses this string
# to find the file.
# Instructions: On your computer, you may need to use a file manager to see the full path of this file,
# then edit the text string inside the single quotes to match your system.
# Leave the leading lowercase r in place (this tells Python not to react to the symbols in the text)
#
# What you see below is on a Windows system... on a Mac this might look like
# r'/Users/john/Documents/CIS156/ch4_us_state_info.csv'
INPUT_FILE = r'C:\Users\john\Documents\CIS156\ch4_us_state_info.csv'
# We're starting to move toward programs with a bit more structure, but don't let that throw you.
# This week you'll be putting your code inside a function called main, which begins right below this comment.
def main():
print('ch4: us_states_v5.py')
print('This program works with more states and their populations')
print("Load data from this file:", INPUT_FILE) # quick check to see if the filename looks ok
get_all_states() # this loads the two lists
if print_internal_details:
print_all_states() # this displays debugging information, useful as a quick check when needed.
print(' Part 1 - check the first five entries for Arizona')
# Check if any of the first five entries in state_names is Arizona. If yes, print a line that
# indicates you found Arizona somewhere in the first five entries. Otherwise, print a line
# that says you didn't find Arizona in the first five entries.
# Hint: several ways to tackle this... you could use a series of if.. elif statements.
# You could use a series of if statements that keep track on any matches you find, perhaps using a boolean variable.
# You could get a slice of the first five in state_names and use a membership test.
# insert your code next - IMPORTANT... indent your code to line up with the beginning of this comment
# this indentation should happen automatically in IDLE...
# just put your cursor at the end of this line and press Enter
# end of part 1
print(' Part 2 - check the first three entries for big population Pacific or Gulf states')
# Check the first three entries in the lists, and test to see if any of them meets BOTH of the
# following conditions:
# is their population equal to or greater than 22 million, and
# do they have a coastline on the Pacific Ocean or on the Gulf of Mexico (Alaska, Hawaii, Washington, Oregon,
# California, Texas, Louisiana, Mississippi, Alabama, Florida)
# If yes, print out the name(s) of those states... you can print multiple lines as desired.
# You only need to check the first three entries... otherwise, you'd be typing a lot
# of statements.
# I am asking only for the state names in Part 2, but you can also print out their populations if you wish
# Major Hints:
# 1. Reminder about the logical operators (zyBooks Table 4.5.3 and Participation 4.5.2)
# to test multiple conditions.
# 2. Consider putting the coastal state names in a list, then doing a membership comparision
# (zyBooks Figure 4.9.1 and Instructor notes).
# insert your code next - IMPORTANT... indent your code to line up with this comment
# end of part 2
# what follows are a couple of functions that help this program.
# Later chapters of our class will cover what these functions are doing
# but for now, you can just live with confidence that each accomplishes the job described
# in the initial comment shown for each function
def get_all_states():
"""Opens a .csv file and loads lists with state names and July 2019 population data
The .csv file is assumed to have a header row, but we skip over it."""
infile = open(INPUT_FILE, 'r')
next(infile) # read and toss away the header which is the first line in the file
for line in infile:
one_line = line.split(',') # obtain one line from the file, parse it using the commas
state_names.append(one_line[0]) # append this name to the tail of the list
x = int(one_line[1]) # convert the population string into integer
state_populations.append(x) # and then append to the tail of the list, repeat the loop till EOF
infile.close()
def print_all_states():
"""Quick check that the data loaded properly"""
print(' Our lists have', len(state_names), 'state names and', len(state_populations), 'populations.')
print("state_names:", state_names)
print()
print("state_populations:", state_populations)
# call main
if __name__ == '__main__':
main()
This was the solution I was working with below. I wrote it into the program, but I keep recieving errors.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
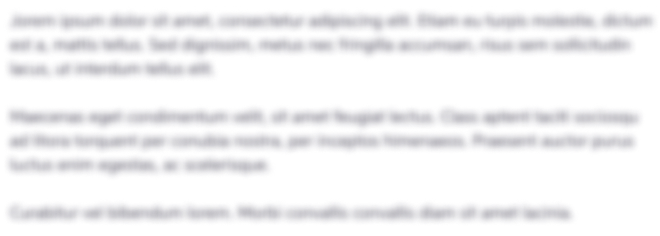
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started