Question
I want the solution in Netbeans In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount
I want the solution in Netbeans
In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Add get methods for the invoice number and sale amount fields so their values can be used in comparisons. Next, write a program that declares an array of five Purchase objects and prompt a user for their values. Then, in a loop that continues until a user inputs a sentinel value, ask the user whether the Purchase objects should be sorted and displayed in invoice number order or sale amount order. Save the file as SortPurchasesArray.java.
Purchase
public class Purchase { int InvoiceNumber; double saleAmount,saleTaxAmount; /*---------------Getter and Setter---------------*/
public int getInvoiceNumber() { return InvoiceNumber; }
public void setInvoiceNumber(int InvoiceNumber) { this.InvoiceNumber = InvoiceNumber; }
public double getSaleAmount() { return saleAmount; }
public void setSaleAmount(double saleAmount) { this.saleAmount = saleAmount; }
public double getSaleTaxAmount() { return saleTaxAmount; }
public void setSaleTaxAmount(double saleTaxAmount) { this.saleTaxAmount = (saleTaxAmount*5)/100; //calculating sale tax amount } public void display() //display() { System.out.println("Invoice Number: "+InvoiceNumber); System.out.println("Sale Amount: "+saleAmount); System.out.println("Sale Tax Amount: "+saleTaxAmount); } /*--------------XXXXXXXX------------------*/ }
Create Purchase
import java.util.Scanner;
public class CreatePurchase //create purchase class { public static void main(String arg[]) { Purchase purchase; //purchase object purchase = new Purchase(); Scanner scanner=new Scanner(System.in); //scanner int invoiceNumber; //to take invoice number from user boolean run=true; //to control while loop
while(run) //while loop { System.out.println("Enter InVoice Number: "); invoiceNumber=scanner.nextInt(); //taking invoice number from user if(invoiceNumber>=1000&&invoiceNumber<=8000) //condition for valid in voice number { purchase.setInvoiceNumber(invoiceNumber); while(run) { double saleAmount; //to take amount from user System.out.println("Enter Sale Amount: "); saleAmount=scanner.nextDouble(); if(saleAmount>=0) //condition for valid amount { run=false; //to stop while loop purchase.setSaleAmount(saleAmount); purchase.setSaleTaxAmount(saleAmount); }else { System.out.println("Invalid Amount.Try Again"); } } }else { System.out.println("Wrong Invoice Number.Try Again"); } } System.out.println("Purchase Detail: "); purchase.display(); //printing purchase details } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
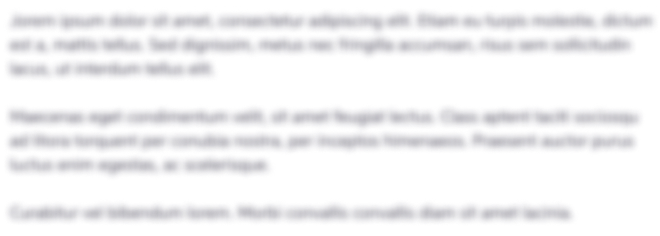
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started