Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I want to figure it out how to display a maze. The below URL is general presentation. Actually I have to do many things but
I want to figure it out how to display a maze.
The below URL is general presentation. Actually I have to do many things but first trying to do maze.1 input.
and tex. file
\documentclass[10pt]{article} \usepackage{tikz} \usetikzlibrary{shapes.misc} \usepackage[margin=0cm]{geometry} \pagestyle{empty} \tikzstyle{every node}=[cross out, draw, red] \begin{document} \vspace*{\fill} \begin{center} \begin{tikzpicture}[x=0.5cm, y=-0.5cm, ultra thick, blue] % Walls \draw (0,0) -- (1,0); \draw (4,0) -- (5,0); \draw (6,0) -- (7,0); \draw (0,1) -- (1,1); \draw (3,1) -- (4,1); \draw (0,2) -- (1,2); \draw (2,2) -- (6,2); \draw (2,3) -- (3,3); \draw (5,3) -- (6,3); \draw (0,4) -- (1,4); \draw (4,4) -- (5,4); \draw (6,4) -- (7,4); \draw (0,5) -- (1,5); \draw (3,5) -- (5,5); \draw (0,1) -- (0,5); \draw (1,1) -- (1,2); \draw (1,4) -- (1,5); \draw (2,0) -- (2,2); \draw (2,3) -- (2,5); \draw (3,0) -- (3,1); \draw (4,1) -- (4,3); \draw (5,0) -- (5,1); \draw (5,4) -- (5,5); \draw (6,0) -- (6,2); \draw (6,3) -- (6,5); \draw (7,1) -- (7,2); \draw (7,4) -- (7,5); % Pillars \fill[green] (1,3) circle(0.2); \fill[green] (7,3) circle(0.2); \fill[green] (3,4) circle(0.2); % Inner points in accessible cul-de-sacs \node at (2.5,0.5) {}; \node at (2.5,1.5) {}; \node at (3.5,1.5) {}; \node at (4.5,4.5) {}; \node at (6.5,4.5) {}; % Entry-exit paths without intersections \draw[dashed, yellow] (3.5,0.5) -- (4.5,0.5); \draw[dashed, yellow] (4.5,1.5) -- (5.5,1.5); \draw[dashed, yellow] (3.5,-0.5) -- (3.5,0.5); \draw[dashed, yellow] (4.5,0.5) -- (4.5,1.5); \draw[dashed, yellow] (5.5,-0.5) -- (5.5,1.5); \end{tikzpicture} \end{center} \vspace*{\fill} \end{document}
class MazeError(Exception): def __init__(self, message): self.message = message class Maze: def __init__(self, filename): pass # REPLACE PASS ABOVE WITH YOUR CODE # POSSIBLY DEFINE OTHER METHODS def analyse(self): pass # REPLACE PASS ABOVE WITH YOUR CODE def display(self): pass # REPLACE PASS ABOVE WITH YOUR CODE
here is what I've done so far
import sys import os class MazeError(Exception): def __init__(self,message): # Call the base class constructor with the parameters super(MazeError, self).__init__(message) class Maze: def __init__(self, filename): self.filename = filename file = open(filename, 'r') def validate_maze(self, maze_array): allowed_digits = (0,1,2,3) maze_list = [] for line in maze_array: if line: if len(line)==1: try: line = list(map(int, str(line[0]))) except: raise MazeError('Incorrect input.') else: try: line = [int(i) for i in line] except: raise MazeError('Incorrect input.') for i in line: if i not in allowed_digits: raise MazeError('Incorrect input.') maze_list.append(line) if len(maze_list)<2 or len(maze_list)>31: raise MazeError('Incorrect input.') a=len(maze_list[0]) for i in maze_list: if len(i)!=a or len(i)>41 or len(i)<2: raise MazeError('Incorrect input.') allowed_column = (2,0) allowed_row = (1,0) for i in maze_list: if i[len(i)-1] not in allowed_column: raise MazeError('Input does not represent a maze.') for i in maze_list[len(maze_list)-1]: if i not in allowed_row: raise MazeError('Input does not represent a maze.') self.maze_list = maze_list self.y_dim_of_maze = len(maze_list[0]) self.x_dim_of_maze = len(maze_list) def draw(maze_array, wall_graph): m = len(maze_array) # m is number of y coordinates n = len(maze_array[0]) # n is number of x coordinates maze_file_path = sys.argv[1] tex_file_path = os.path.splitext(maze_file_path)[0] + ".tex" print(tex_file_path) with open(tex_file_path, "w", encoding='utf-8') as tex: tex.write(r"""\documentclass[10pt]{article} \usepackage{tikz} \usetikzlibrary{shapes.misc} \usepackage[margin=0cm]{geometry} \pagestyle{empty} \tikzstyle{every node}=[cross out, draw, red] \begin{document} \vspace*{\fill} \begin{center} \begin{tikzpicture}[x=0.5cm, y=-0.5cm, ultra thick, blue] % Walls """) for y in range(m): for x in range(n): for node_connection in wall_graph[x][y]: tex.write(f" \draw ({x},{y}) -- ({node_connection[0]},{node_connection[1]}); ") tex.write(r"""% Pillars """) for y in range(m): for x in range(n): if len(wall_graph[x][y]) == 0: tex.write(f" \\fill[green] ({x},{y}) circle(0.2); ") tex.write(r"""\end{tikzpicture} \end{center} \vspace*{\fill} \end{document} """) def get_maze_array(self): maze_array = [] # 2d list with open(sys.argv[1], 'r') as maze_txt: for line in maze_txt.readlines(): sanitized_line: str = "".join(line.split()) # should look like"10221230" if not sanitized_line: # if line is empty continue numbers: list[str] = list(sanitized_line) # ["1", 0, 2, 2, 1, 2, 3, 0] maze_array.append(numbers) return maze_array # maze_array has been populated as a 2d list of numbers ranging from 0 to 3 def transform_maze_array_into_wall_graph(self,maze_array): m = len(maze_array) # m is number of y coordinates n = len(maze_array[0]) # n is number of x coordinates # initialize wall graph wall_graph[x][y] = [(Tuple)] wall_graph = [] for x in range(n): new = [] for y in range(m): new.append([]) wall_graph.append(new) # populate wall graph for y in range(m): for x in range(n): value = maze_array[y][x] if value == "0": continue if value == "1": wall_graph[x][y].append((x+1, y)) wall_graph[x+1][y].append((x, y)) if value == "2": wall_graph[x][y].append((x, y+1)) wall_graph[x][y+1].append((x, y)) if value == "3": wall_graph[x][y].append((x+1, y)) wall_graph[x][y+1].append((x, y)) wall_graph[x][y].append((x, y+1)) wall_graph[x+1][y].append((x, y)) return wall_graph def main(): maze_array = get_maze_array() validate_maze(maze_array) wall_graph = transform_maze_array_into_wall_graph(maze_array) # maze_graph = transform_wall_graph_into_maze_graph(wall_graph) # search(maze_graph) # find cul de sac, find distinct accessible regions, find inaccessible regions, find the best path draw(maze_array, wall_graph) if __name__ == "__main__": main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
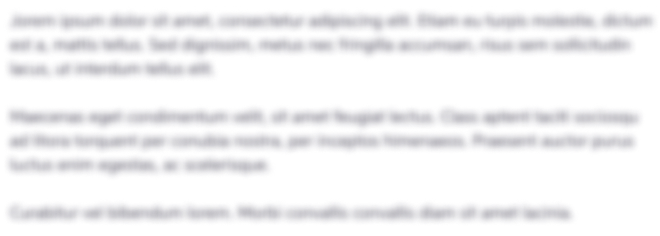
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started