Question
I want to solve a task of using the queue , using this classes and complete the last code Task : A normal queue is
I want to solve a task of using the queue , using this classes and complete the last code
Task :
A normal queue is a FIFO data structure; elements are dequeued from the front of the queue and enqueued at the end of the queue. A priority queueis a queue in which elements are also dequeued from the front of the queue; but they are enqueued according to their associated priorities. A higher priority item is always enqueued before a lower priority element. An element that has the same priority as one or more elements in the queue is enqueued after all the elements with that priority.
(a) Complete the implementation of the PriorityQueueAsLinkedListclass in the CSE202.lab4package by overriding the enqueuemethod.
(b) What is the big-O complexity of the enqueuemethod ?
(c) Patients in a hospital are assigned priorities to see a doctor. Write a test program, TestPriorityQueuein the CSE202.lab4package so that:
1. For each patient: It prompts for and reads the patient ID and the priority of the patient (an integer between 1 and 5) to see a doctor.
2. Initializes a priority queue with the data that is read. Each patient object is an Associationobject that contains the priority as the key, and the ID as the associated value.
3. Displays the contents of the priority queue using the PrintingVisitorpackage.
Sample input/ output:
postfix expression | value |
5 9 + 2 * 6 5 * + | 58 |
6 2 + 5 8 4 / - * | 24 |
6 2 + 5 * 8 4 / - | 38 |
...........................................
public interface Queue extends Container {
public abstract Object getHead();
public abstract void enqueue(Object obj);
public abstract Object dequeue();
}
.....................................
import java.util.NoSuchElementException;
public class QueueAsLinkedList extends AbstractContainer implements Queue { protected MyLinkedList list; public QueueAsLinkedList() { list = new MyLinkedList(); }
public void purge() { list.purge(); count = 0; }
public Object getHead() { if(count == 0) throw new ContainerEmptyException(); else return list.getFirst(); }
public void enqueue(Object obj) { list.append(obj); count++; }
public Object dequeue() { if(count == 0) throw new ContainerEmptyException(); else { Object obj = list.getFirst(); list.extractFirst(); count--; return obj; } }
public Iterator iterator() { return new Iterator() { MyLinkedList.Element position = list.getHead();
public boolean hasNext() { return position != null; }
public Object next() { if(position == null) throw new NoSuchElementException(); else { Object obj = position.getData(); position = position.getNext(); return obj; } } }; } }
...........................................
import java.util.NoSuchElementException; import CSE202.*;
public class PriorityQueueAsLinkedList extends QueueAsLinkedList { public void enqueue(Object obj) { // to be completed by students } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
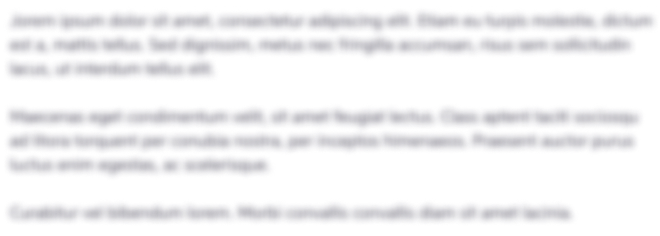
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started