Question
I will upvote as soon as I can! **Note: A starting GUI was already provided. YOU DO NOT need to do create any methods pertaining
I will upvote as soon as I can!
**Note: A starting GUI was already provided. YOU DO NOT need to do create any methods pertaining to ClassJar this is only for the GUI. If you need more info please comment, although I will admit the instructions are sort of vague but more context is provided within the provided GUI.
Instructions: Create 3 ChangeJars with associated JButtons. Call using JButtons, or any other JComponent so all of the methods you created in your ChangeJar class (load, save, inc, etc.) are called from your GUI. Create a JButton that calls the mutate(Boolean on) method (only one JButton is required for all ChangeJars). Finally, show the values for quarters, dimes, nickels and pennies on the display for each ChangeJar.
**Context:
public class ChangeJarPanel extends JPanel{ private ChangeJar jar; NumberFormat fmt = NumberFormat.getCurrencyInstance(); JButton takeOutButton; JTextField qField, dField, nField, pField; /** labels for message and credits */ JLabel message, credits; public ChangeJarPanel(){ // create the game object as well as the ChangeJarGUI Frame jar = new ChangeJar(100,2,3,4); // set the layout to GridBag setLayout(new GridLayout(6,2)); setBackground(Color.lightGray); qField = new JTextField("0", 3); add(qField); add(new JLabel("Quarters:")); dField = new JTextField("0", 3); add(dField); add(new JLabel("Dimes:")); nField = new JTextField("0", 3); add(nField); add(new JLabel("Nickels:")); pField = new JTextField("0", 3); add(pField); add(new JLabel("Pennies:")); takeOutButton = new JButton("Take Out"); add(takeOutButton); credits = new JLabel(); credits.setText(fmt.format(jar.getAmount())); add (new JLabel(" ")); add(new JLabel("Total:")); add(credits); // register the listeners takeOutButton.addActionListener(new ButtonListener()); } /**************************************************************** Class used to repond to the user action ****************************************************************/ private class ButtonListener implements ActionListener{ public void actionPerformed(ActionEvent event){ int quarters, dimes, nickels, pennies; if (event.getSource() == takeOutButton){ try{ quarters = Integer.parseInt(qField.getText()); dimes = Integer.parseInt(dField.getText()); nickels = Integer.parseInt(nField.getText()); pennies = Integer.parseInt(pField.getText()); jar.takeOut(quarters,dimes,nickels,pennies); }catch(NumberFormatException io){ JOptionPane.showMessageDialog(null,"Enter an integer in all fields"); }catch(IllegalArgumentException e){ JOptionPane.showMessageDialog(null,"Not enough specified coins for this operation"); } } // update the labels credits.setText(fmt.format(jar.getAmount())); } }
======================
public class ChangeJarPanelMain extends JPanel { private JMenuItem quitItem; private JMenuItem suspendItem; public ChangeJarPanelMain (JMenuItem quitItem, JMenuItem suspendItem) { JPanel panel = new JPanel(); panel.add(new ChangeJarPanel()); panel.add(new ChangeJarPanel()); panel.add(new ChangeJarPanel()); add(panel); this.quitItem = quitItem; this.suspendItem = suspendItem; quitItem.addActionListener(new Mylistener()); suspendItem.addActionListener(new Mylistener()); } private class Mylistener implements ActionListener { public void actionPerformed(ActionEvent e){ if(e.getSource() == quitItem){ System.exit(1); } if(e.getSource() == suspendItem){ ChangeJar.mutate(suspendItem.isSelected()); } } } }Implement the following methods and properties in Change Jar class. For properties, you will need four instance variables: quarters (integer), dimes (integer), nickels (integer), pennies (integer). There are no dollars bills (or dollar coins) in this Change Jar. For methods, you will need to implement the following (include any setters or getters that are needed). Unless otherwise stated, you can assume the input has no errors for step 2 (i.e., a valid set of numbers) contained within (later steps this does not apply). public ChangeJar() Default constructor that sets the Change Jar to zero. public ChangeJar (double amount) A constructor that initializes the instance variables with the provided value converted to quarters, dimes, nickels, and pennies. For example, if amount was 1.34 then you would have 5 quarters, 1 nickel, 4 pennies. Page public Changejar (ChangeJar other) A constructor that initializes the instance variables with the other ChangeJar parameter. public ChangeJar (String amount) A constructor that accepts a string as a parameter with the provided value converted to quarters, dimes, nickels, and pennies. For example, if amount was 1.34" then you would have 5 quarters, 1 nickel, 4 pennies. More examples: o 1.3 is 5 quarters, 1 nickel o 1.34 is 5 quarters, 1 nickel, 4 pennies O "0.34 is 34 cents; 1 quarter, 1 nickel, 4 pennies O "0.04 is 4 cents o "500. is not allowed, instead "500.0 is used. public boolean equals (Object other) A method that returns true if this Change Jar object is exactly the same (in terms of the amount in the Change Jar) as the other object (Note: you must cast the other object as a Change Jar object). public static boolean equals (ChangeJar jari, ChangeJar jar2) A static method that returns true if Change Jar object jarl is exactly the same (in terms of amount in the Change Jar) as if Change Jar object jar2. public int compareTo (ChangeJar other) A method that returns 1 if this Change Jar object is greater than (based upon the total in the ChargeJar) the other Change Jar object; returns -1 if the "this Change Jar object is less than the other Change Jar; returns 0 if the this Change Jar object is equal to the other ChangeJar object. public static int compareTo (ChangeJar jari, Change Jar jar2) A method that returns 1 if Change Jar object jarl is greater (in terms of the amount in the Change Jar) than ChangeJar object jar2; returns -1 if the Change Jar object jarl is less than Change Jar jar2; returns 0 if the Change Jar object jarl is equal to ChangeJar object jar2. public void subtract(int quarters, int dimes, int nickels, int pennies) A method that subtracts the parameters from the this ChangeJar object. You may assume all of the parameter are positive. public void subtract (Changejar other) A method that subtracts Change Jar other to the this Change Jar object. (For step 2 there are no worries about errors) public void dec (). A method that decrements the this ChangeJar by 1 penny. public void add (int quarters, int dimes, int nickels, int pennies) A method that adds the parameters from the this ChangeJar object. You may assume all of the parameter are positive. public void add (Changejar other) A method that add ChangeJar other to the this Change Jar object. public void inc() A method that increments the this ChangeJar by 1 penny. public String toString() Method that returns a string that represents a ChangeJar with the following format: 10 quarters, 1 dime, 0 nickels, 1 penny. Be sure to use proper pluralization. For example, 1 penny or 2 pennies. public void save (String fileName) A method that saves the 'this" Changejar to a file; use the parameter filename for the name of the file. public void load (String fileName) A method that loads the this Change Jar object from a file; use the parameter filename for the name of the file. public Change Jar takeOut (double amount) Take out amount using the available quarters, dimes, nickels and pennies. For example: 1.23 would be 4 quarters, 2 dimes, 3 pennies. Example 2. The Changejar holds 4 quarters, 3 dimes, 0 nickels, 3 pennies; now takeout(.80) which would be 2 quarters and 3 dimes. O This sounds easy, it is not. public static void Mutate (Boolean on) A method that turns off (false) and on' (true) any subtract/add methods in Change Jar based on the value (true,false) of the variable on. In other words, when on is false, this prevents any subtract/add method from changing (mutate) the state of the "this object as it relates to the amount in the ChangeJar. Implement the following methods and properties in Change Jar class. For properties, you will need four instance variables: quarters (integer), dimes (integer), nickels (integer), pennies (integer). There are no dollars bills (or dollar coins) in this Change Jar. For methods, you will need to implement the following (include any setters or getters that are needed). Unless otherwise stated, you can assume the input has no errors for step 2 (i.e., a valid set of numbers) contained within (later steps this does not apply). public ChangeJar() Default constructor that sets the Change Jar to zero. public ChangeJar (double amount) A constructor that initializes the instance variables with the provided value converted to quarters, dimes, nickels, and pennies. For example, if amount was 1.34 then you would have 5 quarters, 1 nickel, 4 pennies. Page public Changejar (ChangeJar other) A constructor that initializes the instance variables with the other ChangeJar parameter. public ChangeJar (String amount) A constructor that accepts a string as a parameter with the provided value converted to quarters, dimes, nickels, and pennies. For example, if amount was 1.34" then you would have 5 quarters, 1 nickel, 4 pennies. More examples: o 1.3 is 5 quarters, 1 nickel o 1.34 is 5 quarters, 1 nickel, 4 pennies O "0.34 is 34 cents; 1 quarter, 1 nickel, 4 pennies O "0.04 is 4 cents o "500. is not allowed, instead "500.0 is used. public boolean equals (Object other) A method that returns true if this Change Jar object is exactly the same (in terms of the amount in the Change Jar) as the other object (Note: you must cast the other object as a Change Jar object). public static boolean equals (ChangeJar jari, ChangeJar jar2) A static method that returns true if Change Jar object jarl is exactly the same (in terms of amount in the Change Jar) as if Change Jar object jar2. public int compareTo (ChangeJar other) A method that returns 1 if this Change Jar object is greater than (based upon the total in the ChargeJar) the other Change Jar object; returns -1 if the "this Change Jar object is less than the other Change Jar; returns 0 if the this Change Jar object is equal to the other ChangeJar object. public static int compareTo (ChangeJar jari, Change Jar jar2) A method that returns 1 if Change Jar object jarl is greater (in terms of the amount in the Change Jar) than ChangeJar object jar2; returns -1 if the Change Jar object jarl is less than Change Jar jar2; returns 0 if the Change Jar object jarl is equal to ChangeJar object jar2. public void subtract(int quarters, int dimes, int nickels, int pennies) A method that subtracts the parameters from the this ChangeJar object. You may assume all of the parameter are positive. public void subtract (Changejar other) A method that subtracts Change Jar other to the this Change Jar object. (For step 2 there are no worries about errors) public void dec (). A method that decrements the this ChangeJar by 1 penny. public void add (int quarters, int dimes, int nickels, int pennies) A method that adds the parameters from the this ChangeJar object. You may assume all of the parameter are positive. public void add (Changejar other) A method that add ChangeJar other to the this Change Jar object. public void inc() A method that increments the this ChangeJar by 1 penny. public String toString() Method that returns a string that represents a ChangeJar with the following format: 10 quarters, 1 dime, 0 nickels, 1 penny. Be sure to use proper pluralization. For example, 1 penny or 2 pennies. public void save (String fileName) A method that saves the 'this" Changejar to a file; use the parameter filename for the name of the file. public void load (String fileName) A method that loads the this Change Jar object from a file; use the parameter filename for the name of the file. public Change Jar takeOut (double amount) Take out amount using the available quarters, dimes, nickels and pennies. For example: 1.23 would be 4 quarters, 2 dimes, 3 pennies. Example 2. The Changejar holds 4 quarters, 3 dimes, 0 nickels, 3 pennies; now takeout(.80) which would be 2 quarters and 3 dimes. O This sounds easy, it is not. public static void Mutate (Boolean on) A method that turns off (false) and on' (true) any subtract/add methods in Change Jar based on the value (true,false) of the variable on. In other words, when on is false, this prevents any subtract/add method from changing (mutate) the state of the "this object as it relates to the amount in the ChangeJar
Step by Step Solution
There are 3 Steps involved in it
Step: 1
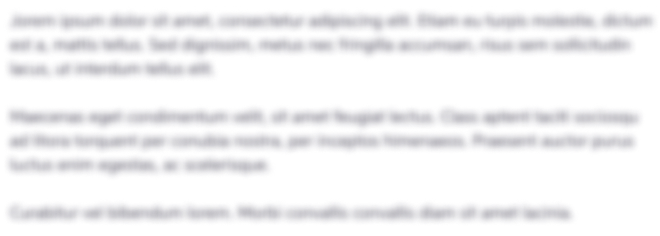
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started