Question
I would appreciate any help on these below functions. Thank you so much for looking at this. this code places items into inventories. Can you
I would appreciate any help on these below functions. Thank you so much for looking at this. this code places items into inventories. Can you provide these functions or explanations on how I may write them? I will be sure to thumbs up for any effort you can provide me.
The Inventory class is partially implemented. However, I still need to complete the Inventory ADT.
What I need help with is listed below. You will see some blank areas where I simply wrote comments on what the code should do.
- Copy Constructor
- Destructor
- Inventory::isFull - check if the inventory is full. return (occupied < slots) if inventory is full return false.
- Inventory::findMatchingItemStackNode - refer to my comments in the code
- Inventory::mergeStacks - (merge 2 item stacks. lhs item stack where items need to be added. rhs item stack with the number of items to add. lhs.id == rhs.id) return nullptr
- Inventory::addItemStackNoCheck - update lhs, append a node. when this method is invoked all special cases have already been covered in 'addItems'
The partial implementation provided assumes that each Inventory will keep track of items in a linked list. (Which I would like to keep the way it is)
The first file, itemsList-0x.txt, lists all possible items. Each line represents one item in the form id name.
Example 1: Sample itemsList-0x.txt
0 Air 1 HP Potion 2 MP Potion 5 Iron Ore 3 Bow Tie 4 Dirt 6 Diamond Ore 7 Iron Ingot 8 Diamond 9 Diamond Block
The second file, inventoryList-0x.txt, lists each individual inventoryor storage chestfollowed by a list of items.
Example 2: Sample inventoryList-0x.txt
# 5 - 1 10 - 2 5 - 3 2 # 6 - 4 3 - 5 27 - 6 44 - 7 55 - 8 1 - 9 4 - 4 3 # 2 - 2 5 - 9 4 - 8 1 - 5 2 - 10 5
Each line preceded by # denotes the start of a new inventory. Each line preceded by - denotes an item. The program creates a new inventory each time a # is encountered.
When a - is encountered, a stack of items, ItemStack, is created. The ItemStack is placed in the Inventory based on the following rules:
- If the Inventory is empty, store the ItemStack, and return true.
- If the Inventory is not empty, examine the Inventory.
- If a matching ItemStack is found, merge the two ItemStacks and return true.
- If no matching ItemStack is found, store the new ItemStack and return true.
- If the Inventory is full, return false.
The output consists of three reports written to standard output, one after the other.
-
A report listing items that were stored or discarded.
-
A report listing all valid items.
-
Finally, a detailed report is printed. listing data for each inventory:
- Maximum Capacityi.e., total slots.
- Utilized Capacityi.e., occupied slots
- Listing of all items.
If the program is run with the provided input files, the following output should be generated
Example 3: Sample Output
Processing Log: Stored (10) HP Potion Stored ( 5) MP Potion Stored ( 2) Bow Tie Stored ( 3) Dirt Stored (27) Iron Ore Stored (44) Diamond Ore Stored (55) Iron Ingot Stored ( 1) Diamond Stored ( 4) Diamond Block Stored ( 3) Dirt Stored ( 5) MP Potion Stored ( 4) Diamond Block Discarded ( 1) Diamond Discarded ( 2) Iron Ore Item List: 0 Air 1 HP Potion 2 MP Potion 3 Bow Tie 4 Dirt 5 Iron Ore 6 Diamond Ore 7 Iron Ingot 8 Diamond 9 Diamond Block Storage Summary: -Used 3 of 5 slots (10) HP Potion ( 5) MP Potion ( 2) Bow Tie -Used 6 of 6 slots ( 6) Dirt (27) Iron Ore (44) Diamond Ore (55) Iron Ingot ( 1) Diamond ( 4) Diamond Block -Used 2 of 2 slots ( 5) MP Potion ( 4) Diamond Block
Step by Step Solution
There are 3 Steps involved in it
Step: 1
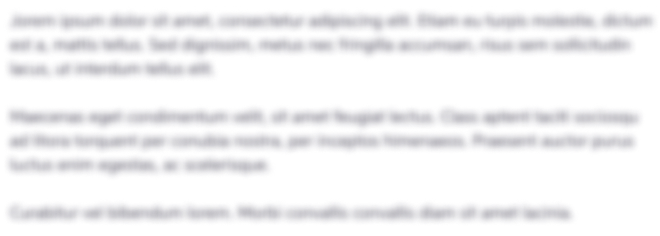
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started