Question
I would like some assistance with fixing this code in order to not get compile errors. Also I am looking for some help with creating
I would like some assistance with fixing this code in order to not get compile errors. Also I am looking for some help with creating a recipeBox application for this code.
Any assistance that can be provided would be great.
Thanks,
Ingredient class
import java.util.Scanner;
public class Ingredient { /** * create instance variables */ private String nameOfIngredient; private double ingredientAmount; private String unitMeasurement; private int numberCaloriesPerUnit;
Ingredient() { }
public String getnameOfIngredient(){ return nameOfIngredient; } public void setnameOfIngredient(String ingredientName) {
this.nameOfIngredient = ingredientName; } public double getingredientAmount(){ return ingredientAmount; } public void setIngredientAmount(double ingredientAmount) {
this.ingredientAmount = ingredientAmount; } public double getnumberCaloriesPerUnit(){ return numberCaloriesPerUnit; } public String getunitMeasurement() { return unitMeasurement; } public void setunitMeasurement(String unitMeasurement) {
this.unitMeasurement = unitMeasurement;
} public Ingredient (String nameOfIngredient, double ingredientAmount, String unitMeasurement, int numberCaloriesPerUnit){ this.nameOfIngredient = nameOfIngredient; this.ingredientAmount = ingredientAmount; this.unitMeasurement = unitMeasurement; this.numberCaloriesPerUnit= numberCaloriesPerUnit; } public Ingredient addIngredient() { String regExp = "^[a-zA-Z]+$"; // reg expression used to validate string. Scanner scnr = new Scanner(System.in); //loop to keep accepting Ingredient name until the validation is done while(true) { System.out.println("Please enter the name of the ingredient: "); nameOfIngredient = scnr.next(); //prompting user to input the name of the ingredient //check whether the ingridient name matches with regular expression if(nameOfIngredient.matches(regExp)) break; //exit the loop else { //display an error message System.out.println("Name should contain alphabets only. Please try again"); } } while(true) { //try block to handle the part fo the code that may generate exception try { System.out.println("Please enter the amount of "+ nameOfIngredient + " we'll need: "); ingredientAmount = scnr.nextFloat(); //prompting user to input the ingredient amount break; //if the input is valid then exit the loop } //handle the exception and show error message catch (Exception e) { System.out.println("Please enter numeric value only."); scnr.next(); //get the input again } } //prompting user to input the ingredient amount //loop to keep accpeting unitMeasurement until the validation is done while(true) { System.out.println("Please enter the unit of measurement "); unitMeasurement = scnr.next(); //asking user to input the measurement amount //check whether the unitMeasurement matches with regular expression if(unitMeasurement.matches(regExp)) break; //exit the loop else { //display an error message System.out.println("ERROR! unitMeasurement should contain alphabets only (Eg. gram, KG, ML etc."); } } /**loop to keep accepting calories until the validation is done * */ while(true) { //try block to handle the part fo the code that may generate exception try { System.out.println("Please enter the number of calories per unit: "); numberCaloriesPerUnit = scnr.nextInt(); // prompting user to enter the number of calories per cup break; } //handle the exception and show error message catch (Exception e) { System.out.println("Please enter integer value only."); scnr.next(); } } // prompting user to enter the number of calories per cup float totalCalories = (float) (ingredientAmount * numberCaloriesPerUnit); // expression to calculate the total calories assigned to totalCalories System.out.println(nameOfIngredient + " uses " + ingredientAmount + " and has " + totalCalories + " calories."); return null; }
void setNumberOfCaloriesPerIngredient(int ingredientCalories) { } }
//Recipe class
import java.util.ArrayList; import java.util.List; import java.util.Scanner;
public class Recipe { private String recipeName; private int servings; private List recipeIngredients; private double totalRecipeCalories; public List listofRecipes = new ArrayList(); public String getRecipeName() { return recipeName; } public void setRecipeName(String recipeName) { this.recipeName = recipeName; } public int getServings() { return servings; } public void setServings(int servings) { this.servings = servings; } public List getRecipeIngredients() { return recipeIngredients; } public void setRecipeIngredients(List recipeIngredients) { this.recipeIngredients = recipeIngredients; } public double getTotalRecipeCalories() { return totalRecipeCalories; } public void setTotalRecipeCalories(double totalRecipeCalories) { this.totalRecipeCalories = totalRecipeCalories; }
//default constructor public Recipe() { this.recipeName = ""; this.servings = 0; this.recipeIngredients = new ArrayList; this.totalRecipeCalories = 0; } //overloaded constructor to create custom recipe public Recipe(String recipeName, int servings, ArrayList recipeIngredients, double totalRecipeCalories) { this.recipeName = recipeName; this.servings = servings; this.recipeIngredients = recipeIngredients; this.totalRecipeCalories = totalRecipeCalories; } public void printRecipe() { int singleServingCalories = 0; singleServingCalories = (int) (this.totalRecipeCalories/this.servings); System.out.println("Recipe: " + this.recipeName); System.out.println("Serves: " + this.servings); System.out.println("Ingredients: "); for(Ingredient ingredients : recipeIngredients) { System.out.println("Ingredient name: " + ingredients.getnameOfIngredient() ); System.out.println("Ingredient Amount: " + ingredients.getingredientAmount() ); System.out.println("Each serving has " + singleServingCalories + " Calories with measurement: " + ingredients.unitMeasurement); } } /* * add new recipe per user inputs */ public Recipe addNewRecipe() { double totalRecipeCalories = 0.0; boolean addMoreIngredients = true; Scanner scnr = new Scanner(System.in); System.out.println("Please enter the recipe name: "); String recipeName = scnr.nextLine(); System.out.println("Please enter the number of servings: "); int servings = scnr.nextInt(); do { Ingredient thisIngredient = new Ingredient(); System.out.println("Please enter the ingredient name " + "or type end if you are finished entering ingredients: "); String ingredientName = scnr.next(); if (ingredientName.toLowerCase().equals("end")) { addMoreIngredients = false; } else { thisIngredient.setnameOfIngredient(ingredientName); //adding ingredient to recipe list System.out.println("Please enter the ingredient amount: "); float ingredientAmount = scnr.nextFloat(); thisIngredient.setIngredientAmount(ingredientAmount); System.out.println("Please enter the ingredient Calories: "); int ingredientCalories = scnr.nextInt(); thisIngredient.setNumberOfCaloriesPerIngredient(ingredientCalories); //Add the total Calories from this ingredient totalRecipeCalories = totalRecipeCalories + (ingredientCalories * ingredientAmount); recipeIngredients.add(thisIngredient); } } while (addMoreIngredients); //looping to taking multiple ingredients from user until typed as "end" //create new recipe with user inputs Recipe recipe; recipe = new Recipe(recipeName,servings, recipeIngredients, totalRecipeCalories); recipe.printRecipe(); return recipe; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
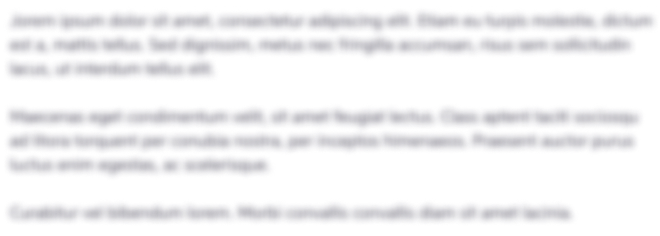
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started